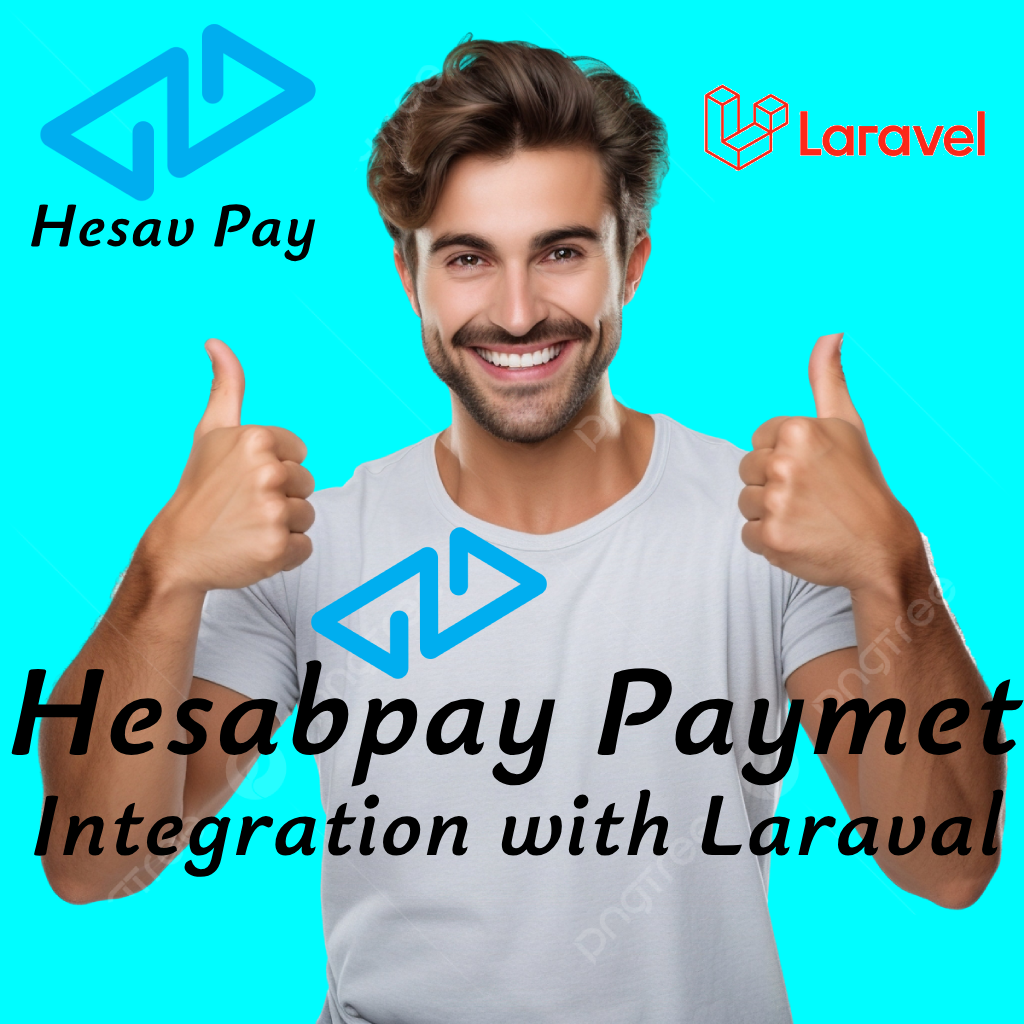
Before you start Hesabpay Payment Integration in your Website or in Mobile app for Receiving your business Payments follow the following terms.
- You Need Business HesabPay Account from https://developers-sandbox.hesab.com/
- Login into your Verified Hesabpay Sandbox
- Your Dashboard will look like this.
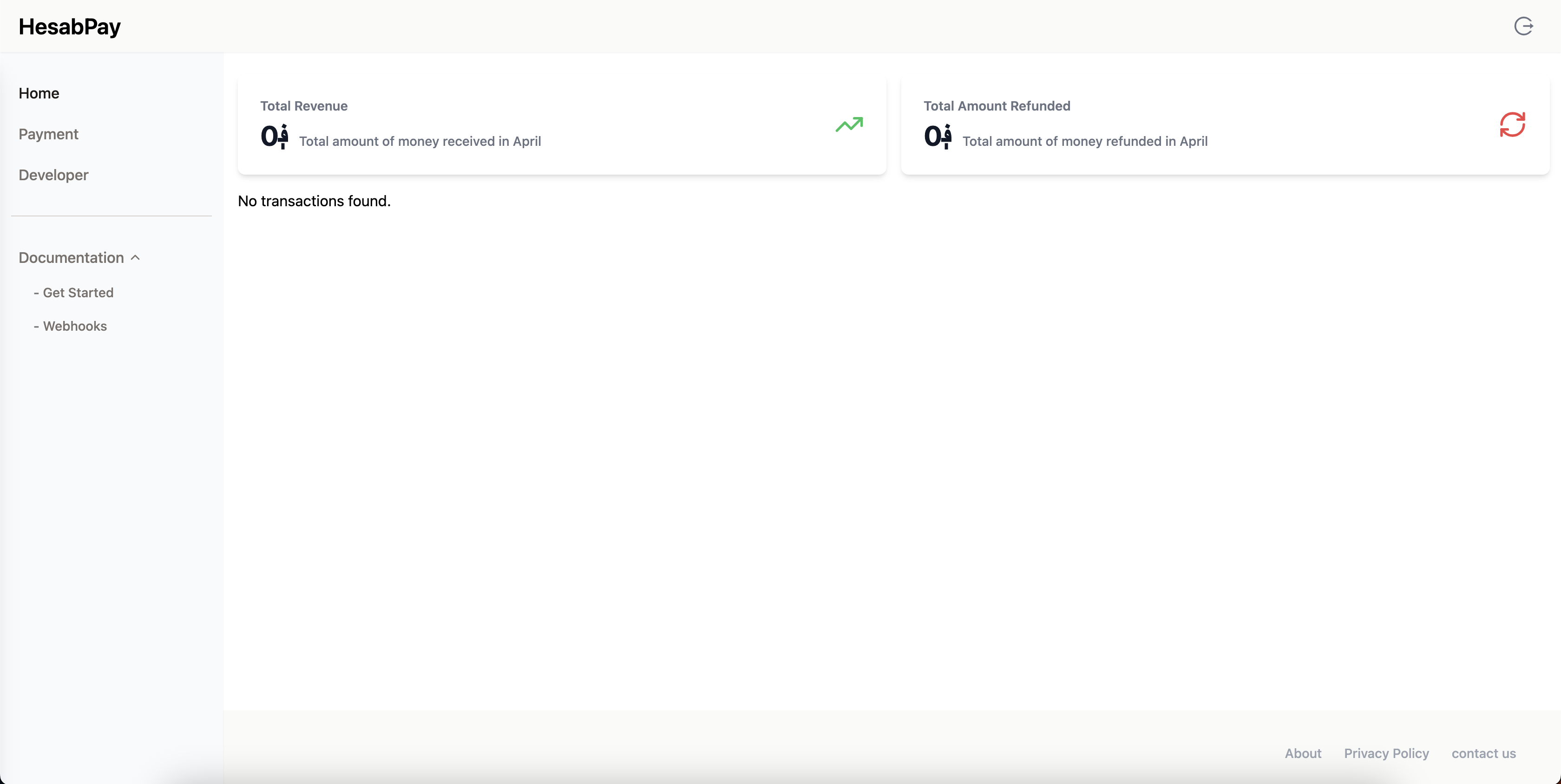
5. Go to Developer to create API
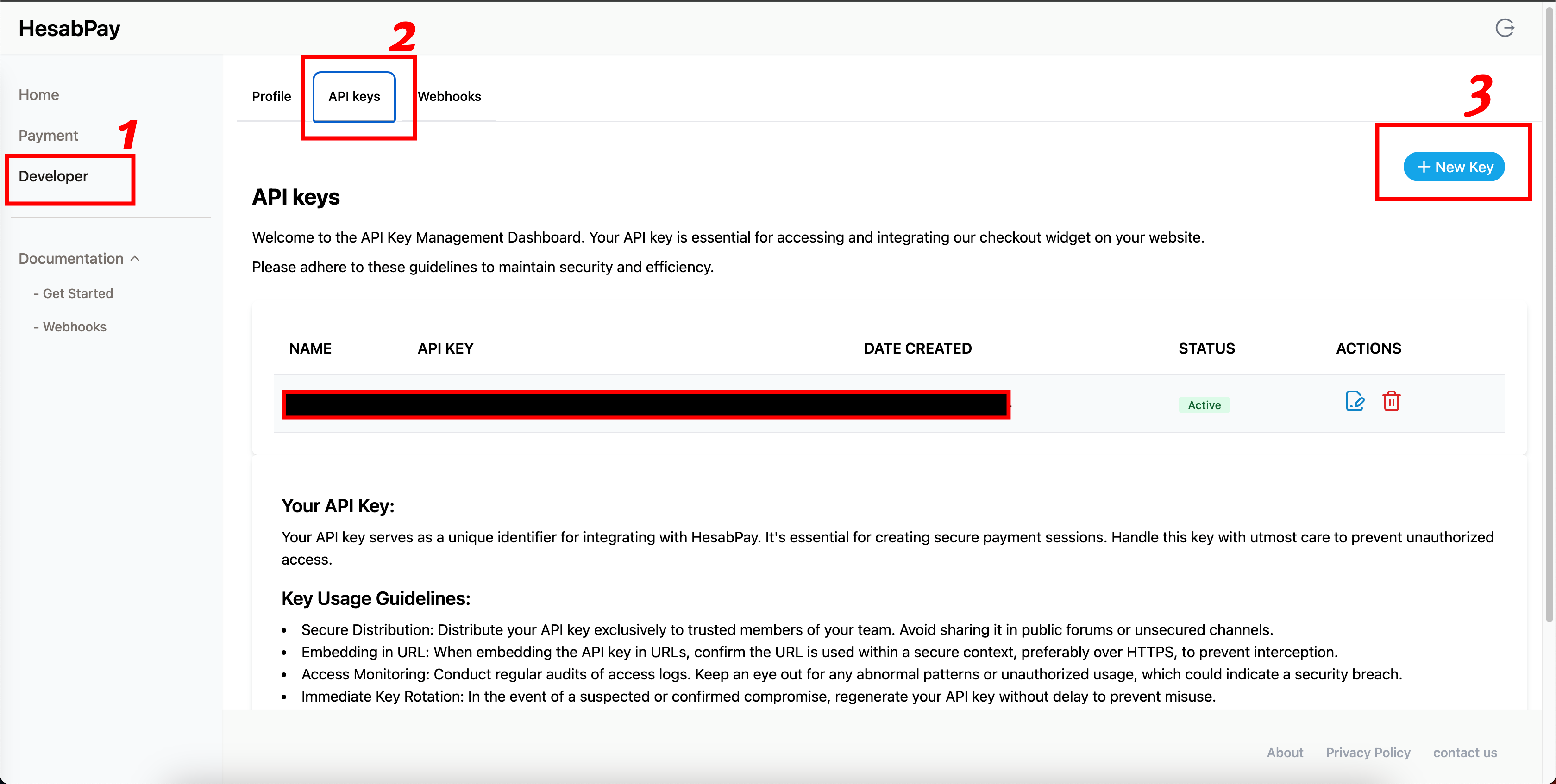
6. Once you Created an API then go to Documentation>>Get Started
7. Select Backend Language suc as Python, PHP, NodeJs
Python :
import requests
def create_payment_session(api_key, items):
endpoint = "https://api-sandbox.hesab.com/api/v1/payment/create-session"
headers = {
"Authorization": f"API-KEY {api_key}"
}
payload = {
"items": items
}
try:
response = requests.post(endpoint, json=payload, headers=headers)
if response.status_code == 200:
return response.json()
else:
return {"error": f"HTTP Error: {response.status_code}", "message": response.text}
except requests.exceptions.RequestException as e:
return {"error": "Request Exception", "message": str(e)}
PHP:
<?php
function create_payment_session($api_key, $items) {
$endpoint = "https://api-sandbox.hesab.com/api/v1/payment/create-session";
$headers = ["Authorization: API-KEY " . $api_key];
$payload = ["items" => $items];
$ch = curl_init($endpoint);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($payload));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
if(curl_getinfo($ch, CURLINFO_HTTP_CODE) == 200) {
return json_decode($response, true);
} else {
return ["error" => "HTTP Error: " . curl_getinfo($ch, CURLINFO_HTTP_CODE), "message" => $response];
}
curl_close($ch);
}
?>
NodeJs:
const axios = require('axios');
async function create_payment_session(api_key, items) {
const endpoint = 'https://api-sandbox.hesab.com/api/v1/payment/create-session';
const headers = {
'Authorization': `API-KEY ${api_key}`
};
const payload = {
items
};
try {
const response = await axios.post(endpoint, payload, { headers });
if (response.status === 200) {
return response.data;
} else {
return { error: `HTTP Error: ${response.status}`, message: response.statusText };
}
} catch (error) {
return { error: 'Request Exception', message: error.message };
}
}
Lets try for PHP/Laravel
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Http;
class CheckoutController extends Controller
{
public function index()
{
// Retrieve the list of items available for selection
$items = [
['id' => 1, 'name' => 'Item 1', 'price' => 10],
['id' => 2, 'name' => 'Item 2', 'price' => 20]
];
// Pass the list of items to the view
return view('checkout.index', compact('items'));
}
public function checkout(Request $request)
{
// Validate the request data
$request->validate([
'numItems' => 'required|integer|min:1',
]);
$api_key = 'Your API KEY HERE';
$numItems = $request->input('numItems');
$items = [];
// Collect selected items
for ($i = 1; $i <= $numItems; $i++) {
$items[] = ['name' => 'mobile a32', 'price' => '32'];
}
// Call the function to create payment session
$response = $this->create_payment_session($api_key, $items);
// Check if the response contains a new URL
if (isset($response['url'])) {
// Append total amount as a query parameter to the URL
$totalAmount = array_reduce($items, function ($carry, $item) {
return $carry + $item['price'];
}, 0);
$redirectUrl = $response['url'] . '?totalAmount=' . $totalAmount;
// Redirect the user to the new URL
return redirect()->away($redirectUrl);
}
// Display the response
dd($response);
}
public function create_payment_session($api_key, $items)
{
$endpoint = "https://api-sandbox.hesab.com/api/v1/payment/create-session";
$headers = ["Authorization: API-KEY " . $api_key];
$payload = ["items" => $items];
$ch = curl_init($endpoint);
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_POST, true);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($payload));
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
if (curl_getinfo($ch, CURLINFO_HTTP_CODE) == 200) {
return json_decode($response, true);
} else {
return ["error" => "HTTP Error: " . curl_getinfo($ch, CURLINFO_HTTP_CODE), "message" => $response];
}
curl_close($ch);
}
}
if your session were success you will be redirected to the Payment Page will look like this
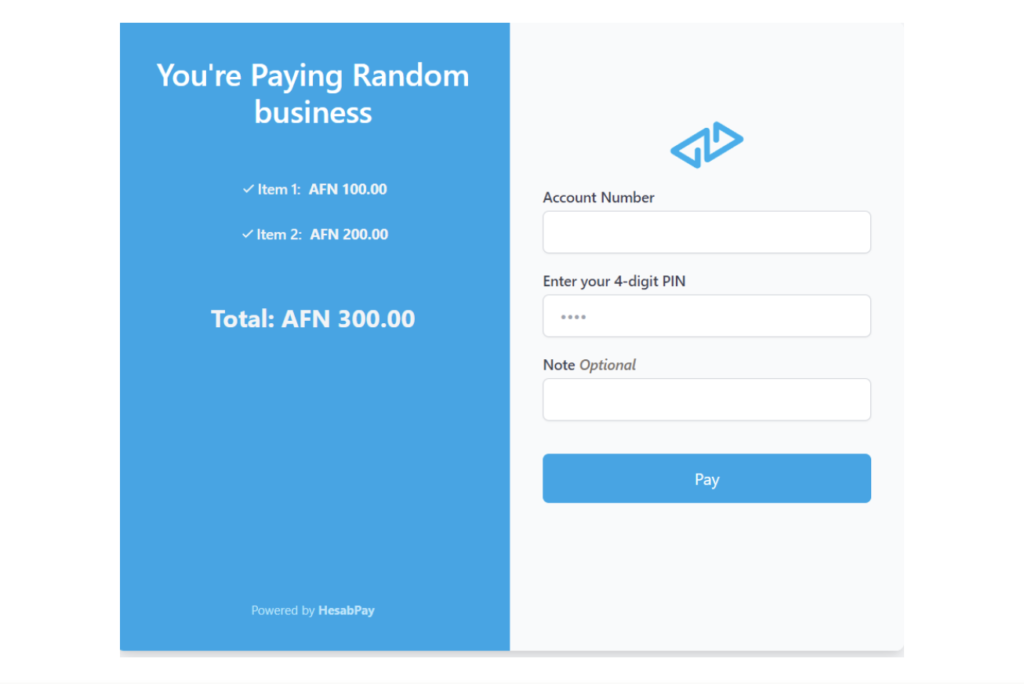
Your customer will be redirected to the payment page mentioned above there will be your business name ,items & total price user/customer will enter his/her hesabpay account number and their 4 digit pin to make payment on your platform.
at the last lets setup webhook? what webhook is please check on Hesabpay developer portal
first you need to create public endpoint on your server(hosting)
eg: xyz.com/webhook.php
once you created webhook.php
add the following code to test is your server receives transection json data from hesabpay.
<?php
// Get the raw POST data
$data = file_get_contents('php://input');
// Log the data to a file
$logFile = 'logs.txt';
if (file_put_contents($logFile, $data . PHP_EOL, FILE_APPEND) !== false) {
// Return a response with the logged data
echo 'Data logged successfully. Received data: ' . $data;
} else {
echo 'Failed to log data.';
}
?>
above code will write logs in logs.txt once you made a test payment you will received transaction data like this
{ "status_code": 10, "success": true, "message": "Operation successful", "sender_account": "793111416", "transaction_id": "0328379001707719668", "amount": 1, "memo": "random test memo", "signature": "d44ec5b76dcd5b367807f9b582b718a8b1971638ea72e017132281a8b70d6229", "timestamp": "1707719607", "transaction_date": "2024-02-12 11:04:28"}
then you need to verify signature with hesabpay use the following code to verify signature
<?php
// Get the raw POST data
$data = file_get_contents('php://input');
// Log the data to a file
$logFile = 'logs.txt';
if (file_put_contents($logFile, $data . PHP_EOL, FILE_APPEND) !== false) {
// Return a response with the logged data
echo 'Data logged successfully. Received data: ' . $data;
// Decode the JSON data to an array
$decodedData = json_decode($data, true);
if ($decodedData === null) {
echo 'Failed to decode JSON data.';
exit;
}
// Send the signature and timestamp for verification
$url = "https://api-sandbox.hesab.com/api/v1/hesab/webhooks/verify-signature";
$api_key = "your api key here";
$dataToSend = array(
"signature" => $decodedData["signature"],
"timestamp" => $decodedData["timestamp"]
);
$headers = array(
"Authorization: API-KEY $api_key",
"Content-Type: application/json"
);
$ch = curl_init($url);
curl_setopt($ch, CURLOPT_POST, 1);
curl_setopt($ch, CURLOPT_POSTFIELDS, json_encode($dataToSend));
curl_setopt($ch, CURLOPT_HTTPHEADER, $headers);
curl_setopt($ch, CURLOPT_RETURNTRANSFER, true);
$response = curl_exec($ch);
$httpcode = curl_getinfo($ch, CURLINFO_HTTP_CODE);
curl_close($ch);
// Log the response
$logFile = 'verifiedlogs.txt';
file_put_contents($logFile, $response . PHP_EOL, FILE_APPEND);
// Check if the request was successful
if ($httpcode == 200) {
echo "Success: $response";
} else {
echo "Error: $response";
}
} else {
echo 'Failed to log data.';
}
?>
?>
Note: if you need Hesabpay Payment Integration in your Website/Mobile app to receive Business Payments you can hire our Waqad Soft Developers (+93 70 033 0730/ +93747544242) thank you.
1 thought on “HesabPay Payment Integration in Laravel PHP”