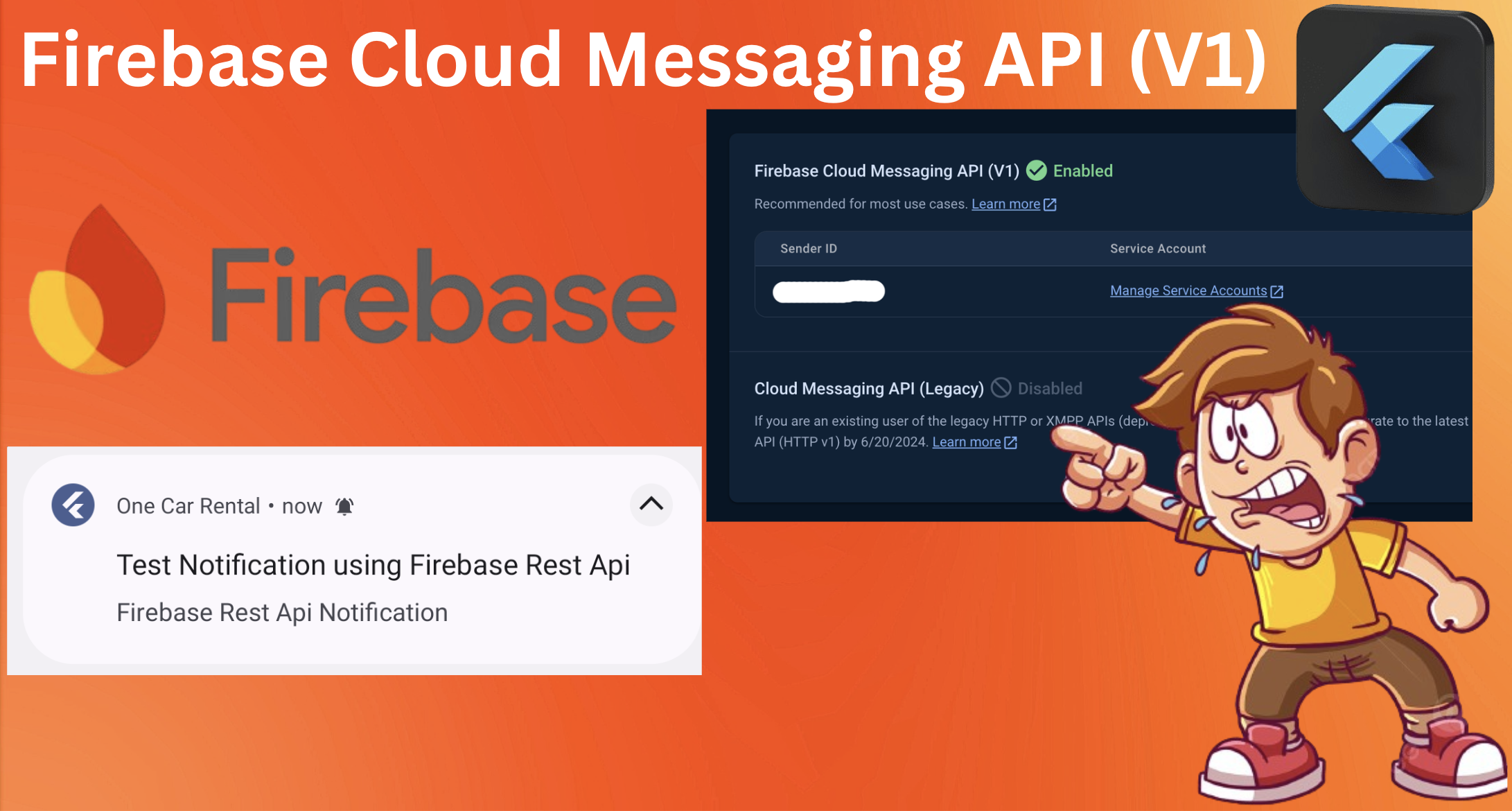
Firebase Cloud Messaging API V1 is an essential tool for developers aiming to enhance user engagement through notifications and messages across various platforms. this supports multiple device types and excels in managing complex messaging scenarios. In this guide, you will discover the core features of Firebase Cloud Messaging API V1, including real-time message delivery, customizable notifications, and much more. We will provide a comprehensive, step-by-step implementation guide to help you get started with the FCM API V1. This guide will ensure that you make the most of the API’s capabilities and seamlessly integrate it into your applications.
how to send “firebase cloud Messaging using Firebase Rest Api”?
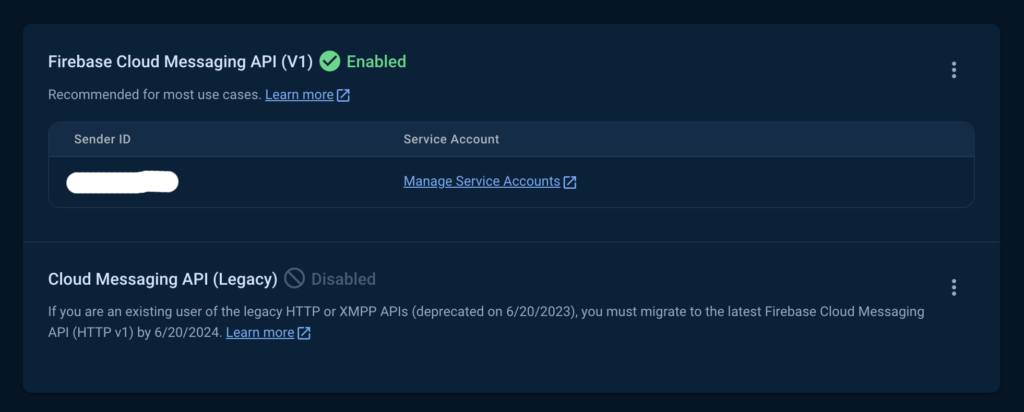
step 1: open your brand new firebase project go to project settings >> Service accounts
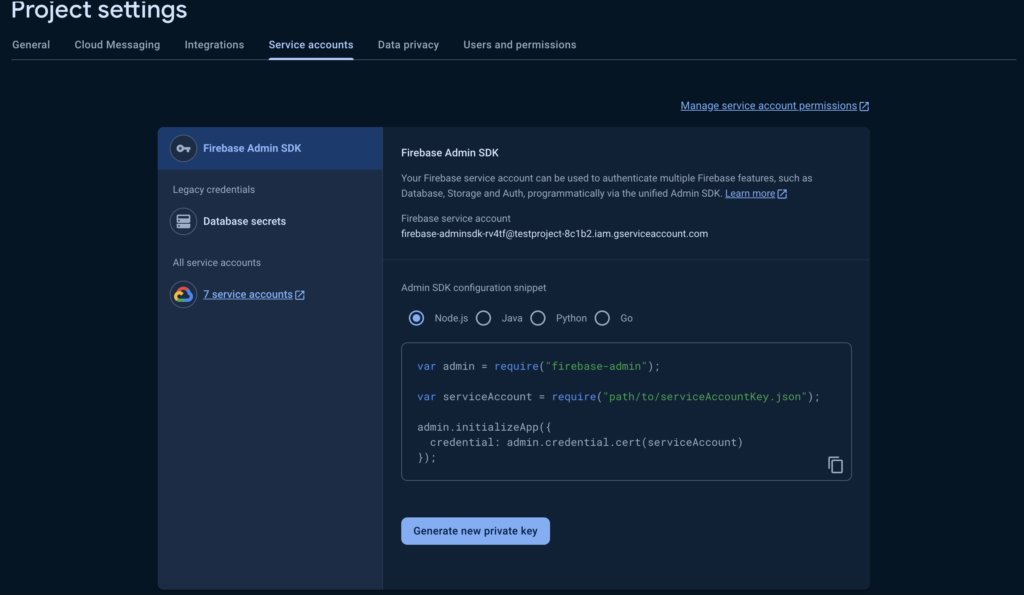
then select Node.js and click on Generate new private Key.
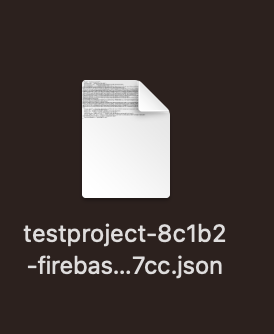
your json file will look like this now lets create your new flutter project.
flutter create pushnotifications
Step 2: add the following dependencies
firebase_messaging: ^15.0.4
googleapis: ^13.1.0
googleapis_auth: ^1.4.1
Step 3: create sendnotification.dart file and past code below
import 'dart:convert';
import 'dart:io';
import 'package:http/http.dart' as http;
import 'package:googleapis_auth/auth_io.dart' as auth;
Future<void> sendNotification(String title, String body, String screen, List<String> tokens) async {
final accountCredentials = auth.ServiceAccountCredentials.fromJson(r'''
you will replace json file like this which you downloaded recently. you can use my project exact for testing
{
"type": "service_account",
"project_id": "testproject-8c1b2",
"private_key_id": "e96522ef1420abb0c130521c67a5cd28c48af35a",
"private_key": "-----BEGIN PRIVATE KEY-----\nMIIEvgIBADANBgkqhkiG9w0BAQEFAASCBKgwggSkAgEAAoIBAQCnnfSyqsxVRNxX\nN6Ndy0LoCG45SKO7BQ4NekjOsqtVkXGNEf7lHM9nyB5cMQLArTrhY9WaxPoOQJBo\ntShfHbzrYIF6gSesIjpkK09vD4YxLU74oQAC5NeuOW1blWreq4/oRhsC/L3UZV7C\npxCJGO3GJBtoWUIuU3tAcIQuoQMk97GnrY+ElCVBxvXwpqGZ1NJEsML4R5Ilmof/\nzvleiFiKoy6KFAtsEy6oezjyE/lxUvh/eCIt8nL/mOYgX39WjUnV5s5QwDocb5FU\nAGylPrXgfpnzCpe3ddYzXvpe9uD9j9lQ54myOnbSlVDKBfK9YppF1VuVPTIX8Tv8\nRqnCHl6rAgMBAAECggEATyf/pp6VR5Fw8vlR+5nhe1173C6LL+dXtxKwLnjfA9Zf\nRw0gEEfWRL8TY1s73w0P2OACQIhURIXGDMS8j4qmLIwTKWyz9QznAWYYqaytpSyt\n9ilmLXevyVXSaKU75nMjzz/IQtVjulmy8f7ehrgQlfl4PgOl
BuaitpBMRA8CwqI4\n8imckSNTJxY8gMhiPBadHjfiWFDIRNSajK1aw3BuhhetppnxO87044GL+XSYXj4L\nCgeijnr0nYW/UVlxsONm3yxnlP+MgEaQkO/NtwEFsP8JyATxzZyyeGCHhwwycvET\neKd1j50WQv+XI0JNw6b5uZEKLa9WGW85pLyO6fW6QQKBgQDV1FmzIr252HEjprq7\nDCXRivjsxmJJs3I03kjkNi4AsEMYXzmAHGC/cbwMaWb9pGKS5H1kOS6CxxNpiUAk\nDerMY/gS/Zl4yzxQpfYGLcgFom+YsfLeE18efUPDhdDukpmWnRbFm6WS4MnSAhHY\nQIeZXEALQA1HV/MPg9Lyq6vDwQKBgQDIrHeofzPS0q63BX8Bccfj41x00ygRE0em\naR5tqBJ2vBAHSvinaWuADhWcmMA5gv8ReqYR10omzPv6USF1C/yuRGZKY+EmcC5D\nGFoGZPIhm5uaK3PUiRDMdKye1ED2OciHJtC13mu3OUZOSDqiev8dLhkuR/cKHyZt\nL/Z0MhLNawKBgQC0XRoMcBF44YkNeWWowOZlicPOdMgTQrnivlzdopXLHuJ5fFkU\nJtu0oErubTniWPaM0Q6zq0gAJ8vICW2A+/xBy1FFM4Rwz31gyZxsOdGubwWS1wb1\nK4HgRekS050Yn6/Ny1OZ+1/+NH4IFpbWwhee1KFw2+ZXd2gefiNBbmzEAQKBgQCU\nl6DDE+273mWhAGDbIPVyuZhsqS6x9iTIBG6TpDCH2xnDTLByxozjdYprOnCcJXPZ\n7YerDk/KC19KK2kq0oV4RQ25Z9wAKnEXHaELuxPP7vl5X1OVvSjDPGHV+jVgHclu\nyj8hbGE2eyKkNLe2OH1PjiL3MjKrPAWoNCu6PpnNoQKBgFpwLFjuspkgYd8nNVsp\njOl5f3JrouH5qhXT3vID7k1QyOZq90hjcIDZnpMZbqb7GiJgcb5KxSm+2KC1ku3z\nZx3fS4wGv/PFbau2mnzTRSRHaXOlGnlbWJY3voBNO8thlHAKYDQrqtINj582vA2y\nEIP3cQDvn92I4yCD7MtIO6NO\n-----END PRIVATE KEY-----\n",
"client_email": "firebase-adminsdk-rv4tf@testproject-8c1b2.iam.gserviceaccount.com",
"client_id": "108819671381699474946",
"auth_uri": "https://accounts.google.com/o/oauth2/auth",
"token_uri": "https://oauth2.googleapis.com/token",
"auth_provider_x509_cert_url": "https://www.googleapis.com/oauth2/v1/certs",
"client_x509_cert_url": "https://www.googleapis.com/robot/v1/metadata/x509/firebase-adminsdk-rv4tf%40testproject-8c1b2.iam.gserviceaccount.com"
}
''');
// Define the required scopes for Firebase Cloud Messaging API
final scopes = ['https://www.googleapis.com/auth/firebase.messaging'];
// Obtain an authenticated HTTP client
final authClient =
await auth.clientViaServiceAccount(accountCredentials, scopes);
final fcmUrl =
'https://fcm.googleapis.com/v1/projects/testproject-8c1b2/messages:send';
for (String token in tokens) {
final response = await authClient.post(
Uri.parse(fcmUrl),
headers: {'Content-Type': 'application/json'},
body: jsonEncode({
"message": {
"token": token,
"data": {"screen": screen},
"notification": {
"title": title,
"body": body
}
}
}),
);
if (response.statusCode == 200) {
print('Notification sent successfully to $token!');
} else {
print('Failed to send notification to $token. Error: ${response.body}');
}
}
}
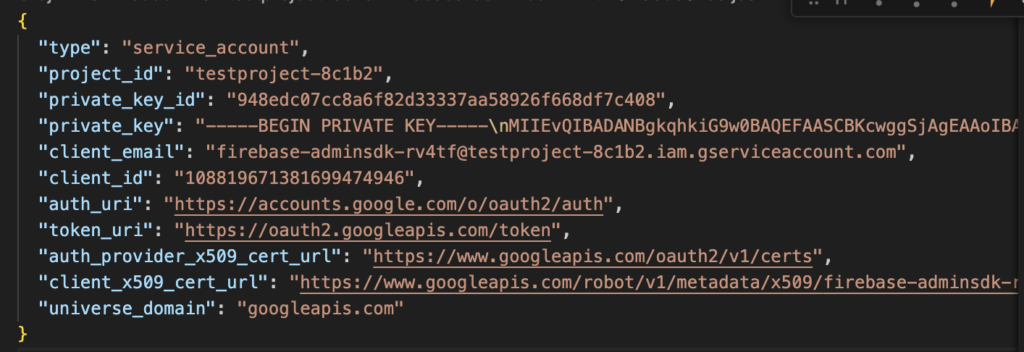
your downloaded json file will look like this you need to replace in above code
now lets trigger the function to send notification
we will just create a simple send button to send notification you can modify as per your requirements
create bellow function in your screen to send notification by calling the given function
sendNotification(
'New Booking Available',
'A new booking has been created. Check it out!',
'home',
tokens,
);
you can modify as per your requirements as mentioned above . also you will need to pass users tokens to send them notification you can pass one or more as i am using list for tokens
if you have any confusion you can comment or text me on my facebook page to resolve thank you for visiting my blog.
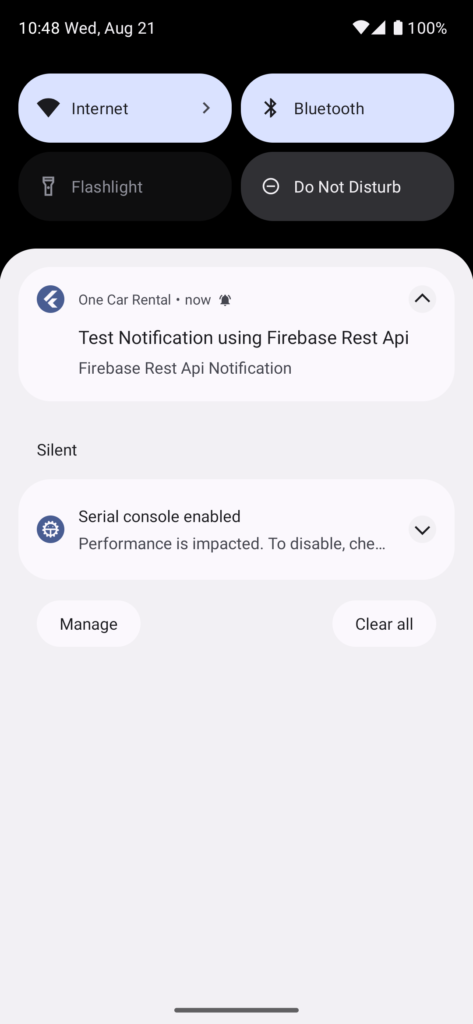
very helpful and easy to understand.
thank you for your valuable feedback keep visiting our site for more interesting stuffs also we have pushed our flutter beginnerโs to advance course with examples
https://codericu.com/category/flutter-for-beginners/