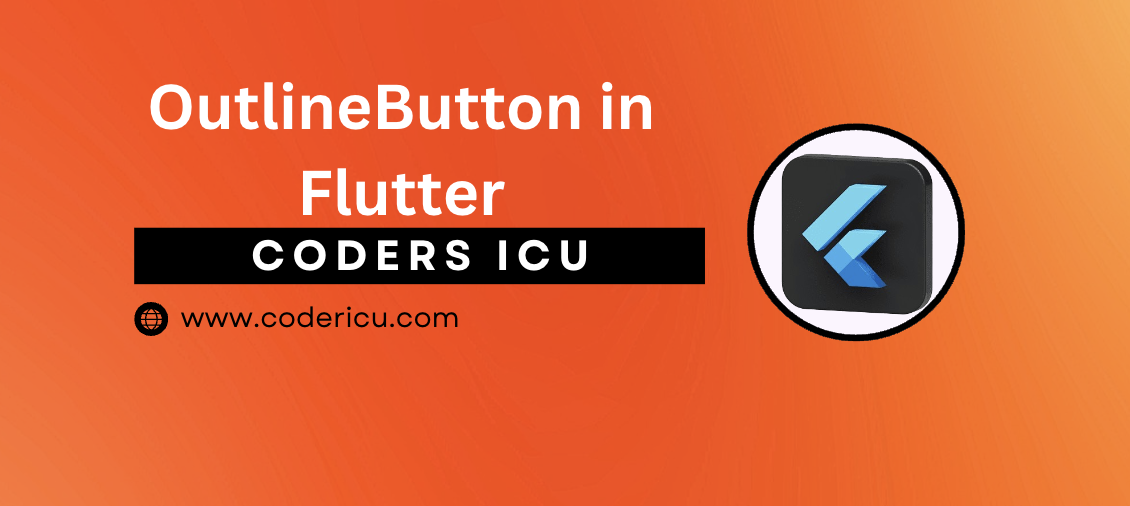
In Flutter, an OutlineButton is a type of button that displays a border with a transparent background, allowing the underlying content to be visible. It’s essentially a button with an outline or border but without a solid background color.
You may make buttons that look lightweight and minimalistic by using the OutlineButton widget.
It’s crucial to remember, though, that OutlineButton has been superseded by OutlinedButton as of Flutter 2.0. Similar functionality is provided by the OutlinedButton widget, which also has updated design features and more flexibility
Properties
- child: The content of the button (e.g., text, icon)
- onPressed: The callback function when the button is pressed
- borderSide: The color and width of the border
- borderRadius: The radius of the button’s corners
OutlinedButton.styleFrom()
properties:
- foregroundColor: The color of the button’s text and icon.
- backgroundColor: The background color of the button (optional).
side
: The border color and width.
- color: The color of the border.
- width: The width of the border.
padding: The padding inside the button.
horizontal
: The horizontal padding.vertical
: The vertical padding.
RoundedRectangleBorder
: A rectangular border with rounded corners.borderRadius
: The radius of the rounded corners.
Example:
OutlinedButton(
onPressed: () {
// Handle button press here
},
style: OutlinedButton.styleFrom(
foregroundColor: Colors.blue,
side: BorderSide(
color: Colors.blue, width: 2), // Border color and width
padding: EdgeInsets.symmetric(
horizontal: 20, vertical: 10), // Padding
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10), // Rounded corners
),
),
child: Text('Outlined Button'),
),
Code :
import 'package:flutter/material.dart';
class OutlinebuttonDemo extends StatefulWidget {
const OutlinebuttonDemo({Key? key}) : super(key: key);
@override
State<OutlinebuttonDemo> createState() => _OutlinebuttonDemoState();
}
class _OutlinebuttonDemoState extends State<OutlinebuttonDemo> {
@override
Widget build(BuildContext context) {
return SafeArea(
child: Scaffold(
appBar: AppBar(
title: Text(
"Outline Button",
style: TextStyle(color: Colors.white, fontWeight: FontWeight.bold),
),
flexibleSpace: Container(
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.deepPurple, Colors.orangeAccent],
begin: Alignment.topLeft,
end: Alignment.topRight,
)),
),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Center(
child: OutlinedButton(
onPressed: () {
// Handle button press here
},
style: OutlinedButton.styleFrom(
foregroundColor: Colors.blue,
side: BorderSide(
color: Colors.blue, width: 2), // Border color and width
padding: EdgeInsets.symmetric(
horizontal: 20, vertical: 10), // Padding
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10), // Rounded corners
),
),
child: Text('Outlined Button'),
),
),
],
),
),
);
}
}
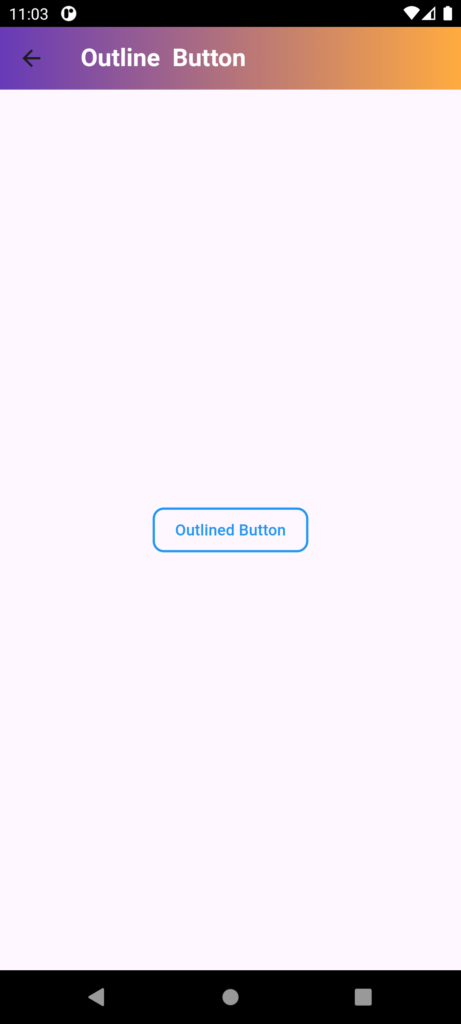