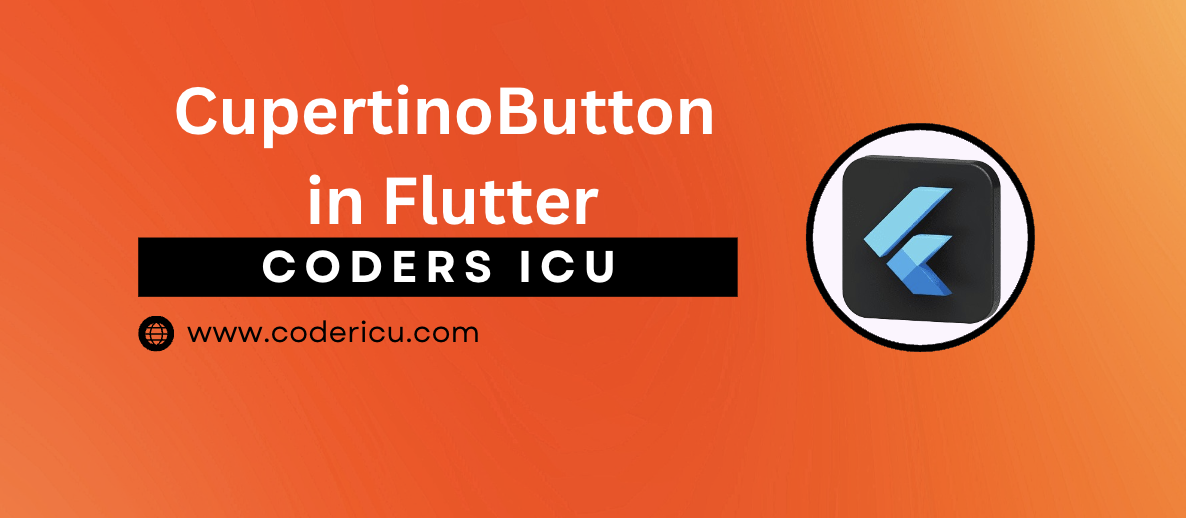
A button that follows the design guidelines of IOS Cupertino design language, is used in iOS apps.
CupertinoButton is used in iOS-themed apps, while ElevatedButton and OutlinedButton are used in Material-themed apps.
Properties
- child: The button’s content (e.g., text, icon).
- onPressed: The callback function when the button is pressed.
- color: The background color of the button.
- disabledColor: The background color of the button when disabled.
padding
: The padding around the content.- borderRadius: The radius of the button’s corners.
- minSize: The minimum size of the button.
Types:
- CupertinoButton: The default Cupertino button.
- CupertinoButton
.
filled: A filled Cupertino button with a solid background color. - CupertinoButton
.
outlined: An outlined Cupertino button with a border.
CupertinoButton(
onPressed: () {
// Handle button press
},
color: CupertinoColors.activeBlue,
borderRadius: BorderRadius.circular(8),
padding: EdgeInsets.symmetric(horizontal: 20, vertical: 10),
child: Text('Cupertino Button'),
),
Code:
import 'package:flutter/cupertino.dart'; // Importing Cupertino widgets
import 'package:flutter/material.dart'; // Importing Material widgets
class CupertinoButtonDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
return CupertinoApp(
// The main entry point for a Cupertino (iOS-style) app
home: CupertinoPageScaffold(
// A basic page layout with a navigation bar and content
navigationBar: CupertinoNavigationBar(
// The navigation bar at the top of the page
middle: Text('Cupertino Button Example'), // Title of the navigation bar
),
child: Center(
// Centers the child widget within the available space
child: CupertinoButton(
// CupertinoButton is an iOS-style button
onPressed: () {
// Action to be executed when the button is pressed
// Implement the functionality here
},
color: CupertinoColors.activeBlue, // Background color of the button
borderRadius: BorderRadius.circular(8), // Rounded corners of the button
padding: EdgeInsets.symmetric(horizontal: 20, vertical: 10), // Padding inside the button
child: Text('Cupertino Button'), // Text displayed inside the button
),
),
),
);
}
}
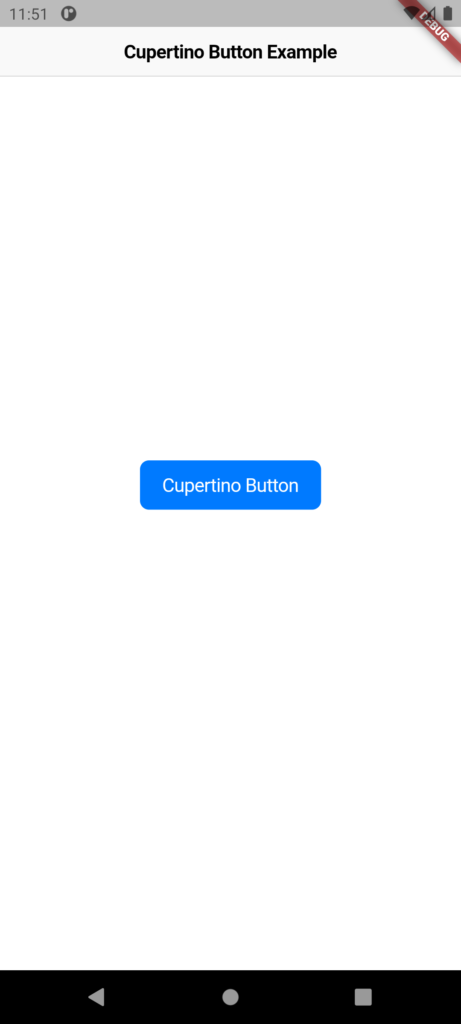