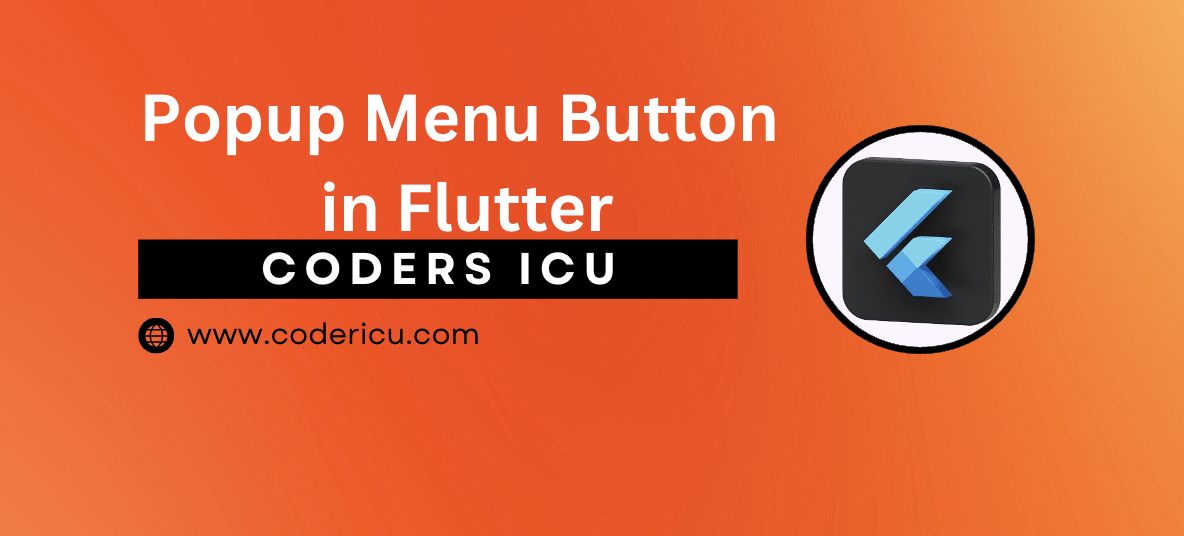
A button that displays a popup menu when Clicked, is used to provide a list of items to the user.
Properties:
- icon: The icon to display on the button.
- items: The list of menu items to display.
- onSelected: The callback function when a menu item is selected.
- onCanceled: The callback function when the menu is dismissed without selecting an item.
- tooltip: A message to display when the button is long-pressed.
- elevation: The elevation of the pop up menu.
- padding: The padding around the popup menu.
- child: The button’s content (e.g., text, icon).
PopupMenuButton<String>(
// Callback function executed when a menu item is selected
onSelected: (String choice) {
_onSelected(context, choice); // Handles the selected menu item
},
// Function that builds the menu items
itemBuilder: (BuildContext context) {
// List of menu item choices
return ['First', 'Second', 'Third'].map((String choice) {
return PopupMenuItem<String>(
value: choice, // Value of the menu item
child: Text(choice), // Text displayed in the menu item
);
}).toList(); // Converts the iterable to a list
},
// Icon displayed on the button
icon: Icon(Icons.more_vert),
// Background color of the menu button
color: Colors.white,
// Elevation of the button, which affects the shadow depth
elevation: 5,
),
Code:
import 'package:flutter/material.dart';
class PopupbuttonDemo extends StatefulWidget {
@override
State<PopupbuttonDemo> createState() => _PopupbuttonDemoState();
}
class _PopupbuttonDemoState extends State<PopupbuttonDemo> {
// Method to handle the selection of a menu item
void _onSelected(BuildContext context, String choice) {
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(
content: Text('You selected: $choice'), // Corrected string interpolation to include the dollar sign
),
);
}
@override
Widget build(BuildContext context) {
return SafeArea(
child: Scaffold(
appBar: AppBar(
title: Text('Popup MenuButton Code'), // Title displayed in the AppBar
flexibleSpace: Container(
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.deepPurple, Colors.orangeAccent], // Gradient colors for the AppBar background
begin: Alignment.topLeft, // Start of the gradient
end: Alignment.topRight, // End of the gradient
),
),
),
),
body: Center(
// Centers the PopupMenuButton in the available space
child: PopupMenuButton<String>(
// Callback function executed when a menu item is selected
onSelected: (String choice) {
_onSelected(context, choice); // Calls the method to handle the selected choice
},
// Function that builds the menu items
itemBuilder: (BuildContext context) {
return ['First', 'Second', 'Third'].map((String choice) {
// Creates a PopupMenuItem for each choice
return PopupMenuItem<String>(
value: choice, // Value of the menu item
child: Text(choice), // Text displayed in the menu item
);
}).toList(); // Converts the iterable to a list
},
// Icon displayed on the PopupMenuButton
icon: Icon(Icons.more_vert),
// Background color of the PopupMenuButton
color: Colors.white,
// Elevation of the PopupMenuButton, affecting shadow depth
elevation: 5,
),
),
),
);
}
}
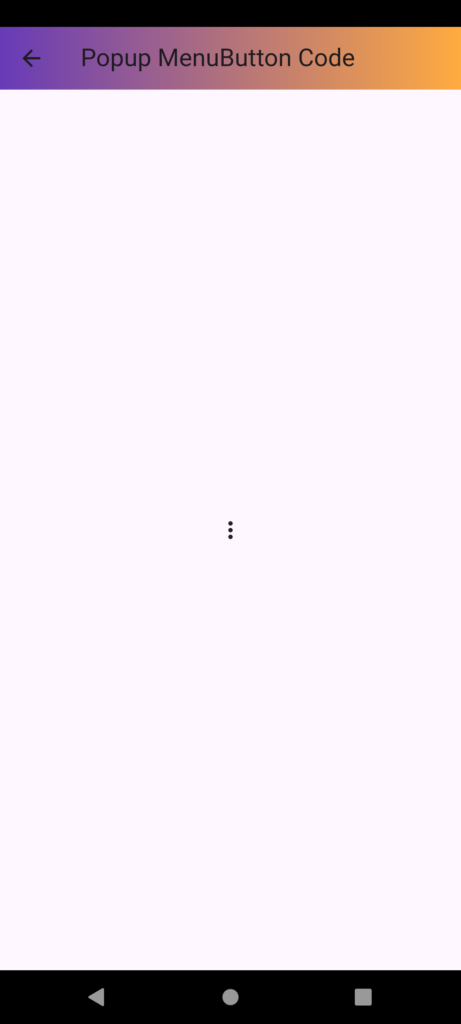
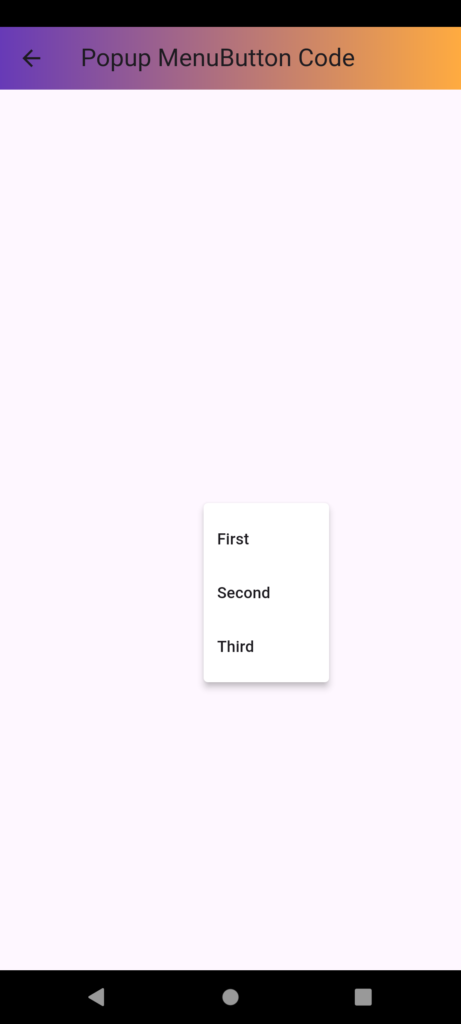