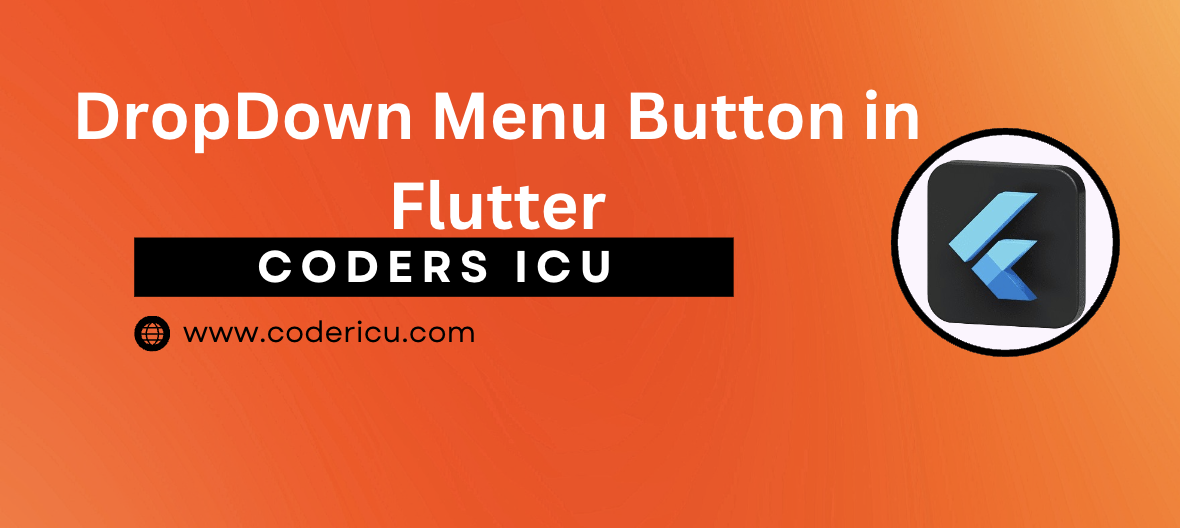
A button that displays a dropdown menu when pressed, used to select a single value from a list.
DropdownButton vs PopupMenuButton:
- Selection: While PopupMenuButton offers a list of alternatives to do various tasks, DropdownButton allows selecting a single value from a list.
- Menu Display: Whereas PopupMenuButton shows a popup menu that may be placed anywhere on the screen, DropdownButton shows a dropdown menu beneath the button.
- Value Storage:
DropdownButton
stores the selected value, whilePopupMenuButton
doesn’t store any value. - Usage:
DropdownButton
is used for selecting an option (e.g., selecting a country from a list), whilePopupMenuButton
is used for performing actions (e.g., editing or deleting an item). - UI:
DropdownButton
typically displays the selected value on the button, whilePopupMenuButton
displays an icon or text on the button.
Properties:
- items: The list of menu items to display.
- value: The currently selected value.
- onChanged: The callback function when a new value is selected.
- hint: A message to display when no value is selected.
- elevation: The elevation of the dropdown menu.
- icon: The icon to display on the button.
- iconSize: The size of the icon.
- isExpanded: Whether the dropdown menu is expanded.
DropdownButton<String>(
isExpanded: true,
value: _selectedValue,
icon: Icon(Icons.arrow_downward),
iconSize: 24,
elevation: 16,
style: TextStyle(color: Colors.deepPurple),
underline: Container(
height: 2,
color: Colors.deepPurpleAccent,
),
onChanged: (String? newValue) {
setState(() {
_selectedValue = newValue!;
});
},
items: <String>['One', 'Two', 'Three', 'Four']
.map<DropdownMenuItem<String>>((String value) {
return DropdownMenuItem<String>(
value: value,
child: Text(value),
);
}).toList(),
),
import 'package:flutter/material.dart';
class DropButton extends StatefulWidget {
// Constructor for the DropButton widget
const DropButton({Key? key}) : super(key: key);
@override
State<DropButton> createState() => _DropButtonState(); // Creates the mutable state for this widget
}
class _DropButtonState extends State<DropButton> {
// Holds the currently selected value of the dropdown button
String _selectedValue = 'One';
@override
Widget build(BuildContext context) {
return Scaffold(
// Provides the basic visual structure of the app, including an AppBar and body
appBar: AppBar(
// The AppBar at the top of the screen
title: Text(
"Dropdown Button", // Title displayed in the AppBar
style: TextStyle(
color: Colors.white, // Color of the title text
fontWeight: FontWeight.bold, // Font weight of the title text
),
),
flexibleSpace: Container(
// FlexibleSpace allows for custom decorations behind the AppBar content
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.deepPurple, Colors.orangeAccent], // Gradient colors
begin: Alignment.topLeft, // Starting point of the gradient
end: Alignment.topRight, // Ending point of the gradient
),
),
),
),
body: Column(
// Column widget arranges its children vertically
mainAxisAlignment: MainAxisAlignment.center, // Centers children vertically in the column
children: [
DropdownButton<String>(
// The dropdown button widget
isExpanded: true, // Expands the dropdown button to fill the available horizontal space
value: _selectedValue, // The currently selected value of the dropdown
icon: Icon(Icons.arrow_downward), // Icon displayed on the dropdown button
iconSize: 24, // Size of the dropdown icon
elevation: 16, // Elevation of the dropdown menu (shadow effect)
style: TextStyle(color: Colors.deepPurple), // Style of the text displayed in the dropdown button
underline: Container(
height: 2, // Height of the underline below the dropdown button
color: Colors.deepPurpleAccent, // Color of the underline
),
onChanged: (String? newValue) {
// Callback function when a new value is selected
setState(() {
// Updates the state with the new value
_selectedValue = newValue!;
});
},
items: <String>['One', 'Two', 'Three', 'Four']
.map<DropdownMenuItem<String>>((String value) {
// Maps each value to a DropdownMenuItem
return DropdownMenuItem<String>(
value: value, // Value of the menu item
child: Text(value), // Text displayed in the menu item
);
}).toList(), // Converts the iterable to a list of DropdownMenuItem
),
],
),
);
}
}