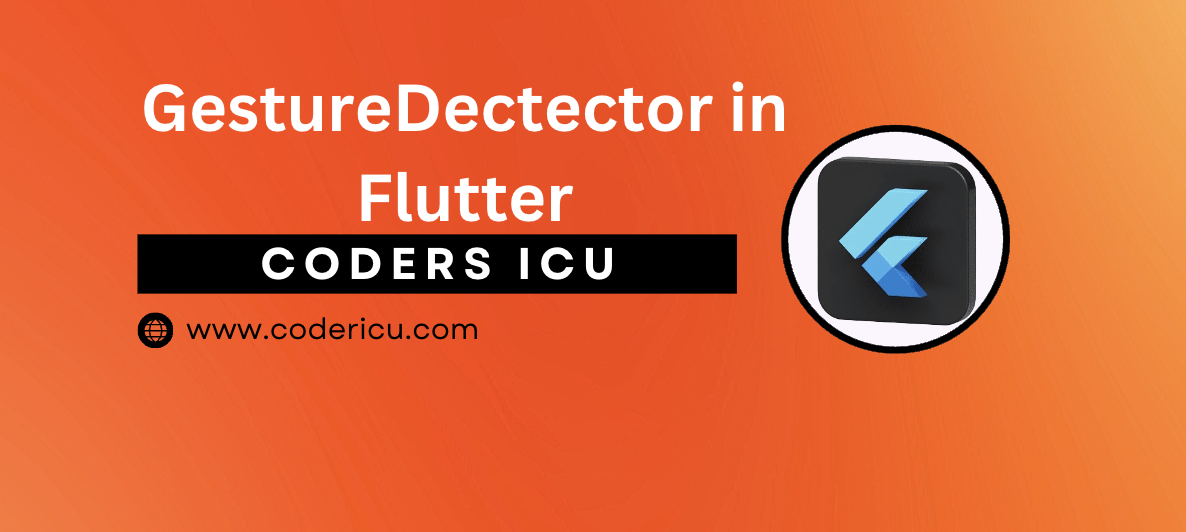
GestureDetector is a widget in Flutter that detects gestures, such as taps, swipes, and pinches, and allows you to respond to them.
It takes a child widget and a set of gesture recognizers, which define the gestures to detect. When a gesture is detected, the corresponding callback is triggered.
It is used to detect various user gestures and interactions. It provides a way to handle touch and gesture events on a widget, allowing developers to create interactive and responsive user interfaces.
- GestureDetector listens for different types of gestures (e.g., taps, swipes, pinches) and responds to them with specified callback functions.
Common Gestures
- onTap: Called when the user taps the widget.
- onDoubleTap: Called when the user double-taps the widget.
- onLongPress: Called when the user long-presses the widget.
- onSwipe: Called when the user swipes the widget.
- onPan: Called when the user pans (drags) the widget.
GestureDetector is an invisible wrapper that enhances other widgets with gesture recognition capabilities. It doesn’t have a visual appearance but empowers its child widgets to respond to touch events like taps, swipes, and pinches, triggering specified actions
Example
// Create a GestureDetector widget to detect gestures
GestureDetector(
// Callback for a single tap
onTap: () {
// Print a message when the widget is tapped
print(' tapped!');
},
// Callback for double tap
onDoubleTap: () {
// Print a message when the widget is double-tapped
print('Widget double-tapped!');
},
// Callback for long press
onLongPress: () {
// Print a message when the widget is long-pressed
print(' long-pressed!');
},
// The child widget that will be wrapped with gesture detection
child: Container(
// Set the container's background color
color: Colors.blue,
// Set the container's width and height
width: 100,
height: 100,
// Center the child widget
child: Center(
// Display a text widget
child: Text(
// Text to display
'Tap me!',
// Text style
style: TextStyle(
// Set text color to white
color: Colors.white,
),
),
),
),
);
Code
import 'package:flutter/material.dart';
// Define a StatefulWidget called GestiredetectorbuttonDemo
class GestiredetectorbuttonDemo extends StatefulWidget {
// Constructor for the StatefulWidget
const GestiredetectorbuttonDemo({Key? key}) : super(key: key);
@override
State<GestiredetectorbuttonDemo> createState() => _GestiredetectorbuttonDemoState();
}
// State class for GestiredetectorbuttonDemo
class _GestiredetectorbuttonDemoState extends State<GestiredetectorbuttonDemo> {
@override
Widget build(BuildContext context) {
return SafeArea(
// SafeArea ensures that the child widgets are displayed within the visible screen area
child: Scaffold(
// Scaffold provides a high-level structure for the visual interface
appBar: AppBar(
// AppBar is a material design app bar at the top of the screen
title: Text(
"GestureDetector Button",
style: TextStyle(
color: Colors.white, // Set the text color to white
fontWeight: FontWeight.bold, // Make the text bold
),
),
flexibleSpace: Container(
// Container inside AppBar to apply a gradient decoration
decoration: BoxDecoration(
gradient: LinearGradient(
// Apply a linear gradient to the AppBar
colors: [Colors.deepPurple, Colors.orangeAccent], // Gradient colors
begin: Alignment.topLeft, // Gradient starts from the top left
end: Alignment.topRight, // Gradient ends at the top right
),
),
),
),
body: Column(
// Column arranges its children vertically
mainAxisAlignment: MainAxisAlignment.center, // Center the children vertically
children: [
Center(
// Center widget aligns its child to the center of the available space
child: GestureDetector(
// GestureDetector listens for gestures and executes the corresponding callback
onTap: () {
// Callback when the widget is tapped
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('GestureDetector tapped!')), // Show a SnackBar message
);
},
onDoubleTap: () {
// Callback when the widget is double-tapped
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('GestureDetector double tapped!')), // Show a SnackBar message
);
},
onLongPress: () {
// Callback when the widget is long-pressed
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('GestureDetector long pressed!')), // Show a SnackBar message
);
},
child: Container(
// Container to hold the visual representation of the GestureDetector
padding: EdgeInsets.all(20), // Add padding inside the container
decoration: BoxDecoration(
gradient: LinearGradient(
// Apply a gradient background to the container
colors: [Colors.deepPurple, Colors.orangeAccent], // Gradient colors
),
borderRadius: BorderRadius.circular(10), // Rounded corners with a radius of 10
),
child: Text(
"GestureDetector Button",
style: TextStyle(
color: Colors.white, // Set the text color to white
),
),
),
),
)
],
),
),
);
}
}
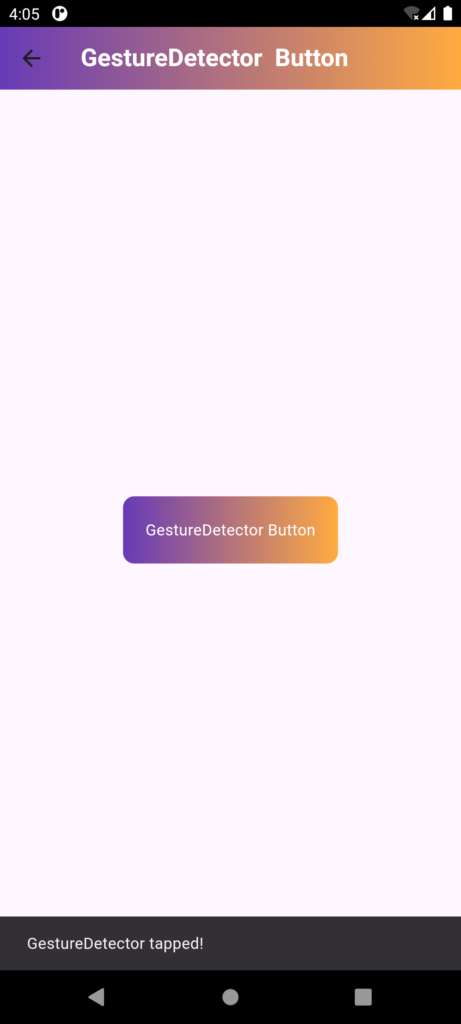