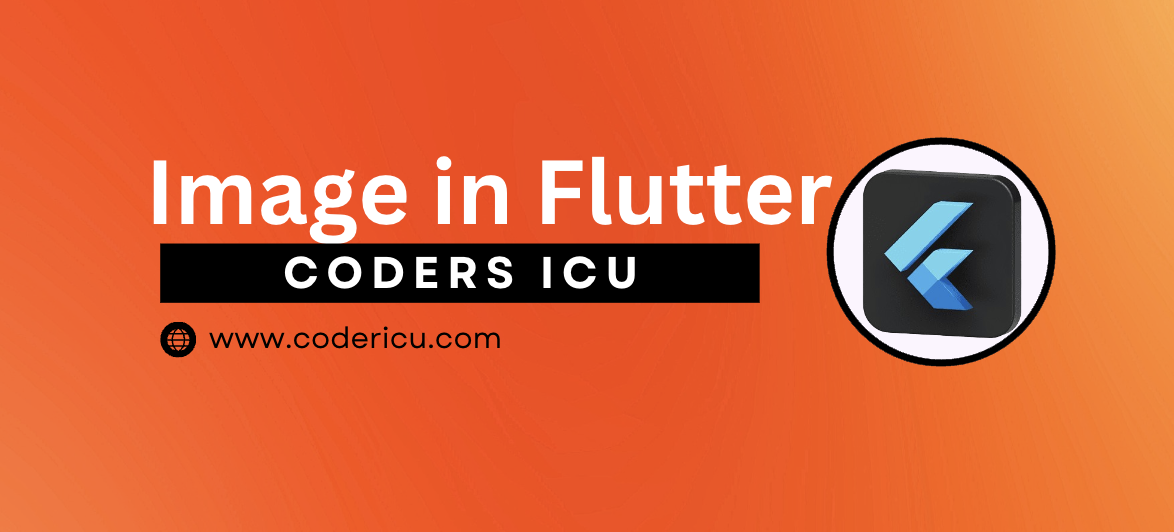
In Flutter, the Image widget is used to display images. It can load images from various sources, including:
- Assets (images bundled with the app)
- Files (stored on the device)
- Network (loaded from a URL)
- Memory (loaded from a byte array)
The Image widget provides various properties to customize the image display, such as:
- width and
height
to set the image size - fit to control how the image is scaled to fit the widget’s size
- alignment to position the image within the widget
- repeat to repeat the image in a specific direction
Steps to use AssetImage:
Add Image Files to Your Project:
- Create an Assets Directory:
- Create a directory for your images. Typically, you create an assets folder at the root of your project. Inside this, you can create subdirectories if needed.
your_project/
└── assets/
└── images/
└── example_image.png
Place Your Images:
- Place Your Images:
- Add the image files to the appropriate directory. For example, place example_image.png inside the
assets/images/ directory.
2. Configure pubspec.yaml :
Open pubspec.yaml :
- This file is located in the root of your Flutter project.
3 Declare the Assets:
- Under the flutter section in your pubspec, specify the asset files or directories that you want to include in your app.
Example for individual files:
flutter:
assets:
- assets/images/example_image.png
- assets/images/
# Make sure the indentation is correct; assets: should be indented under flutter
Example for all files in a directory:
flutter:
assets:
- assets/images/
# Make sure the indentation is correct; assets: should be indented under flutter
4 Save and Get Dependencies:
- After editing
pubspec.yaml
, save the file. Run flutterpub get
to update the project with the new asset configuration.
Example of AssetImage
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Image Asset Example'),
),
body: Center(
child: Image.asset(
'assets/images/example_image.png', // Path to the asset
width: 100, // Optional: specify width
height: 100, // Optional: specify height
fit: BoxFit.cover, // Optional: specify how the image should be fit
),
),
);
}
}
Network Image:
Using network images in Flutter allows you to display images that are hosted on the internet. This is useful for dynamic content where the image URL may change or be provided by a remote server
To display an image from the internet, you use the Image.network widget.
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Network Image Example'),
),
body: Center(
child: Image.network(
'https://example.com/image.png', // Replace with your image URL
width: 150, // Optional: specify width
height: 150, // Optional: specify height
fit: BoxFit.cover, // Optional: specify how the image should be fit
),
),
);
}
}
Handling Loading and Error States:
To manage image loading and error states, you can use the loadingBuilder and errorBuilder properties of the Image.network
widget.
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Network Image with Loading and Error Handling'),
),
body: Center(
child: Image.network(
'https://example.com/image.png', // Replace with your image URL
width: 150,
height: 150,
fit: BoxFit.cover,
loadingBuilder: (BuildContext context, Widget child, ImageChunkEvent? loadingProgress) {
if (loadingProgress == null) {
return child;
} else {
return Center(
child: CircularProgressIndicator(
value: loadingProgress.expectedTotalBytes != null
? loadingProgress.cumulativeBytesLoaded / (loadingProgress.expectedTotalBytes ?? 1)
: null,
),
);
}
},
errorBuilder: (BuildContext context, Object error, StackTrace? stackTrace) {
return Center(child: Icon(Icons.error));
},
),
),
);
}
}
Caching Network Images:
For better performance and to avoid reloading images every time they are displayed, you can use packages like cached_network_image to handle caching automatically
Add cached_network_image to your pubspec.yaml
:
dependencies:
cached_network_image: ^3.4.1
Use CachedNetworkImage:
import 'package:flutter/material.dart';
import 'package:cached_network_image/cached_network_image.dart';
// Define the MyHomePage widget, which is a StatelessWidget
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
// Build the widget tree
return Scaffold(
// Scaffold provides a basic structure for the app, including an AppBar and body
appBar: AppBar(
// AppBar is a material design app bar that holds the title and other widgets
title: Text('Cached Network Image Example'), // Title of the app bar
),
body: Center(
// Center widget centers its child within its parent
child: CachedNetworkImage(
// CachedNetworkImage is a widget provided by the cached_network_image package
// It handles loading, caching, and displaying network images
imageUrl: 'https://example.com/image.png', // Replace with the URL of the image you want to display
width: 150, // Width of the displayed image
height: 150, // Height of the displayed image
fit: BoxFit.cover, // BoxFit controls how the image should be fit within its container
placeholder: (context, url) => Center(
// Placeholder is a widget displayed while the image is loading
child: CircularProgressIndicator(), // Shows a loading spinner
),
errorWidget: (context, url, error) => Center(
// ErrorWidget is displayed if there is an error loading the image
child: Icon(Icons.error), // Displays an error icon
),
),
),
);
}
}