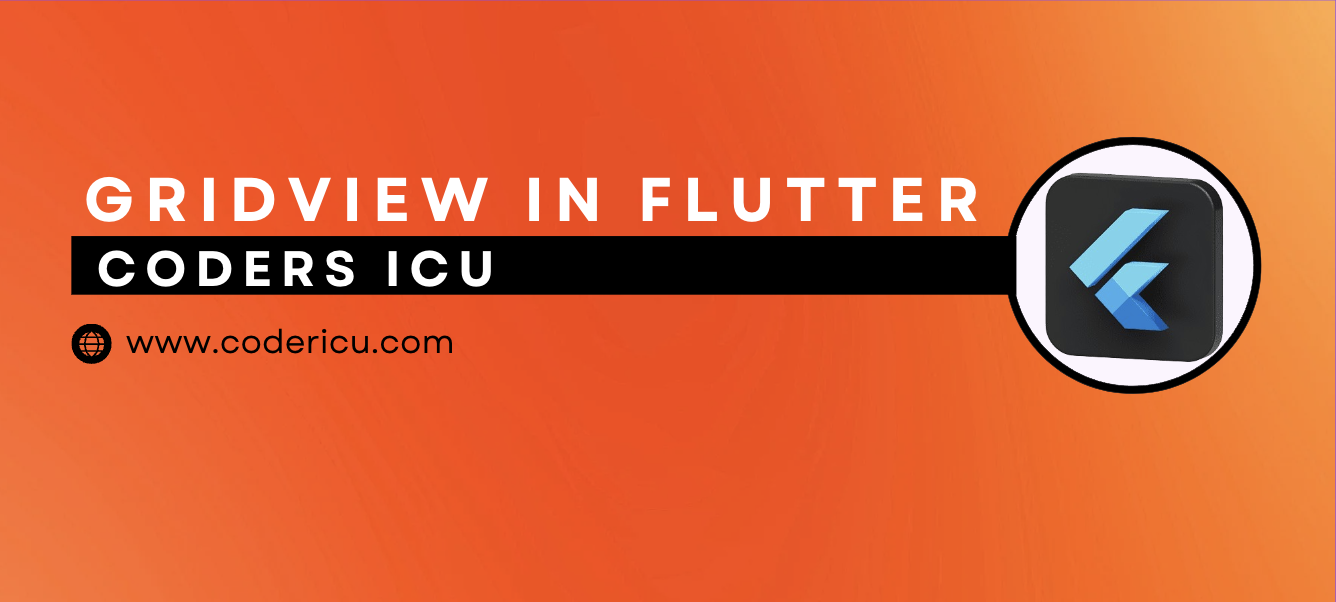
A “GridView in Flutter” is a widget in Flutter that displays a scrolling grid of widgets (for more details). It is a powerful and flexible widget that can be used to create a wide range of grid-based layouts.
Properties
Here are some of the key properties of the “GridView” widget:
- gridDelegate: Defines the layout and structure of the grid. There are several types of grid delegates available, including:
- SliverGridDelegateWithFixedCrossAxisCount: Creates a grid with a fixed number of columns.
- SliverGridDelegateWithMaxCrossAxisExtent: Creates a grid with a maximum cross-axis extent.
- SliverGridDelegateWithFlexibleCrossAxisCount: Creates a grid with a flexible number of columns.
- children: A list of widgets to be displayed in the grid.
- crossAxisCount: The number of columns in the grid.
- mainAxisSpacing: The spacing between items in the main axis (vertical spacing).
- crossAxisSpacing: The spacing between items in the cross axis (horizontal spacing).
- childAspectRatio: The aspect ratio of each child widget.
- padding: The padding around the grid.
Types
There are two types of “GridView” widgets:
- “GridView“: A scrolling grid of widgets.
- GridView.builder: A scrolling grid of widgets that uses a builder function to create the widgets on demand.
Use
“GridViews” are commonly used to display a collection of items in a grid layout, such as:
- Image galleries
- Product lists
- News feeds
- Calendars
“Gridview” Simple Example
import 'package:flutter/material.dart';
class SimpleGridView extends StatelessWidget {
@override
Widget build(BuildContext context) {
return GridView.count(
// Number of columns in the grid
crossAxisCount: 2,
// Spacing between items
crossAxisSpacing: 10,
mainAxisSpacing: 10,
// Create a fixed list of items for demonstration
children: List.generate(20, (index) {
return Container(
decoration: BoxDecoration(
color: Colors.primaries[index % Colors.primaries.length],
borderRadius: BorderRadius.circular(15),
boxShadow: [
BoxShadow(
color: Colors.black26,
blurRadius: 8,
offset: Offset(4, 4),
),
],
),
child: Center(
child: Text(
'Item $index',
style: TextStyle(
color: Colors.white,
fontWeight: FontWeight.bold,
fontSize: 16,
),
),
),
);
}),
);
}
}
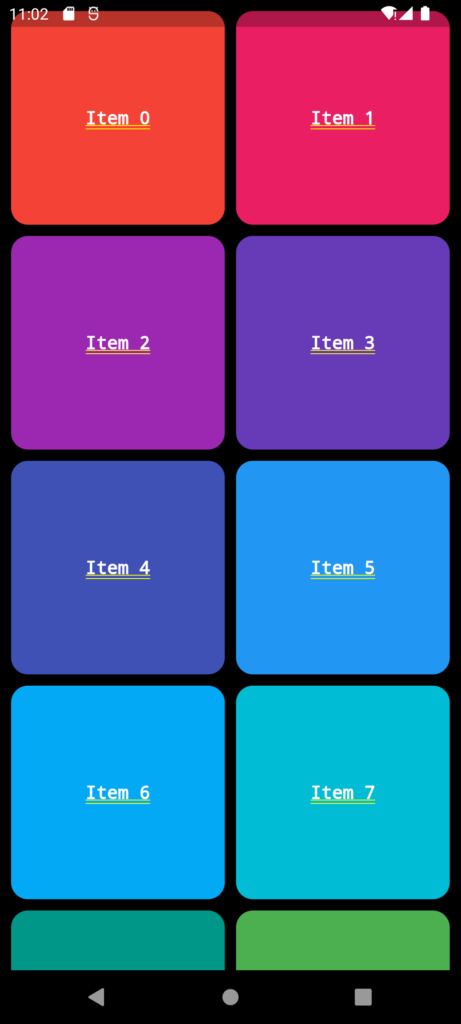
2 thoughts on “GridView in Flutter”