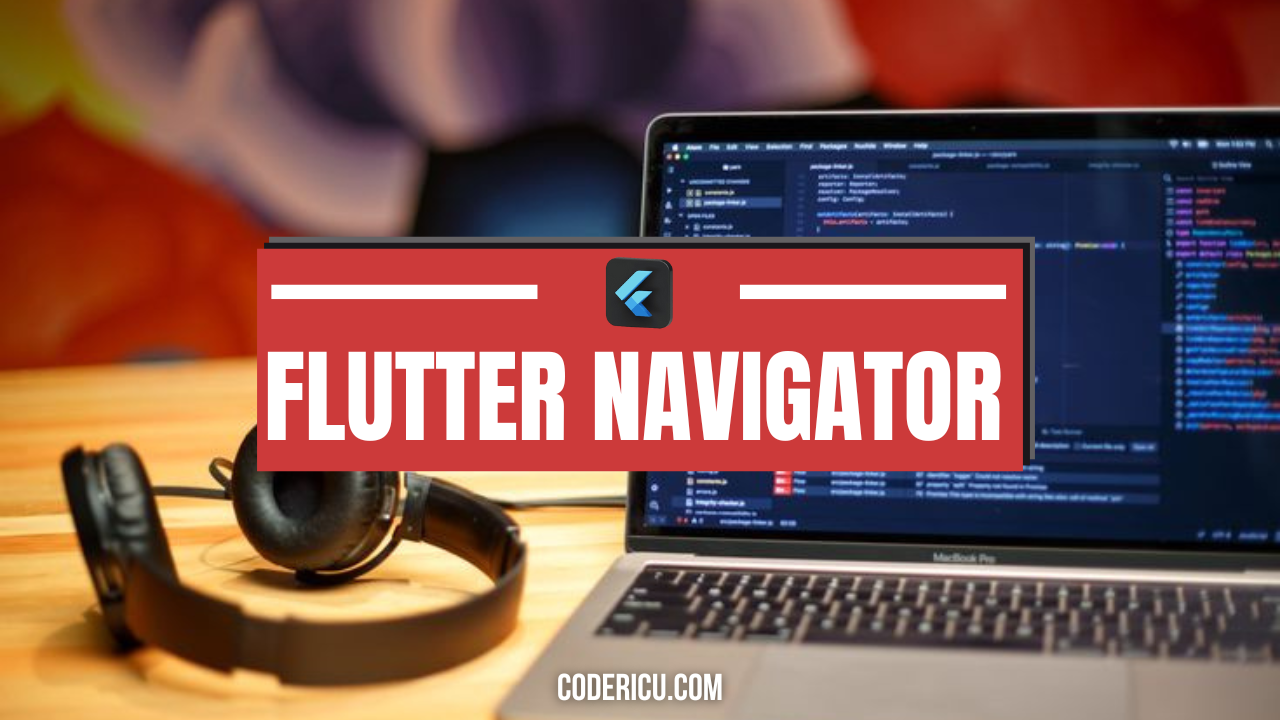
The Navigator widget in Flutter is a built-in widget that manages a stack of routes, allowing for navigation between different screens or pages in an app. It is a fundamental component for building multi-screen apps.
Key Features:
- Route Management: The Navigator widget manages a stack of routes, where each route represents a screen or page in the app.
- Push and Pop: Routes can be pushed (added) or popped (removed) from the stack, allowing for navigation between screens.
- Stack-based Navigation: The Navigator uses a stack data structure to manage routes, making it easy to navigate back and forth between screens.
Commonly Used Methods:
Navigator.push():
This method is used to navigate to a new screen by pushing a new route onto the stack. The new screen is added on top of the current screen, and users can return to the previous screen by popping the top route (e.g., pressing the back button).
Navigator.push(
context,
MaterialPageRoute(builder: (context) => NewScreen()),
);
Navigator.pop():
This method is used to return to the previous screen by removing the current route from the stack. It is commonly used when a user has completed a task on a screen and wants to go back to the previous screen.
Navigator.pop(context);
Navigator.pushReplacement():
This method pushes a new route onto the stack and removes the previous route, replacing it. It is useful when you want to navigate to a new screen and do not want the user to be able to return to the previous screen using the back button.
Navigator.pushReplacement(
context,
MaterialPageRoute(builder: (context) => NewScreen()),
);
Navigator Properties:
- onGenerateRoute: Callback to generate a route based on the given RouteSettings.
- onUnknownRoute: Callback to handle unknown routes.
- initialRoute: The initial route to display.
- routes: A map of route names to route builders.
Example:
import 'package:flutter/material.dart';
// HomeScreen is a StatelessWidget that represents the first screen of the app.
class HomeScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Home Screen'), // Title displayed in the AppBar
),
body: Center(
child: ElevatedButton(
// This button navigates to the SecondScreen when pressed
onPressed: () {
// Navigate to SecondScreen using Navigator.push
Navigator.push(
context,
MaterialPageRoute(builder: (context) => SecondScreen()),
);
},
child: Text('Go to Second Screen'), // Button text
),
),
);
}
}
// SecondScreen is a StatelessWidget that represents the second screen of the app.
class SecondScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Second Screen'), // Title displayed in the AppBar
),
body: Center(
child: ElevatedButton(
// This button navigates back to the previous screen (HomeScreen) when pressed
onPressed: () {
// Return to the previous screen using Navigator.pop
Navigator.pop(context);
},
child: Text('Back to Home Screen'), // Button text
),
),
);
}
}
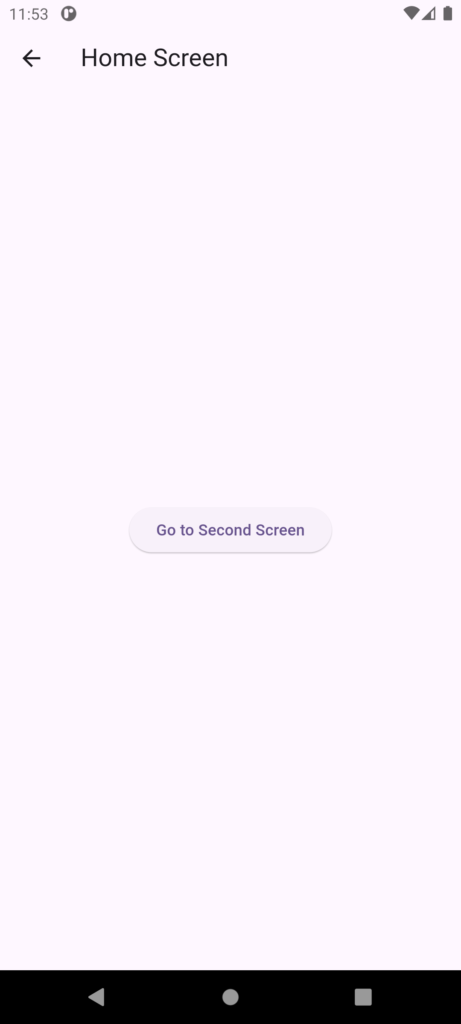
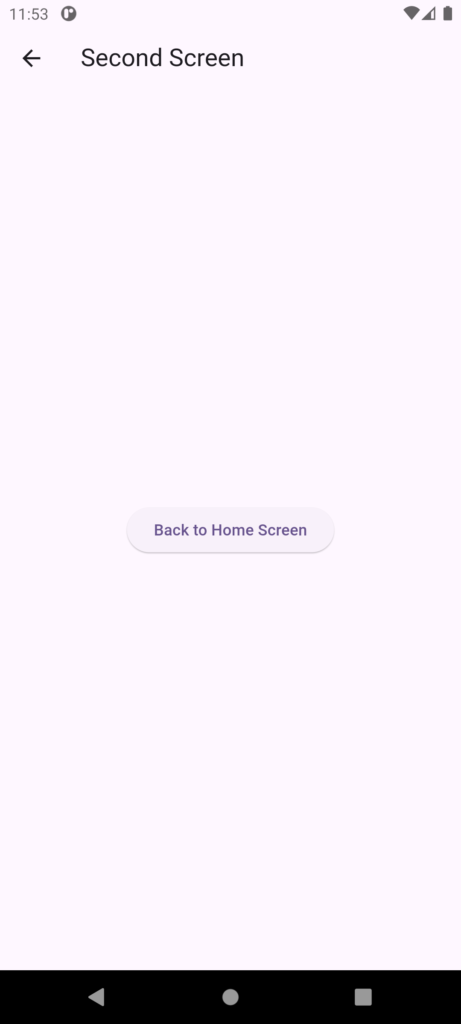