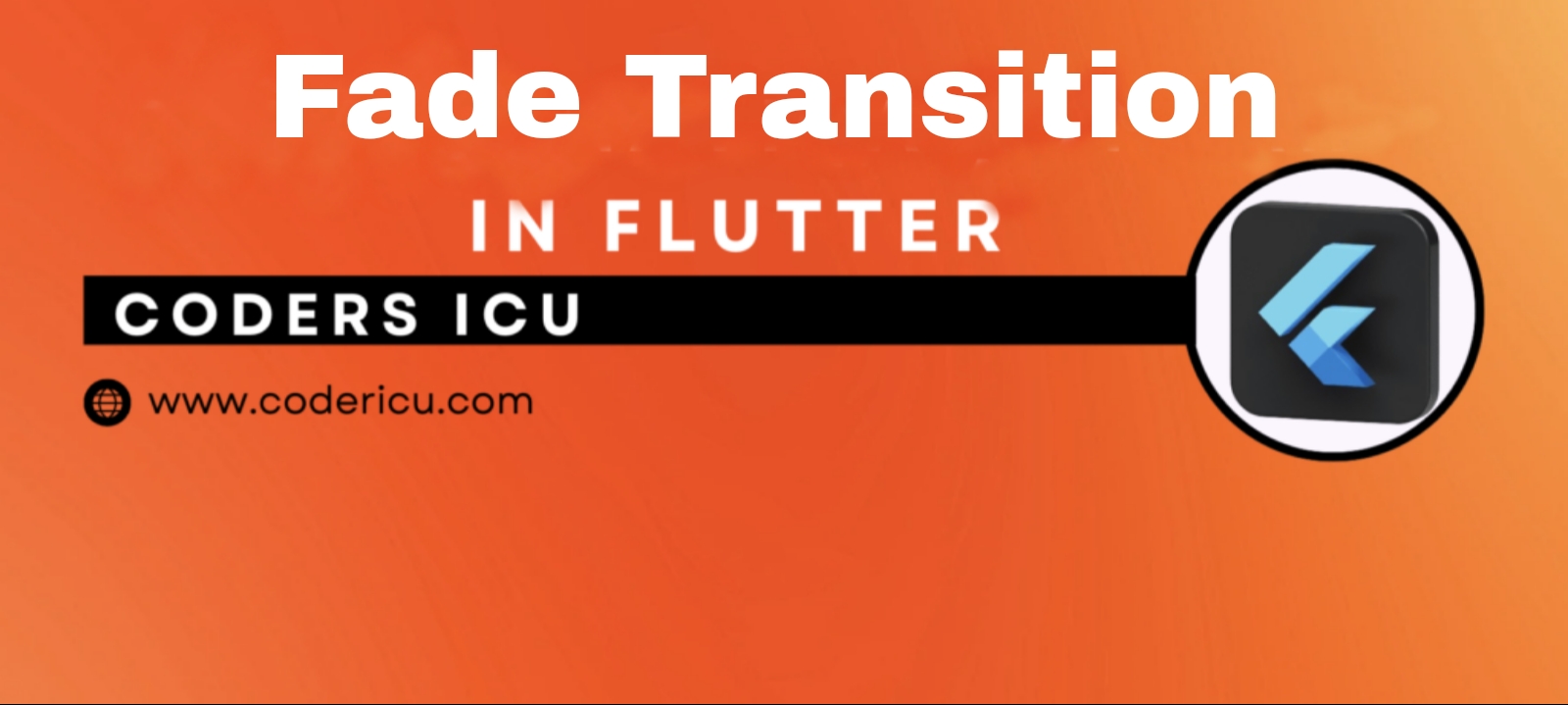
{"remix_data":[],"remix_entry_point":"challenges","source_tags":["local"],"origin":"unknown","total_draw_time":0,"total_draw_actions":0,"layers_used":0,"brushes_used":0,"photos_added":0,"total_editor_actions":{},"tools_used":{"clone":1},"is_sticker":false,"edited_since_last_sticker_save":true,"containsFTESticker":false}
A Fade Transition in Flutter is a type of animation that allows widgets to gradually appear or disappear from the screen by adjusting their opacity. This transition effect enhances the user experience by providing a smooth and visually appealing way to add or remove widgets.
1. Fade In
- The widget starts with an opacity of 0 (fully transparent) and gradually increases to 1 (fully opaque).
2. Fade Out
- The widget starts with an opacity of 1 (fully opaque) and gradually decreases to 0 (fully transparent).
Implementing Fade Transition in Flutter
To implement a Fade Transition in Flutter, you can use the FadeTransition
widget. This widget takes a child widget and an animation object as input.
FadeTransition Widget
Dart
FadeTransition({
Key? key,
required Animation<double> opacity,
Widget? child,
})
//key: An optional key for the widget.
//opacity: The animation object that controls the opacity of the child widget.
//child: The widget to be faded in or out.
Example:
import 'package:flutter/material.dart';
// Stateful widget for the FadeTransition demo
class FadetransitionDemo extends StatefulWidget {
// Constructor with a required decoration parameter
const FadetransitionDemo({Key? key, required BoxDecoration decoration}) : super(key: key);
@override
_FadetransitionDemoState createState() => _FadetransitionDemoState();
}
class _FadetransitionDemoState extends State<FadetransitionDemo> with SingleTickerProviderStateMixin {
// AnimationController manages the animation's timing
late AnimationController _controller;
// Animation object that will control the opacity
late Animation<double> _animation;
// Called once when the widget is inserted into the widget tree
@override
void initState() {
super.initState();
// Initialize the AnimationController with a duration of 2 seconds
// `vsync` ensures the animation is synced with the screen's refresh rate
_controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this, // Uses the State object for vsync
)..repeat(reverse: true); // Repeat the animation back and forth
// Tween is used to define the animation's range (from 0 to 1)
_animation = Tween<double>(begin: 0, end: 1).animate(_controller);
}
// Dispose is called when the widget is removed from the tree
// This is important to free up resources used by the AnimationController
@override
void dispose() {
_controller.dispose(); // Stop the animation controller
super.dispose();
}
@override
Widget build(BuildContext context) {
// SafeArea ensures that content is placed within the visible screen area
return SafeArea(
child: Scaffold(
// AppBar provides the top navigation bar with a title
appBar: AppBar(
title: Text("FadeTransition Example"),
flexibleSpace: Container(
// Adds a gradient background to the AppBar
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.deepPurple, Colors.orangeAccent], // Gradient colors
begin: Alignment.topLeft, // Gradient starts at the top left
end: Alignment.topRight, // Gradient ends at the top right
),
),
),
),
// The main content of the screen is centered
body: Center(
// FadeTransition applies the opacity animation to its child
child: FadeTransition(
opacity: _animation, // The animation controls the opacity
child: Container(
width: 100, // Width of the container (100 units)
height: 100, // Height of the container (100 units)
color: Colors.blue, // The color of the container (solid blue)
),
),
),
),
);
}
}