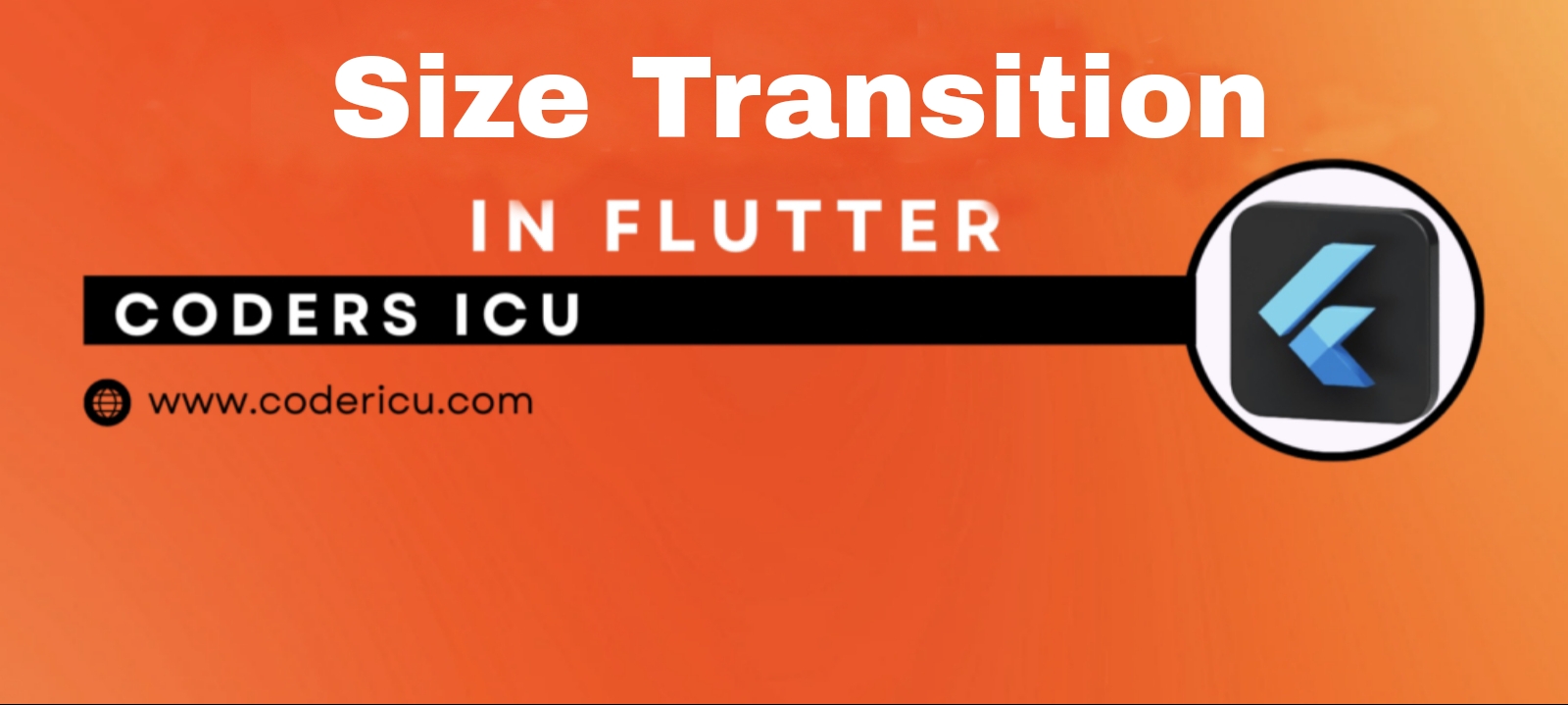
{"remix_data":[],"remix_entry_point":"challenges","source_tags":["local"],"origin":"unknown","total_draw_time":0,"total_draw_actions":0,"layers_used":0,"brushes_used":0,"photos_added":0,"total_editor_actions":{},"tools_used":{},"is_sticker":false,"edited_since_last_sticker_save":false,"containsFTESticker":false}
In Flutter, SizeTransition is a widget that animates the size change of its child widget. It is used to create a size transition effect, where the child widget’s size changes smoothly from its initial size to its final size.
Construction
SizeTransition(
{Key? key,
Axis axis = Axis.vertical,
Widget? child,
double sizeFactor = 1.0,
Curve curve = Curves.linear,
required Animation<double> animation})
Parameters
axis
: The axis along which to animate the size change. Defaults toAxis.vertical
.child
: The child widget.sizeFactor
: Controls the size of the child widget relative to its original size. Defaults to 1.0.curve
: The curve to apply to the animation. Defaults toCurves.linear
.animation
: The animation that drives the size change.
Example:
import 'package:flutter/material.dart';
/// This class represents a screen with a size transition animation.
/// The `SizeTransitionScreen` widget is a StatefulWidget, meaning it can hold mutable state
/// that is managed by its corresponding state class (_SizeTransitionScreenState).
class SizeTransitionScreen extends StatefulWidget {
// Constructor for the SizeTransitionScreen, which accepts a BoxDecoration for customization (though not used here).
const SizeTransitionScreen({Key? key, required BoxDecoration decoration}) : super(key: key);
@override
_SizeTransitionScreenState createState() => _SizeTransitionScreenState();
}
/// The state class manages the animation lifecycle and builds the UI.
class _SizeTransitionScreenState extends State<SizeTransitionScreen> with SingleTickerProviderStateMixin {
late AnimationController _controller; // Controls the animation and its timing.
late Animation<double> _animation; // Defines the animation that will be driven by the controller.
/// Initializes the animation controller and the animation when the widget is inserted into the widget tree.
@override
void initState() {
super.initState();
// Create an AnimationController with a duration of 2 seconds.
// `vsync: this` ensures that the animation is only active when the screen is visible, saving resources.
_controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
)
// The animation will repeat indefinitely, and reverse itself after each cycle.
..repeat(reverse: true);
// Define the animation using a Tween (interpolates values between 0.0 and 1.0).
_animation = Tween<double>(begin: 0.0, end: 1.0).animate(_controller);
}
/// Disposes of the animation controller when the widget is removed from the widget tree.
/// This prevents memory leaks by properly cleaning up resources.
@override
void dispose() {
_controller.dispose(); // Always dispose of controllers when no longer needed.
super.dispose();
}
/// Builds the widget tree for this screen, which includes the AppBar and a SizeTransition in the body.
@override
Widget build(BuildContext context) {
return Scaffold(
// AppBar to display the title of the screen with a custom background gradient.
appBar: AppBar(
title: Text("SizeTransition Example"),
flexibleSpace: Container(
decoration: BoxDecoration(
gradient: LinearGradient(
// A gradient transitioning from deep purple to orange accent.
colors: [Colors.deepPurple, Colors.orangeAccent],
begin: Alignment.topLeft, // Gradient begins at the top-left corner.
end: Alignment.topRight, // Gradient ends at the top-right corner.
),
),
),
),
// The body of the screen contains a Center widget for centering content on the screen.
body: Center(
// The SizeTransition widget animates its child's size.
// It animates the `sizeFactor` which ranges from 0.0 (collapsed) to 1.0 (full size) based on the _animation.
child: SizeTransition(
sizeFactor: _animation, // Uses the animation as the size factor (0.0 to 1.0).
axis: Axis.horizontal, // Animates the size horizontally.
axisAlignment: -1.0, // Aligns the start of the animation with the left side (-1.0 is fully aligned left).
// The animated child widget is a blue container with a fixed width and height.
child: Container(
width: 200, // Fixed width of the container.
height: 100, // Fixed height of the container.
color: Colors.blue, // The container is filled with a solid blue color.
),
),
),
);
}
}