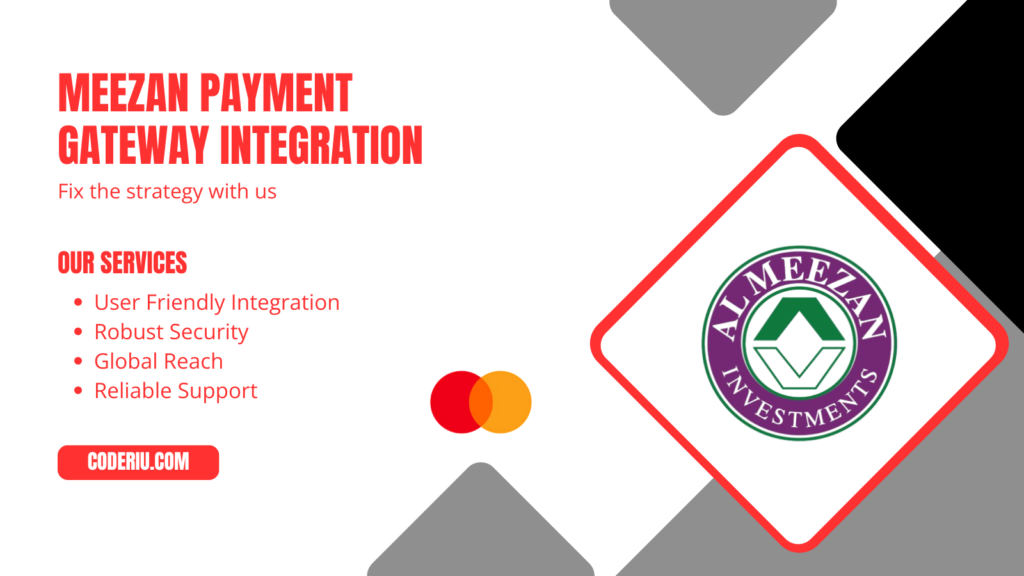
In today’s fast-paced digital economy, integrating a secure and efficient payment gateway is essential for eCommerce businesses. Meezan Payment Gateway is a trusted solution for businesses in Pakistan, providing seamless online transactions with Shariah-compliant financial services. In this guide, we will explore how to integrate Meezan Bank’s payment gateway into your Laravel application.
Why Choose Meezan Payment Gateway?
- Shariah-compliant: Meezan Bank ensures all transactions adhere to Islamic banking principles.
- Secure Transactions: Advanced encryption technology keeps payments safe.
- Multiple Payment Options: Accept payments via debit/credit cards, Meezan’s digital banking, and more.
- Easy Integration with Laravel: Laravel’s robust framework makes API integration smooth.
Prerequisites for Integration
Before integrating Meezan Payment Gateway, ensure you have the following:
- A Meezan Bank merchant account
- API credentials from Meezan Bank
- A Laravel application (Laravel 9 or 10 recommended)
- Basic knowledge of REST APIs and Laravel development
Step-by-Step Guide to Meezan Payment Gateway Integration in Laravel
Step 1: Obtain API Credentials
First, register as a merchant with Meezan Bank and obtain the API key, secret key, and endpoint URLs.
Step 2: Install Required Laravel Packages
Ensure your Laravel project is set up and installed guzzlehttp/guzzle
to handle API requests:
composer require guzzlehttp/guzzle
Step 3: Configure API Credentials
Add your Meezan Bank API credentials to the .env
file:
MEEZAN_API_KEY=your_api_key_here
MEEZAN_SECRET_KEY=your_secret_key_here
MEEZAN_ENDPOINT=https://sandbox.meezanbank.com/api/payment
Then, update config/services.php
:
return [
'meezan' => [
'key' => env('MEEZAN_API_KEY'),
'secret' => env('MEEZAN_SECRET_KEY'),
'endpoint' => env('MEEZAN_ENDPOINT'),
],
];
Step 4: Create a Payment Controller
Generate a Laravel controller to handle payment requests:
php artisan make:controller PaymentController
Inside,PaymentController.php
add the following:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use GuzzleHttp\Client;
class PaymentController extends Controller
{
public function initiatePayment(Request $request)
{
$client = new Client();
$response = $client->post(config('services.meezan.endpoint'), [
'headers' => [
'Authorization' => 'Bearer ' . config('services.meezan.key'),
'Content-Type' => 'application/json'
],
'json' => [
'amount' => $request->amount,
'currency' => 'PKR',
'callback_url' => route('payment.callback'),
'order_id' => uniqid(),
]
]);
$data = json_decode($response->getBody(), true);
return redirect()->to($data['payment_url']);
}
}
Step 5: Define Routes in Laravel
Open routes/web.php
and add:
use App\Http\Controllers\PaymentController;
Route::post('/pay', [PaymentController::class, 'initiatePayment'])->name('payment.initiate');
Route::get('/payment/callback', function () {
return 'Payment Successful!';
})->name('payment.callback');
Step 6: Create a Payment Form
In your Blade template, create a simple payment form:
<form action="{{ route('payment.initiate') }}" method="POST">
@csrf
<label>Enter Amount:</label>
<input type="number" name="amount" required>
<button type="submit">Pay Now</button>
</form>
Step 7: Handle Payment Response
Once the payment is processed, Meezan Bank will redirect the user to the callback URL with a response status. You can handle success/failure messages accordingly.
Conclusion
Meezan Payment Gateway integration with Laravel is a seamless process that enhances the security and efficiency of your eCommerce platform. By following this guide, you can set up a Shariah-compliant, reliable, and scalable payment solution for your online store.
Need expert help with Laravel payment gateway integration? I offer seamless integrations for eCommerce systems. Contact me now! 🚀