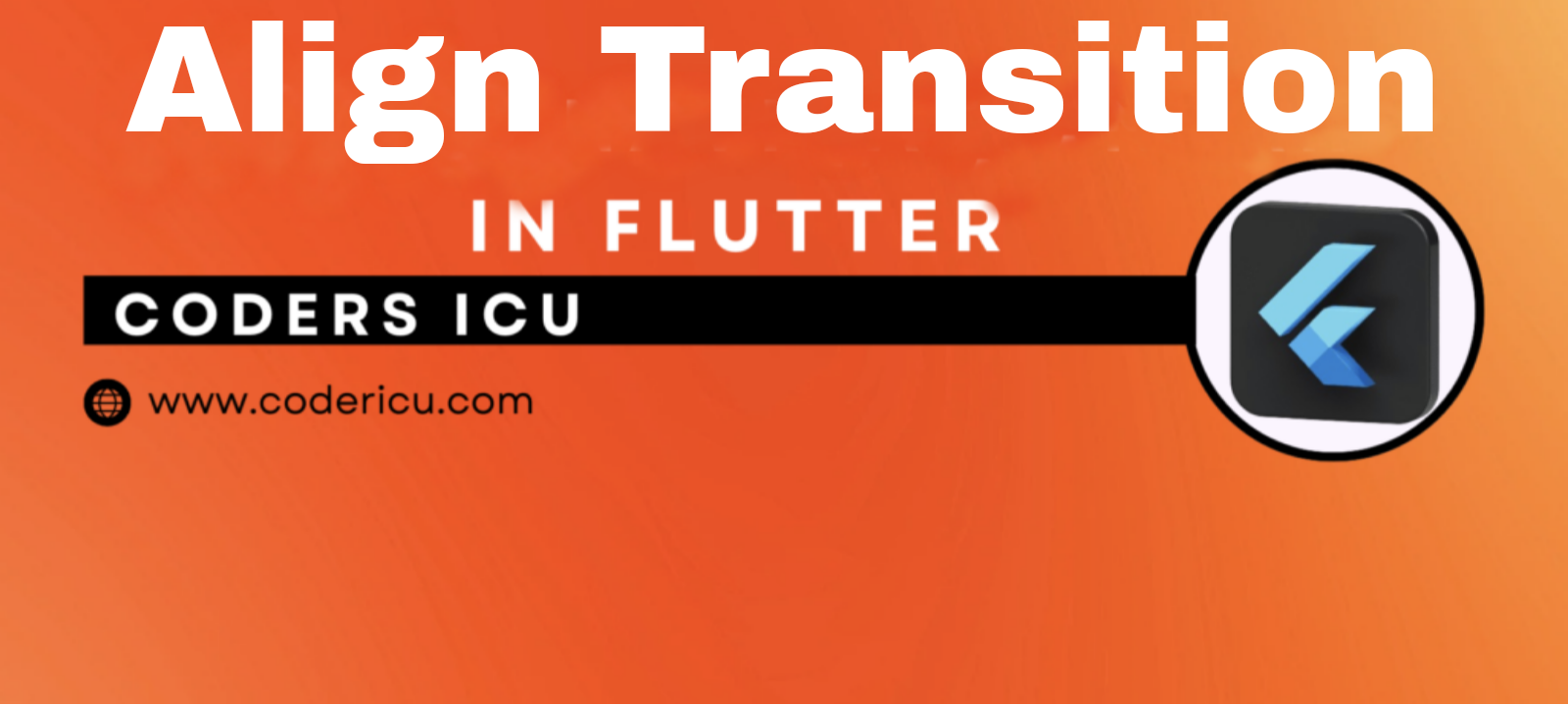
In Flutter, the AlignTransition widget is used to animate the alignment of a widget. This widget smoothly transitions the alignment of a child widget from one point to another over a specific duration. It’s ideal for creating movement animations, where a widget needs to transition between different locations within its parent.
Key Properties of AlignTransition:
- alignment: This is an
Animation<AlignmentGeometry>
that defines the movement. It controls the start and end positions of the widget, such as from top-left to bottom-right. - child: The widget you want to animate, typically placed inside the
AlignTransition
. Thechild
will follow the animated alignment.
How It Works:
- AlignTransition animates the alignment property of a widget over time. You define the start (
begin
) and end (end
) alignment points, and Flutter smoothly transitions the widget between these points. - The alignment points are controlled using the
Tween<AlignmentGeometry>
, which interpolates the alignment from one position to another. - The animation can be repeated, reversed, or controlled with other typical animation properties.
Use Cases:
- Animating a widget’s movement across the screen.
- Creating smooth UI transitions where elements shift positions dynamically.
- Moving elements in response to user interactions or timed events.
Advantages:
- Provides smooth movement animations.
- Easily combined with other animation widgets like
ScaleTransition
orFadeTransition
for more complex effects. - Works well for dynamic, responsive UI designs.
Example
import 'package:flutter/material.dart';
class AlignTransitionScreen extends StatefulWidget {
const AlignTransitionScreen({Key? key, required BoxDecoration decoration}) : super(key: key);
@override
_AlignTransitionScreenState createState() => _AlignTransitionScreenState();
}
class _AlignTransitionScreenState extends State<AlignTransitionScreen> with SingleTickerProviderStateMixin {
late AnimationController _controller;
late Animation<Alignment> _alignment;
@override
void initState() {
super.initState();
_controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
)..repeat(reverse: true);
_alignment = Tween<Alignment>(
begin: Alignment.topLeft,
end: Alignment.bottomRight,
).animate(CurvedAnimation(parent: _controller, curve: Curves.easeInOut));
}
@override
void dispose() {
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("AlignTransition Example"),
flexibleSpace: Container(
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.deepPurple, Colors.orangeAccent],
begin: Alignment.topLeft,
end: Alignment.topRight,
),
),
),
),
body: Center(
child: AlignTransition(
alignment: _alignment,
child: Container(
width: 100,
height: 100,
color: Colors.blue,
),
),
),
);
}
}