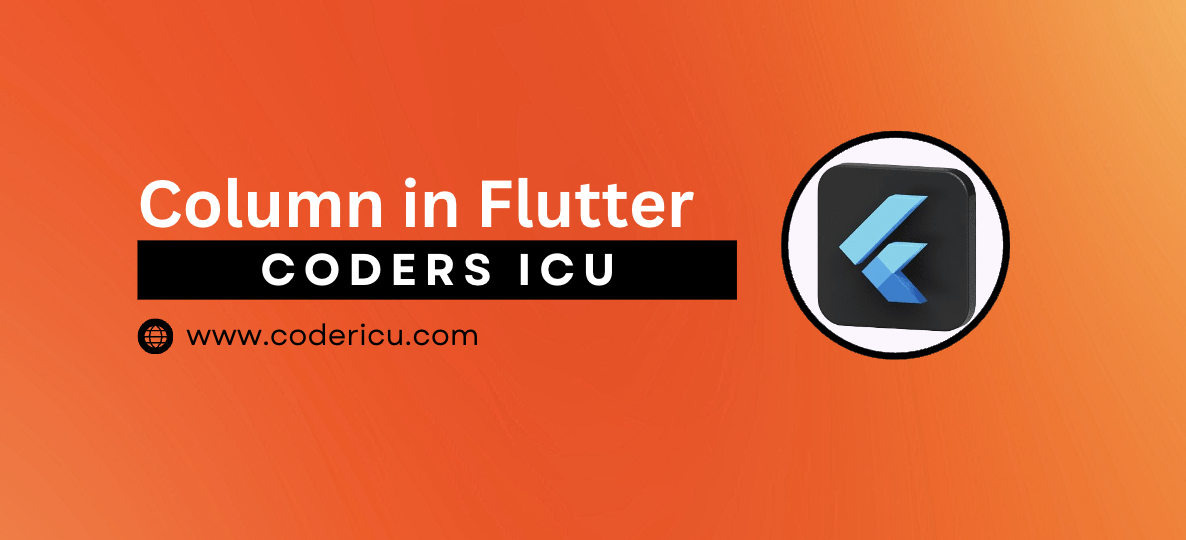
In Flutter, a Column is a widget that displays its children in a vertical array. It is a built-in widget that allows you to create a column of widgets, such as text, images, or other widgets.
Properties
Here are the properties of a Column
widget:
- children: A list of widgets to be displayed in the column
- mainAxisAlignment: Specifies how to align the children vertically (e.g., start, center, end, space-between)
- crossAxisAlignment: Specifies how to align the children horizontally (e.g., start, center, end, stretch)
- mainAxisSize: Specifies the height of the column (e.g., max, min)
Example:
Column(
children: [
Text('Hello'),
Text('World'),
Icon(Icons.heart),
],
)
Use cases:
- Create a vertical array of widgets
- Display a list of items vertically
- Create a custom menu or navigation bar
- Display a vertical progress bar
Note: Column is similar to Row, but it displays its children vertically instead of horizontally.
Code:
import 'package:flutter/material.dart';
class Columcode extends StatefulWidget {
const Columcode({Key? key}) : super(key: key);
@override
State<Columcode> createState() => _ColumcodeState();
}
class _ColumcodeState extends State<Columcode> {
@override
Widget build(BuildContext context) {
return Scaffold(
// Scaffold provides the basic layout structure for the app
appBar: AppBar(
// AppBar widget is used for displaying the top app bar
title: Text(
"Coloum Code",
style: TextStyle(
fontWeight: FontWeight.bold, // Makes the text bold
color: Colors.white, // Sets the text color to white
),
),
flexibleSpace: Container(
// Container used to customize the app bar's background
decoration: BoxDecoration(
// BoxDecoration allows styling of the container
borderRadius: BorderRadius.circular(10), // Rounded corners with a radius of 10
gradient: LinearGradient(
// LinearGradient creates a gradient effect for the background
colors: [Colors.deepPurple, Colors.orangeAccent], // Colors of the gradient
begin: Alignment.topLeft, // Gradient starts from the top-left
end: Alignment.topRight, // Gradient ends at the top-right
),
),
),
),
body: Column(
// Column arranges its children vertically
crossAxisAlignment: CrossAxisAlignment.center, // Aligns children horizontally in the center of the column
mainAxisAlignment: MainAxisAlignment.center, // Aligns children vertically in the center of the column
children: [
// You can add multiple children here that will be arranged vertically
Container(
width: 100,
height: 100,
color: Colors.red,
child: Center(
child: Text(
'Red',
style: TextStyle(color: Colors.white), // Sets the text color to white
),
),
),
SizedBox(height: 10), // Adds vertical space of 10 pixels between children
Container(
width: 100,
height: 100,
color: Colors.green,
child: Center(
child: Text(
'Green',
style: TextStyle(color: Colors.white), // Sets the text color to white
),
),
),
SizedBox(height: 10), // Adds vertical space of 10 pixels between children
Container(
width: 100,
height: 100,
color: Colors.blue,
child: Center(
child: Text(
'Blue',
style: TextStyle(color: Colors.white), // Sets the text color to white
),
),
),
],
),
);
}
}
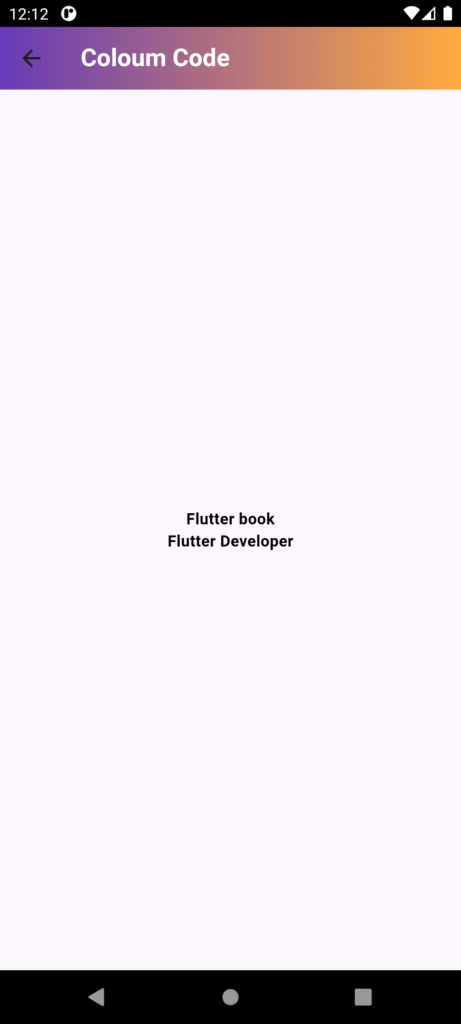