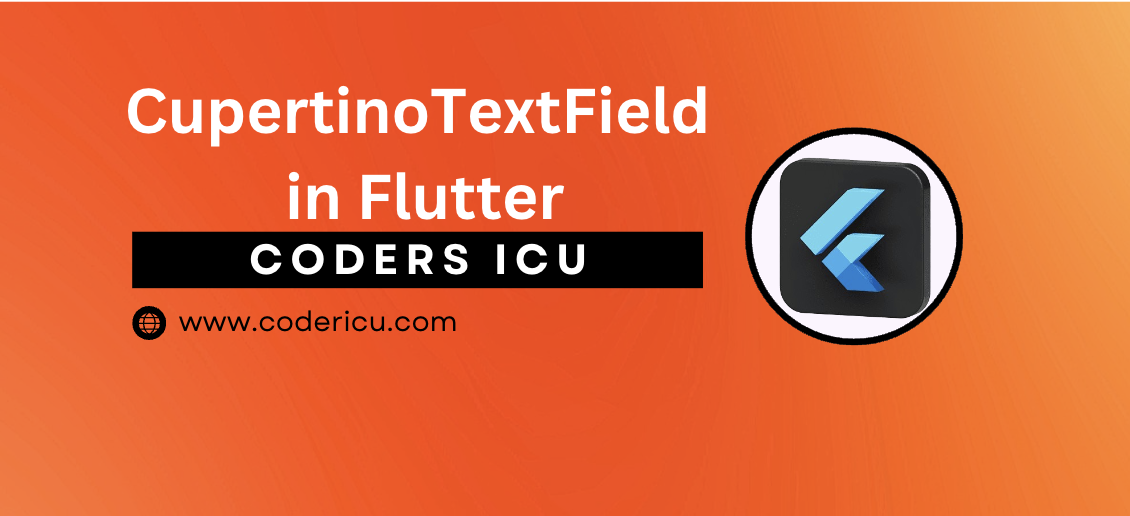
CupertinoTextField is a widget in Flutter that provides a text field with a Cupertino (iOS-style) design. It is a component of the Cupertino library, which enables you to design Flutter app interfaces that resemble those of iOS.
CupertinoTextField is Flutterโs set of widgets that look and feel like native iOS components.
A text field with a clear button, a rounded rectangular border, and placeholder text gives it a Cupertino-esque appearance. It looks like an iOS widget, but it’s comparable to the TextField widget.
To use CupertinoTextField, you need to import the Cupertino library in your Dart file:
Properties:
- placeholder: The placeholder text to display when the field is empty.
- prefix: The prefix icon or widget to display before the text.
- suffix: The suffix icon or widget to display after the text.
- keyboardType: The type of keyboard to display (e.g., text, email, phone).
- onSubmitted: The callback function when the user submits the text.
key features of CupertinoTextField include:
- Rounded rectangle border
- Clear button to delete text
- Placeholder text
- Support for prefix and suffix icons
- Support for error text display
CupertinoTextField(
placeholder: 'Enter your name',
prefix: Icon(CupertinoIcons.person),
suffix: Icon(CupertinoIcons.clear),
onSubmitted: (value) {
print('Submitted: $value');
},
)
//To use CupertinoTextField, you need to import the cupertino library in your Dart file:
import 'package:flutter/cupertino.dart';
mport 'package:flutter/cupertino.dart'; //cupertino Library
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return CupertinoApp(
home: CupertinoPageScaffold(
navigationBar: CupertinoNavigationBar(
middle: Text('Cupertino Text Field Example'),
),
child: Center(
child: Padding(
padding: const EdgeInsets.all(16.0),
child: CupertinoTextField(
placeholder: 'Enter text here',
padding: EdgeInsets.symmetric(horizontal: 16.0, vertical: 12.0),
border: CupertinoBorder(
color: CupertinoColors.systemGrey,
width: 1.0,
),
textInputAction: TextInputAction.done,
keyboardType: TextInputType.text,
),
),
),
),
);
}
}
1 thought on “CupertinoTextField in Flutter”