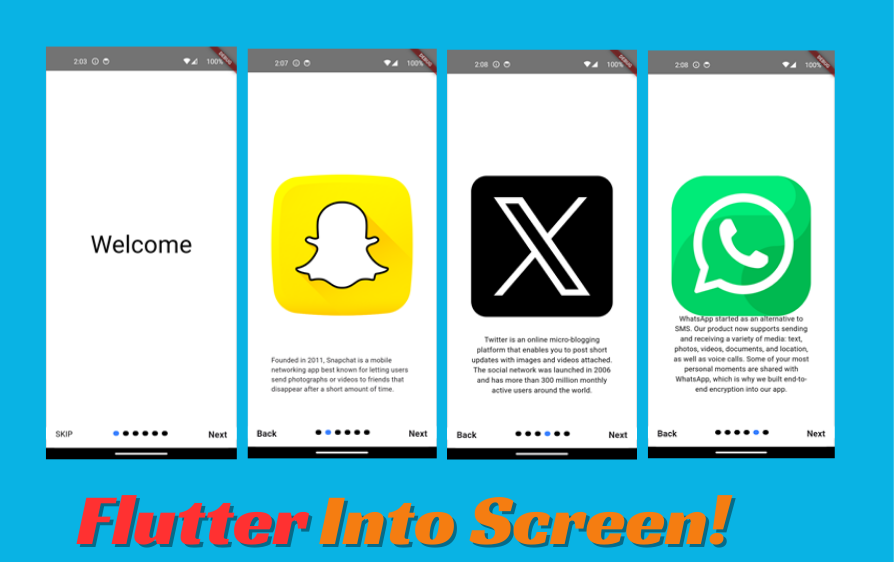
how to use Intro Screen in Flutter?
create flutter project in your cmd/terminal by using following command
flutter create intro_screen
for better styling we will be using ‘Get Widget’ so import getwidget dependency in your pubspec.yaml file. you can use any compatible version from pubdev.
getwidget: ^4.0.0
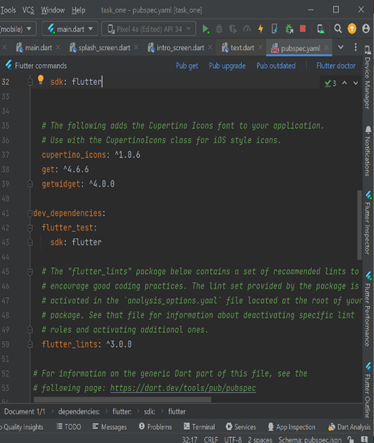
use following command in cmd/terminal to get dependency
flutter pub get
now replace your main.dart
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:intro_screen/IntroScreen.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return GetMaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const IntroScreen(),
);
}
}
create new dart file in lib folder with name of intro_screen.dart
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/painting.dart';
import 'package:getwidget/colors/gf_color.dart';
import 'package:getwidget/components/image/gf_image_overlay.dart';
import 'package:getwidget/components/intro_screen/gf_intro_screen.dart';
import 'package:getwidget/components/intro_screen/gf_intro_screen_bottom_navigation_bar.dart';
class IntroScreen extends StatefulWidget {
const IntroScreen({super.key});
@override
State<IntroScreen> createState() => _IntroScreenState();
}
class _IntroScreenState extends State<IntroScreen> {
late PageController _pageController;
late int initialPage;
@override
void initState() {
_pageController = PageController(initialPage: 0);
initialPage = _pageController.initialPage;
super.initState();
}
void _redirectToHomePage(BuildContext context) {
// Navigate to the home page
Navigator.pushReplacementNamed(context, '/home');
// you can use MaterialPageRout() as well
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.white60,
body: SafeArea(
child: GFIntroScreen(
navigationBarColor: Colors.black26,
navigationBarShape: const RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(20)),
),
pageController: _pageController,
currentIndex: initialPage,
pageCount: 6,
color: Colors.white,
introScreenBottomNavigationBar: GFIntroScreenBottomNavigationBar(
pageController: _pageController,
currentIndex: initialPage,
pageCount: 6,
onForwardButtonTap: () {
// Check if this is the last page
if (_pageController.page == 5) {
// Redirect to the home page
_redirectToHomePage(context);
} else {
// Move to the next page
_pageController.nextPage(
duration: const Duration(milliseconds: 300),
curve: Curves.linear,
);
}
},
navigationBarShape: const OutlineInputBorder(
borderSide: BorderSide.none,
borderRadius: BorderRadius.all(Radius.circular(20)),
gapPadding: 20,
),
dotMargin: const EdgeInsets.all(4),
forwardButtonText: 'Next',
backButtonText: 'Back',
backButtonTextStyle: const TextStyle(
fontWeight: FontWeight.bold,
fontSize: 18,
),
forwardButtonTextStyle: const TextStyle(
fontWeight: FontWeight.bold,
fontSize: 18,
),
dotWidth: 12,
dotHeight: 10,
navigationBarHeight: 60,
navigationBarMargin: const EdgeInsets.all(05),
navigationBarPadding: const EdgeInsets.all(15),
navigationBarWidth: 500,
navigationBarColor: Colors.white,
showDivider: false,
inactiveColor: Colors.black,
activeColor: GFColors.PRIMARY,
),
slides: [
const Center(
child: Text(
'Welcome',
style: TextStyle(fontSize: 50, color: Colors.black),
),
),
Padding(
padding: const EdgeInsets.all(50.0),
child: Container(
width: MediaQuery.of(context).size.width,
height: MediaQuery.of(context).size.height,
decoration: BoxDecoration(
borderRadius: const BorderRadius.only(
topLeft: Radius.circular(40),
topRight: Radius.circular(40),
),
image: DecorationImage(
image: const AssetImage('assets/images/snapchat.png'),
fit: BoxFit.fitWidth,
colorFilter: ColorFilter.mode(
Colors.black.withOpacity(0),
BlendMode.darken,
),
),
),
child: const Align(
alignment: Alignment.bottomCenter,
child: Text(
'Founded in 2011, Snapchat is a mobile networking app best known for letting users send photographs or videos to friends that disappear after a short amount of time.',
style: TextStyle(),
),
),
),
),
Padding(
padding: const EdgeInsets.all(50.0),
child: GFImageOverlay(
height: MediaQuery.of(context).size.width,
colorFilter: ColorFilter.mode(
Colors.black.withOpacity(0),
BlendMode.darken,
),
boxFit: BoxFit.fitWidth,
image: const AssetImage('assets/images/telegram.png'),
child: const Align(
alignment: Alignment.bottomCenter,
child: Text(
'Telegram is a competitor of the popular messaging service WhatsApp, which has faced controversy for sharing user data with its parent company, Facebook. ',
selectionColor: Colors.black,
textAlign: TextAlign.center,
semanticsLabel: 'pakistan',
style: TextStyle(fontSize: 15, color: Colors.black),
),
),
),
),
Padding(
padding: const EdgeInsets.all(50.0),
child: GFImageOverlay(
colorFilter: ColorFilter.mode(
Colors.black.withOpacity(0),
BlendMode.darken,
),
boxFit: BoxFit.fitWidth,
image: const AssetImage('assets/images/twitter.png'),
child: const Align(
alignment: Alignment.bottomCenter,
child: Text(
'Twitter is an online micro-blogging platform that enables you to post short updates with images and videos attached. The social network was launched in 2006 and has more than 300 million monthly active users around the world.',
selectionColor: Colors.black,
textAlign: TextAlign.center,
semanticsLabel: 'pakistan',
style: TextStyle(fontSize: 15, color: Colors.black),
),
),
),
),
Padding(
padding: const EdgeInsets.all(50.0),
child: GFImageOverlay(
colorFilter: ColorFilter.mode(
Colors.black.withOpacity(0),
BlendMode.darken,
),
boxFit: BoxFit.fitWidth,
image: const AssetImage('assets/images/whatsapp.png'),
child: const Align(
alignment: Alignment.bottomCenter,
child: Text(
'WhatsApp started as an alternative to SMS. Our product now supports sending and receiving a variety of media: text, photos, videos, documents, and location, as well as voice calls. Some of your most personal moments are shared with WhatsApp, which is why we built end-to-end encryption into our app.',
selectionColor: Colors.black,
textAlign: TextAlign.center,
semanticsLabel: 'pakistan',
style: TextStyle(fontSize: 15, color: Colors.black),
),
),
),
),
Padding(
padding: const EdgeInsets.all(50.0),
child: GFImageOverlay(
height: MediaQuery.of(context).size.height,
colorFilter: ColorFilter.mode(
Colors.black.withOpacity(0),
BlendMode.darken,
),
width: 100,
padding: const EdgeInsets.all(0),
borderRadius: const BorderRadius.all(Radius.circular(50)),
boxFit: BoxFit.fitWidth,
alignment: Alignment.bottomCenter,
shape: BoxShape.rectangle,
image: const AssetImage('assets/images/instagram.png'),
child: const Align(
alignment: Alignment.bottomCenter,
child: Text(
'Instagram is a free photo and video sharing app available on iPhone and Android. People can upload photos or videos to our service and share them with their followers or with a select group of friends. ',
selectionColor: Colors.black,
textAlign: TextAlign.center,
style: TextStyle(fontSize: 15, color: Colors.black),
),
),
),
),
],
),
),
);
}
}
Note (add images in your assets/images folder)
Screenshots
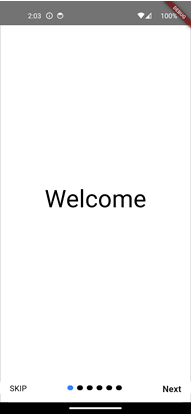
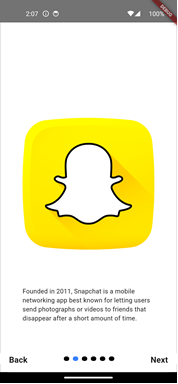
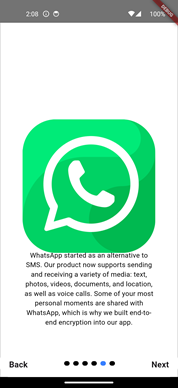