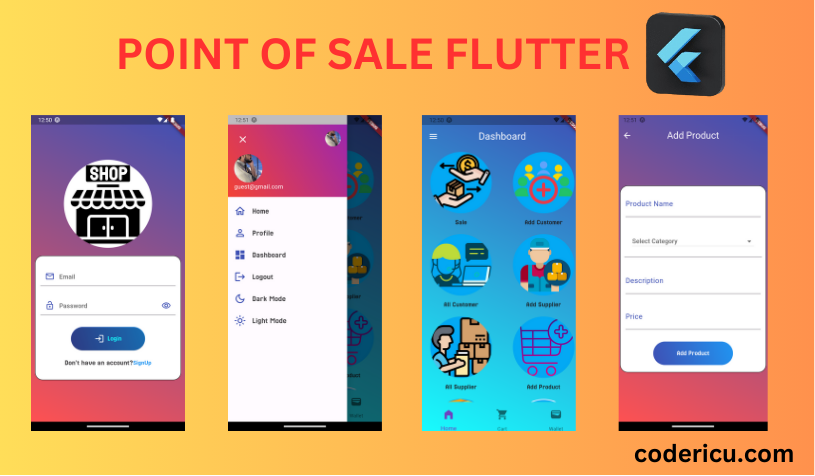
create your project
flutter create point_of_sale
add dependencies in your pubspec.yaml
get: ^4.6.6
getwidget: ^4.0.0
flutter_staggered_grid_view: ^0.7.0
main.dart
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:point_of_sale/screens/add_customer_screen.dart';
import 'package:point_of_sale/screens/add_product.dart';
import 'package:point_of_sale/screens/add_supplier.dart';
import 'package:point_of_sale/screens/cash_in_hand.dart';
import 'package:point_of_sale/screens/cash_ledger.dart';
import 'package:point_of_sale/screens/dashboard_screen.dart';
import 'package:point_of_sale/screens/signup_screen.dart';
import 'package:point_of_sale/screens/splash_screen.dart';
import 'screens/sale_details_screen.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return GetMaterialApp(
title: 'Flutter Demo',
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const SplashScreen(),
);
}
}
create controller.dart in lib folder
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:get/get.dart';
class HomeController extends GetxController {
final formKey = GlobalKey<FormState>();
late PageController pagecontoller = PageController();
validateEmail(String? email) {
if (GetUtils.isEmail(email ?? '')) {
return;
}
return " email is not valid";
}
validatePassword(String? password) {
if (!GetUtils.isLengthGreaterOrEqual(password, 6)) {
return "password is not valid";
}
return null;
}
Future CheckEmail() async {
final isValidate = formKey.currentState!.validate();
}
}
create widget folder in your lib folder
now create custom_textfield.dart under lib>>widget folder
custom_textfield.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
class CustomTextField extends StatelessWidget {
final String hinttext;
final List<TextInputFormatter>? inputformatters;
final String? Function(String?)? validator;
final void Function(String?)? onchange;
final Icon? icon;
final bool? obscuretext;
final TextInputType? keyboardtype;
final IconButton? sufixicon;
final TextEditingController? controller;
final String? labletext;
const CustomTextField({
super.key,
required this.hinttext,
this.inputformatters,
this.validator,
this.onchange,
this.icon,
this.obscuretext,
this.keyboardtype,
this.sufixicon,
this.controller,
this.labletext,
});
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(13.0),
child: TextFormField(
controller: controller,
keyboardType: keyboardtype,
inputFormatters: inputformatters,
validator: validator,
onChanged: onchange,
obscureText: false,
decoration: InputDecoration(
labelText: labletext,
labelStyle: const TextStyle(
color: Colors.indigo,
fontSize: 20,
),
suffixIcon: sufixicon,
suffixIconColor: Colors.indigo,
filled: true,
border: const OutlineInputBorder(
borderRadius: BorderRadius.all(
Radius.circular(20),
),
),
hintText: hinttext,
prefixIcon: icon,
prefixIconColor: Colors.indigo),
),
);
}
}
create classes folder under lib
now create lib>> classes>> dashboard_items.dart
dashboard_items.dart
import 'package:flutter/material.dart';
class Data {
String images;
String text;
Data({required this.images, required this.text});
}
create screens.dart under lib>>classes
class Screen {
var page;
Screen({required this.page});
}
create screens folder in lib
create splash_screen.dart under screens folder
import 'dart:async';
import 'dart:ui';
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:getwidget/components/image/gf_image_overlay.dart';
import 'package:point_of_sale/screens/login_screen.dart';
import 'package:point_of_sale/screens/signup_screen.dart';
import 'dashboard_screen.dart';
class SplashScreen extends StatefulWidget {
const SplashScreen({super.key});
@override
State<SplashScreen> createState() => _SplashScreenState();
}
class _SplashScreenState extends State<SplashScreen> {
@override
void initState() {
Timer(const Duration(seconds: 5), () {
Get.to(const LoginScreen());
});
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
backgroundColor: Colors.black12,
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const Align(
alignment: Alignment.topCenter,
child: Text(
'Point Of Sale',
style: TextStyle(
color: Colors.white60,
fontSize: 35,
fontWeight: FontWeight.bold,
fontFamily: "Jersey"),
),
),
GFImageOverlay(
height: 300,
width: 270,
colorFilter:
ColorFilter.mode(Colors.black.withOpacity(0), BlendMode.darken),
image: const AssetImage('assets/shop (1).png'),
),
const SizedBox(
height: 100,
),
],
),
bottomNavigationBar: const Padding(
padding: EdgeInsets.only(top: 80.0, left: 130),
child: Text(
'CopyRights © 2024',
style: TextStyle(
color: Colors.white60, fontFamily: "Jersey", fontSize: 15),
),
),
);
}
}
create login_screen.dart
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:getwidget/getwidget.dart';
import 'package:point_of_sale/screens/dashboard_screen.dart';
import 'package:point_of_sale/screens/signup_screen.dart';
import 'package:point_of_sale/widgets/custom_textfield.dart';
import 'package:point_of_sale/controller.dart';
class LoginScreen extends StatelessWidget {
const LoginScreen({super.key});
@override
Widget build(BuildContext context) {
TextEditingController emailController = TextEditingController();
TextEditingController passwordController = TextEditingController();
final controller = Get.put(HomeController());
return Scaffold(
body: SafeArea(
child: SingleChildScrollView(
child: Padding(
padding: const EdgeInsets.only(top: 90.0),
child: Form(
key: controller.formKey,
child: Column(
children: [
const GFImageOverlay(
image: AssetImage('assets/shop.png'),
height: 200,
width: 150,
colorFilter:
ColorFilter.mode(Colors.white, BlendMode.darken),
),
CustomTextField(
obscuretext: false,
controller: emailController,
hinttext: 'Email',
labletext: "Email",
icon: const Icon(Icons.email_outlined),
validator: (email) => controller.validateEmail(email),
),
CustomTextField(
controller: passwordController,
obscuretext: false,
labletext: "Password",
hinttext: 'Password',
icon: const Icon(Icons.lock_open),
validator: (password) =>
controller.validatePassword(password),
),
const SizedBox(
height: 20,
),
Padding(
padding: const EdgeInsets.all(18.0),
child: GFButton(
onPressed: () {
controller.CheckEmail();
if (emailController.text == "admin@gmail.com" &&
passwordController.text == "123456") {
Get.to(DashboardScreen());
}
return;
},
shape: GFButtonShape.pills,
elevation: 10,
clipBehavior: Clip.antiAliasWithSaveLayer,
color: GFColors.PRIMARY,
size: 60,
hoverColor: GFColors.FOCUS,
focusColor: GFColors.SECONDARY,
icon: Icon(
Icons.login_outlined,
size: 25,
color: Colors.white,
),
fullWidthButton: true,
child: const Text(
'Login',
style: TextStyle(fontSize: 18, fontFamily: 'Jersey'),
),
),
),
const SizedBox(
height: 50,
),
const Divider(
height: 2,
endIndent: 60,
indent: 60,
thickness: 2,
),
const SizedBox(
height: 50,
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const Text(
"Don't have an account?",
style: TextStyle(fontSize: 19, fontFamily: 'Jersey'),
),
GestureDetector(
child: const Text(
'SignUp',
style: TextStyle(
color: Colors.blue, fontWeight: FontWeight.bold),
),
onTap: () {
Get.to(const SignUpScreen());
},
)
],
)
],
),
),
),
),
),
);
}
}
create singup_screen.dart under screens folder
singup_screen.dart
import 'package:flutter/cupertino.dart';
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:get/get.dart';
import 'package:getwidget/colors/gf_color.dart';
import 'package:getwidget/getwidget.dart';
import 'package:point_of_sale/screens/login_screen.dart';
class SignUpScreen extends StatelessWidget {
const SignUpScreen({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
centerTitle: true,
title: const Text(
'SignUp',
style: TextStyle(
color: Colors.black, fontFamily: "Jersey", fontSize: 35),
),
backgroundColor: Colors.blueAccent,
automaticallyImplyLeading: false,
),
body: SingleChildScrollView(
child: Column(
children: [
const SizedBox(
height: 29,
),
const Padding(
padding: EdgeInsets.all(13.0),
child: TextField(
decoration: InputDecoration(
focusColor: Colors.blue,
filled: true,
border: OutlineInputBorder(
borderRadius: BorderRadius.all(
Radius.circular(20),
),
),
hintText: 'Name',
prefixIcon: Icon(Icons.person),
prefixIconColor: Colors.indigo,
label: Text("Name"),
labelStyle: TextStyle(color: Colors.black),
),
),
),
const Padding(
padding: EdgeInsets.all(13.0),
child: TextField(
decoration: InputDecoration(
filled: true,
focusColor: Colors.blue,
border: OutlineInputBorder(
borderRadius: BorderRadius.all(
Radius.circular(20),
),
),
hintText: 'Email',
prefixIcon: Icon(Icons.email_outlined),
prefixIconColor: Colors.indigo,
label: Text("Email"),
labelStyle: TextStyle(color: Colors.black),
),
),
),
const Padding(
padding: EdgeInsets.all(13.0),
child: TextField(
obscureText: true,
decoration: InputDecoration(
filled: true,
focusColor: Colors.blue,
border: OutlineInputBorder(
borderRadius: BorderRadius.all(
Radius.circular(20),
),
),
hintText: 'Password',
prefixIcon: Icon(Icons.password_outlined),
prefixIconColor: Colors.indigo,
label: Text(" Password"),
labelStyle: TextStyle(color: Colors.black),
),
),
),
const Padding(
padding: EdgeInsets.all(13.0),
child: TextField(
obscureText: true,
decoration: InputDecoration(
filled: true,
focusColor: Colors.blue,
border: OutlineInputBorder(
borderRadius: BorderRadius.all(
Radius.circular(20),
),
),
hintText: 'Confirm Password',
prefixIcon: Icon(Icons.password_outlined),
prefixIconColor: Colors.indigo,
label: Text("Confirm Password"),
labelStyle: TextStyle(color: Colors.black),
),
),
),
Padding(
padding: const EdgeInsets.all(13.0),
child: TextField(
keyboardType: TextInputType.number,
inputFormatters: [FilteringTextInputFormatter.digitsOnly],
decoration: const InputDecoration(
filled: true,
focusColor: Colors.blue,
border: OutlineInputBorder(
borderRadius: BorderRadius.all(Radius.circular(20))),
hintText: 'Phone Number',
prefixIcon: Icon(Icons.phone),
prefixIconColor: Colors.indigo,
label: Text("Phone Number"),
labelStyle: TextStyle(color: Colors.black),
),
),
),
const SizedBox(
height: 20,
),
Padding(
padding: const EdgeInsets.all(18.0),
child: GFButton(
onPressed: () {},
shape: GFButtonShape.pills,
color: GFColors.PRIMARY,
size: 60,
hoverColor: GFColors.FOCUS,
focusColor: GFColors.SECONDARY,
fullWidthButton: true,
child: const Text(
'SignUp',
style: TextStyle(fontSize: 18, fontFamily: 'Jersey'),
),
),
),
const SizedBox(
height: 50,
),
const Divider(
indent: 50,
endIndent: 50,
thickness: 1,
color: Colors.indigo,
),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
const Text(
"Already have an account?",
style: TextStyle(fontSize: 19, fontFamily: 'Jersey'),
),
GestureDetector(
child: const Text(
'Login',
style: TextStyle(
color: Colors.blue,
fontWeight: FontWeight.bold,
fontFamily: 'Jersey',
fontSize: 19),
),
onTap: () {
Get.to(const LoginScreen());
},
)
],
)
],
),
),
);
}
}
create dashboard_screen.dart under screens folder
import 'package:flutter/material.dart';
import 'package:get/get.dart';
import 'package:getwidget/getwidget.dart';
import 'package:point_of_sale/classes/dashboard_items.dart';
import 'package:point_of_sale/classes/screens.dart';
import 'package:point_of_sale/screens/add_supplier.dart';
import 'package:point_of_sale/screens/profile_screen.dart';
import 'package:point_of_sale/screens/sale_details_screen.dart';
import 'add_customer_screen.dart';
import 'add_product.dart';
import 'all_customer.dart';
import 'all_supplier.dart';
import 'cash_in_hand.dart';
import 'cash_ledger.dart';
import 'sale_screen.dart';
class DashboardScreen extends StatefulWidget {
DashboardScreen({super.key});
@override
State<DashboardScreen> createState() => _DashboardScreenState();
}
class _DashboardScreenState extends State<DashboardScreen> {
final List _items = [
Data(images: "assets/direct-marketing.png", text: "Sale"),
Data(images: "assets/queue.png", text: "Add Customer"),
Data(images: "assets/supplier.png", text: "Add Supplier"),
Data(images: "assets/shopping-cart.png", text: "Add Product"),
Data(images: "assets/journal.png", text: "Cash Ledger"),
Data(images: "assets/money.png", text: "Cash in Hand"),
Data(images: "assets/analytics.png", text: "Sale Details"),
Data(images: "assets/consultant.png", text: "All Customer"),
Data(images: "assets/inventory.png", text: "All All Supplier"),
];
final List _screens = [
Screen(page: const SaleScreen()),
Screen(page: const AddCustomer()),
Screen(page: const AddSupplier()),
Screen(page: const AddProduct()),
Screen(page: const CashLedger()),
Screen(page: const CashInHand()),
Screen(page: const SaleDetails()),
Screen(page: const AllCustomer()),
Screen(page: const AllSupplier()),
];
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: GFAppBar(
leading: Builder(
builder: (context) => IconButton(
icon: new Icon(Icons.menu),
onPressed: () => Scaffold.of(context).openDrawer(),
),
),
title: const Text('Dashboard'),
centerTitle: true,
backgroundColor: Colors.lightBlueAccent,
automaticallyImplyLeading: false,
),
drawer: GFDrawer(
child: ListView(
children: [
const GFDrawerHeader(
centerAlign: false,
currentAccountPicture: GFAvatar(
radius: 80.0,
backgroundImage: AssetImage("assets/me.jpg"),
size: 100,
),
otherAccountsPictures: <Widget>[
GFAvatar(
backgroundImage: AssetImage("assets/me.jpg"),
)
],
child: Column(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text(
'Sanaullah@gmail.com',
style: TextStyle(color: Colors.white, fontSize: 15),
),
],
),
),
const ListTile(
leading: Icon(
Icons.home_outlined,
size: 30,
),
title: Text(
'Home',
style: TextStyle(fontFamily: "jersey", fontSize: 20),
),
onTap: null,
iconColor: Colors.indigo,
),
ListTile(
leading: const Icon(
Icons.perm_identity,
size: 30,
),
title: const Text(
'Profile',
style: TextStyle(fontFamily: "jersey", fontSize: 20),
),
onTap: () {
Get.to(() => const ProfileScreen());
},
iconColor: Colors.indigo,
),
const ListTile(
leading: Icon(
Icons.dashboard,
size: 30,
),
title: Text(
'Dashboard',
style: TextStyle(fontFamily: "jersey", fontSize: 20),
),
onTap: null,
iconColor: Colors.indigo,
),
const ListTile(
leading: Icon(
Icons.logout,
size: 30,
),
title: Text(
'Logout',
style: TextStyle(fontFamily: "jersey", fontSize: 20),
),
onTap: null,
iconColor: Colors.indigo,
),
ListTile(
leading: const Icon(
Icons.dark_mode_outlined,
size: 30,
),
title: const Text(
'Dark Mode',
style: TextStyle(fontFamily: "jersey", fontSize: 20),
),
onTap: () {
Get.changeTheme(ThemeData.dark());
},
iconColor: Colors.indigo,
),
ListTile(
leading: const Icon(
Icons.light_mode_outlined,
size: 30,
),
title: const Text(
'Light Mode',
style: TextStyle(fontFamily: "jersey", fontSize: 20),
),
onTap: () {
Get.changeTheme(ThemeData.light());
},
iconColor: Colors.indigo,
),
],
),
),
bottomNavigationBar: BottomNavigationBar(
iconSize: 30,
currentIndex: 0,
items: [
BottomNavigationBarItem(
label: "Home",
icon: IconButton(
onPressed: () {},
icon: const Icon(
Icons.home_filled,
),
),
),
BottomNavigationBarItem(
label: "Cart",
icon: IconButton(
onPressed: () {},
icon: const Icon(
Icons.shopping_cart,
),
),
),
BottomNavigationBarItem(
label: "Wallet",
icon: IconButton(
onPressed: () {},
icon: const Icon(
Icons.wallet,
),
),
),
// BottomNavigationBarItem(
// label: "Profile",
// icon: IconButton(
// onPressed: () {},
// icon: Icon(
// Icons.account_circle_rounded,
// ),
// ),
// ),
],
),
body: Padding(
padding: const EdgeInsets.only(top: 10.0),
child: GridView.builder(
itemCount: _items.length,
gridDelegate: const SliverGridDelegateWithFixedCrossAxisCount(
crossAxisCount: 2, mainAxisSpacing: 10, crossAxisSpacing: 10),
itemBuilder: (context, index) {
return Column(
children: [
InkWell(
onTap: () {
Get.to(_screens[index].page);
},
child: GFImageOverlay(
boxFit: BoxFit.fitHeight,
height: 150,
image: AssetImage(_items[index].images),
colorFilter:
const ColorFilter.mode(Colors.white, BlendMode.darken),
),
),
const SizedBox(
height: 10,
),
Text(
_items[index].text,
style: const TextStyle(fontFamily: "Jersey", fontSize: 17),
),
],
);
},
//controller: PageController(),
),
),
);
}
}
profile_screen.dart
import 'package:flutter/material.dart';
class ProfileScreen extends StatelessWidget {
const ProfileScreen({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Profile"),
centerTitle: true,
),
);
}
}
drawer_tab.dart
import 'package:flutter/material.dart';
import 'package:getwidget/getwidget.dart';
class DrawerScreen extends StatefulWidget {
const DrawerScreen({super.key});
@override
State<DrawerScreen> createState() => _DrawerScreenState();
}
class _DrawerScreenState extends State<DrawerScreen> {
@override
Widget build(BuildContext context) {
return GFDrawer(
child: ListView(
padding: EdgeInsets.zero,
children: const <Widget>[
GFDrawerHeader(
currentAccountPicture: GFAvatar(
radius: 80.0,
backgroundImage: AssetImage("assets/me.jpg"),
),
otherAccountsPictures: <Widget>[
Image(
image: NetworkImage(
"https://cdn.pixabay.com/photo/2019/12/20/00/03/road-4707345_960_720.jpg"),
fit: BoxFit.cover,
),
GFAvatar(
child: Text("Profile"),
)
],
child: Column(
mainAxisAlignment: MainAxisAlignment.start,
crossAxisAlignment: CrossAxisAlignment.start,
children: <Widget>[
Text('user name'),
Text('user@userid.com'),
],
),
),
ListTile(
title: Text('Item 1'),
onTap: null,
),
ListTile(
title: Text('Item 2'),
onTap: null,
),
],
),
);
}
}
sale_screen.dart
import 'package:flutter/material.dart';
import 'package:flutter_staggered_grid_view/flutter_staggered_grid_view.dart';
import 'package:getwidget/components/image/gf_image_overlay.dart';
class SaleScreen extends StatelessWidget {
const SaleScreen({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Sales'),
centerTitle: true,
backgroundColor: Colors.lightBlueAccent,
actions: [IconButton(onPressed: () {}, icon: Icon(Icons.add))],
),
body: SingleChildScrollView(
child: Column(
children: [
StaggeredGrid.count(
crossAxisCount: 4,
mainAxisSpacing: 10,
crossAxisSpacing: 10,
children: [
StaggeredGridTile.count(
crossAxisCellCount: 4,
mainAxisCellCount: 2,
child: GestureDetector(
onTap: () {},
child: Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.black)),
child: Image.asset(
"assets/shoes.png",
),
),
),
),
StaggeredGridTile.count(
crossAxisCellCount: 2,
mainAxisCellCount: 2,
child: GestureDetector(
onTap: () {},
child: Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.black)),
child: Image.asset('assets/watch.png')),
),
),
StaggeredGridTile.count(
crossAxisCellCount: 2,
mainAxisCellCount: 3,
child: GestureDetector(
onTap: () {},
child: Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.black)),
child: Image.asset(
"assets/glasses.png",
),
),
)),
StaggeredGridTile.count(
crossAxisCellCount: 2,
mainAxisCellCount: 2,
child: GestureDetector(
onTap: () {},
child: Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.black)),
child: Image.asset(
"assets/phone.png",
fit: BoxFit.fill,
),
),
),
),
StaggeredGridTile.count(
crossAxisCellCount: 2,
mainAxisCellCount: 2,
child: GestureDetector(
onTap: () {},
child: Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.black)),
child: Image.asset("assets/laptop.png")),
),
),
StaggeredGridTile.count(
crossAxisCellCount: 2,
mainAxisCellCount: 2,
child: Container(
decoration: BoxDecoration(
border: Border.all(color: Colors.black)),
child: Image.asset("assets/pen.png"))),
],
),
],
),
),
);
}
}
sale_details.dart
import 'package:flutter/material.dart';
import 'package:getwidget/components/button/gf_icon_button.dart';
import 'package:getwidget/getwidget.dart';
import 'package:point_of_sale/widgets/custom_textfield.dart';
class SaleDetails extends StatelessWidget {
const SaleDetails({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Sales Details"),
centerTitle: true,
backgroundColor: Colors.lightBlueAccent,
),
body: SingleChildScrollView(
child: Column(
children: [
CustomTextField(hinttext: "Item Code"),
CustomTextField(hinttext: "Item Name"),
CustomTextField(hinttext: "Quantity"),
Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
GFIconButton(
size: 30,
icon: Icon(Icons.add_circle_outline),
onPressed: () {}),
SizedBox(
width: 10,
),
GFIconButton(size: 30, icon: Icon(Icons.add), onPressed: () {}),
],
),
CustomTextField(hinttext: "Unit"),
CustomTextField(hinttext: "Discount%"),
CustomTextField(hinttext: "Price"),
CustomTextField(hinttext: "Total Price"),
Padding(
padding: const EdgeInsets.all(18.0),
child: GFButton(
onPressed: () {},
shape: GFButtonShape.pills,
color: GFColors.PRIMARY,
size: 60,
hoverColor: GFColors.FOCUS,
focusColor: GFColors.SECONDARY,
fullWidthButton: true,
child: const Text(
'Save',
style: TextStyle(fontSize: 18, fontFamily: 'Jersey'),
),
),
),
],
),
),
);
}
}
add_customer.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:getwidget/getwidget.dart';
import 'package:point_of_sale/widgets/custom_textfield.dart';
class AddCustomer extends StatelessWidget {
const AddCustomer({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Add Customer'),
centerTitle: true,
backgroundColor: Colors.lightBlueAccent,
automaticallyImplyLeading: true,
),
body: SingleChildScrollView(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
const CustomTextField(
hinttext: 'Name',
icon: Icon(Icons.person),
),
CustomTextField(
hinttext: 'Phone',
icon: const Icon(Icons.phone),
inputformatters: [FilteringTextInputFormatter.digitsOnly],
keyboardtype: TextInputType.number,
),
const CustomTextField(
hinttext: 'Email',
icon: Icon(Icons.email_outlined),
),
const CustomTextField(
hinttext: 'Address',
),
const CustomTextField(
hinttext: 'Create Password',
icon: Icon(Icons.lock_outline),
obscuretext: true,
),
const CustomTextField(
hinttext: 'Confirm Password',
icon: Icon(Icons.lock_outline),
obscuretext: true,
),
const SizedBox(
height: 20,
),
Padding(
padding: const EdgeInsets.all(18.0),
child: GFButton(
onPressed: () {},
shape: GFButtonShape.pills,
color: GFColors.PRIMARY,
size: 60,
hoverColor: GFColors.FOCUS,
focusColor: GFColors.SECONDARY,
fullWidthButton: true,
child: const Text(
'Add Customer',
style: TextStyle(fontSize: 18, fontFamily: 'Jersey'),
),
),
),
],
),
),
);
}
}
all_customers.dart
import 'package:flutter/material.dart';
import 'package:getwidget/components/appbar/gf_appbar.dart';
import 'package:getwidget/getwidget.dart';
class AllCustomer extends StatefulWidget {
const AllCustomer({super.key});
@override
State<AllCustomer> createState() => _AllCustomerState();
}
class _AllCustomerState extends State<AllCustomer> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: GFAppBar(
title: Text("All Customer"),
centerTitle: true,
),
body: ListView(
children: [
ListTile(
leading: const GFAvatar(
backgroundImage: AssetImage("assets/user.png"),
),
title: const Text("Name"),
subtitle: const Text("Contact Number"),
trailing: IconButton(
onPressed: () {},
icon: Icon(Icons.delete),
),
onTap: () {},
),
ListTile(
leading: const GFAvatar(
backgroundImage: AssetImage("assets/user.png"),
),
title: const Text("Name"),
subtitle: const Text("Contact Number"),
trailing: IconButton(
onPressed: () {},
icon: Icon(Icons.delete),
),
onTap: () {},
),
ListTile(
leading: const GFAvatar(
backgroundImage: AssetImage("assets/user.png"),
),
title: const Text("Name"),
subtitle: const Text("Contact Number"),
trailing: IconButton(
onPressed: () {},
icon: Icon(Icons.delete),
),
onTap: () {},
),
],
),
);
}
}
add_supplier.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:getwidget/getwidget.dart';
import 'package:point_of_sale/widgets/custom_textfield.dart';
class AddSupplier extends StatelessWidget {
const AddSupplier({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text('Add Supplier'),
centerTitle: true,
backgroundColor: Colors.lightBlueAccent,
automaticallyImplyLeading: true,
),
body: SingleChildScrollView(
child: Column(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
const CustomTextField(
hinttext: 'Name',
icon: Icon(Icons.person),
),
CustomTextField(
hinttext: 'Phone',
icon: const Icon(Icons.phone),
inputformatters: [FilteringTextInputFormatter.digitsOnly],
keyboardtype: TextInputType.number,
),
const CustomTextField(
hinttext: 'Email',
icon: Icon(Icons.email_outlined),
),
const CustomTextField(
hinttext: 'Address',
),
const CustomTextField(
hinttext: 'Create Password',
icon: Icon(Icons.lock_outline),
obscuretext: true,
),
const CustomTextField(
hinttext: 'Confirm Password',
icon: Icon(Icons.lock_outline),
obscuretext: true,
),
const SizedBox(
height: 20,
),
Padding(
padding: const EdgeInsets.all(18.0),
child: GFButton(
onPressed: () {},
shape: GFButtonShape.pills,
color: GFColors.PRIMARY,
size: 60,
hoverColor: GFColors.FOCUS,
focusColor: GFColors.SECONDARY,
fullWidthButton: true,
child: const Text(
'Add Supplier',
style: TextStyle(fontSize: 18, fontFamily: 'Jersey'),
),
),
),
],
),
),
);
}
}
all_suppliers.dart
import 'package:flutter/material.dart';
import 'package:getwidget/components/appbar/gf_appbar.dart';
import 'package:getwidget/components/avatar/gf_avatar.dart';
class AllSupplier extends StatefulWidget {
const AllSupplier({super.key});
@override
State<AllSupplier> createState() => _AllSupplierState();
}
class _AllSupplierState extends State<AllSupplier> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: GFAppBar(
title: const Text("All Supplier"),
centerTitle: true,
),
body: ListView(
children: [
ListTile(
leading: const GFAvatar(
backgroundImage: AssetImage("assets/user.png"),
),
title: const Text("Name"),
subtitle: const Text("Contact Number"),
trailing: IconButton(
onPressed: () {},
icon: Icon(Icons.delete),
),
onTap: () {},
),
ListTile(
leading: const GFAvatar(
backgroundImage: AssetImage("assets/user.png"),
),
title: const Text("Name"),
subtitle: const Text("Contact Number"),
trailing: IconButton(
onPressed: () {},
icon: Icon(Icons.delete),
),
onTap: () {},
),
ListTile(
leading: const GFAvatar(
backgroundImage: AssetImage("assets/user.png"),
),
title: const Text("Name"),
subtitle: const Text("Contact Number"),
trailing: IconButton(
onPressed: () {},
icon: Icon(Icons.delete),
),
onTap: () {},
),
],
));
}
}
cash_in_hand.dart
import 'package:flutter/material.dart';
import 'package:point_of_sale/widgets/custom_textfield.dart';
class CashInHand extends StatelessWidget {
const CashInHand({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Cash in Hand"),
centerTitle: true,
backgroundColor: Colors.lightBlueAccent,
),
body: CustomTextField(hinttext: "All Amount Cash"),
);
}
}
cash_ledger.dart
import 'package:flutter/material.dart';
import 'package:getwidget/components/card/gf_card.dart';
import 'package:point_of_sale/widgets/custom_textfield.dart';
class CashLedger extends StatelessWidget {
const CashLedger({super.key});
@override
Widget build(BuildContext context) {
TextEditingController inAmountController = TextEditingController();
TextEditingController OutAmountController = TextEditingController();
return Scaffold(
appBar: AppBar(
title: Text("Cash Ledger"),
centerTitle: true,
backgroundColor: Colors.lightBlueAccent,
),
body: const Column(
mainAxisAlignment: MainAxisAlignment.start,
children: [
ListTile(
title: Text(
'Item Name',
style: TextStyle(fontFamily: "Jersey", fontSize: 25),
),
subtitle: Text(
"Amount",
style: TextStyle(fontFamily: "Jersey", fontSize: 25),
),
//Here is every thing which yu need for example on change or icon validator etc.
trailing: Text(
"In/out",
style: TextStyle(fontFamily: "Jersey", fontSize: 25),
),
),
],
),
);
}
}
Screenshorts
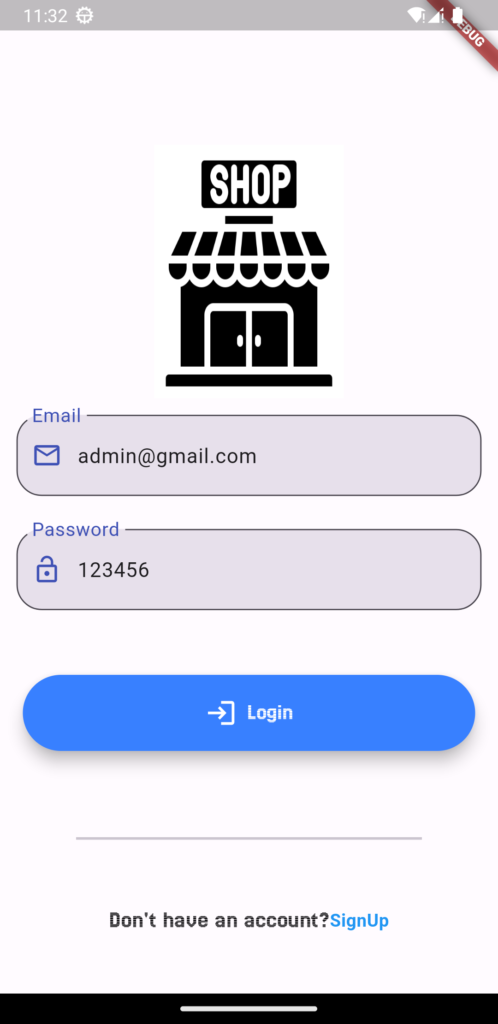
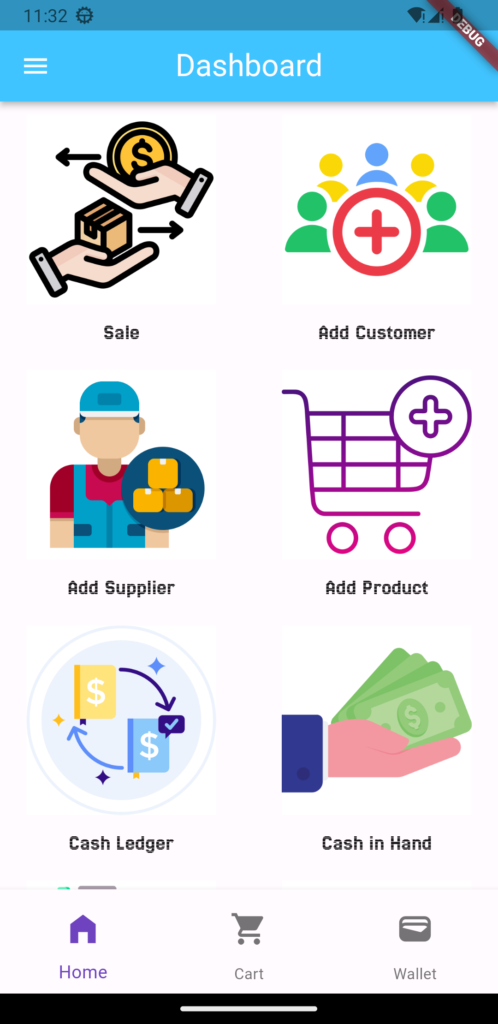
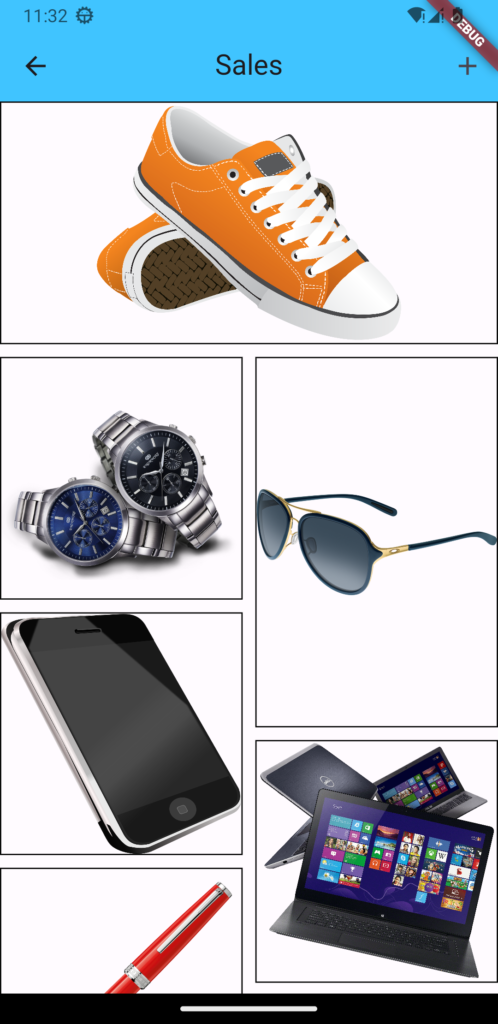
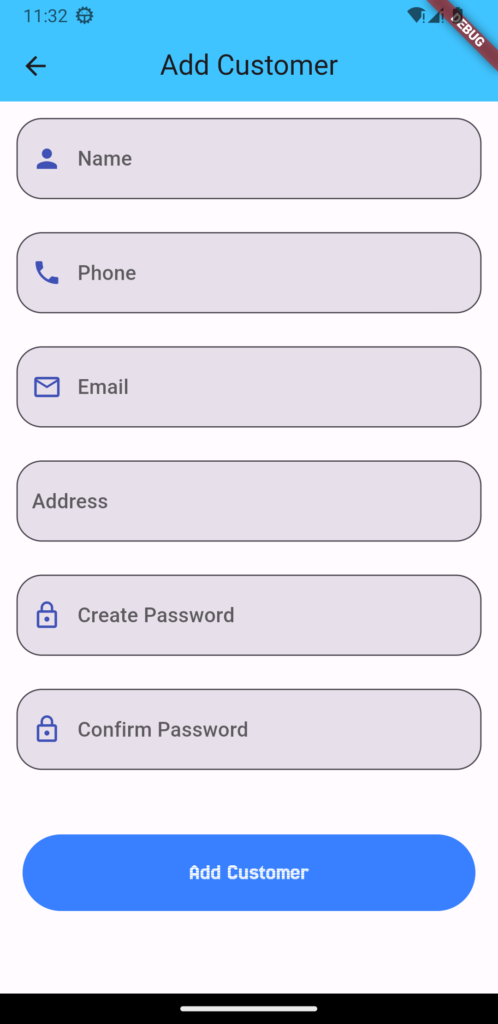
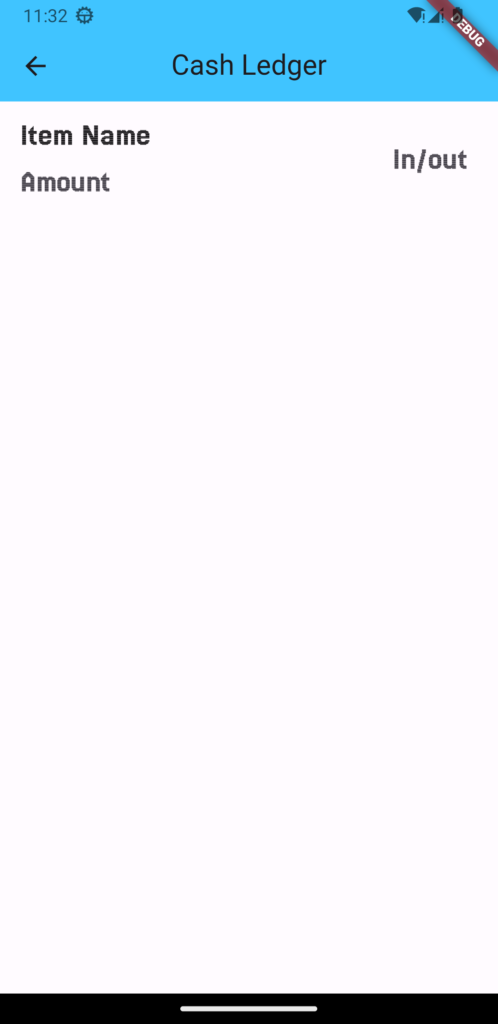
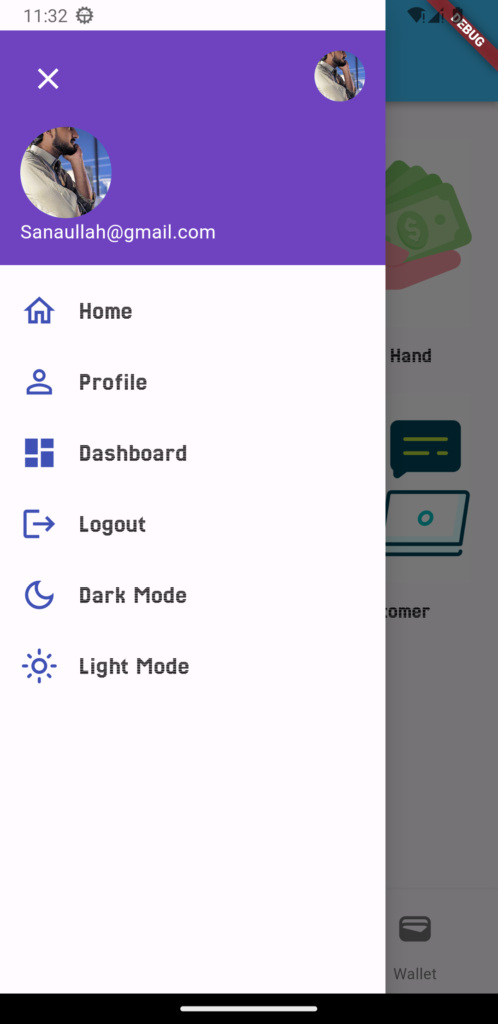
google voice how to make international calls
we can make international calls with agora, sinch, twilio sdk.
Email compliance with privacy laws secures customer data.
yes