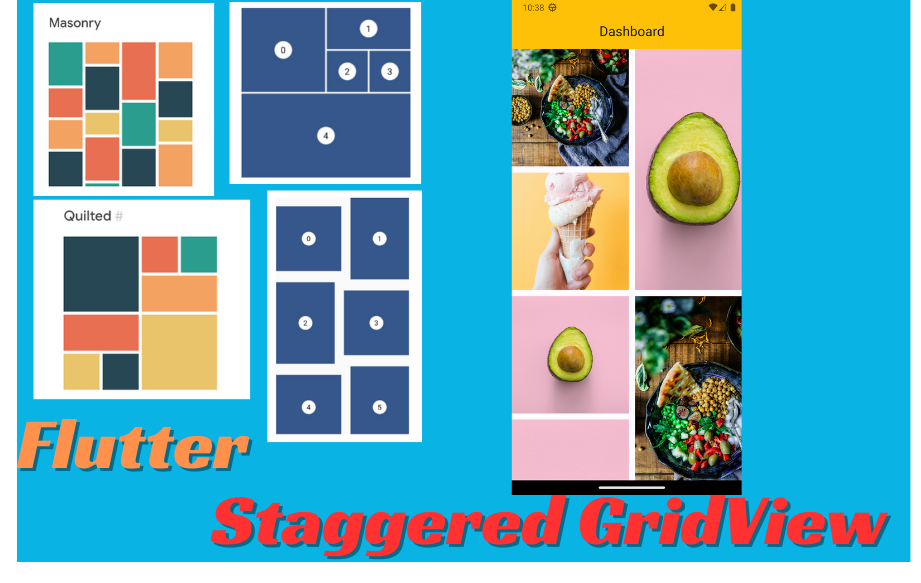
how to display items like instagram Search Screen
create your flutter project
flutter create projectname
once your project is created open your pubspec.yaml add the following dependency
flutter_staggered_grid_view: ^0.7.0
you can use any version of flutter_staggered_grid_view according to your flutter version check versions from here.
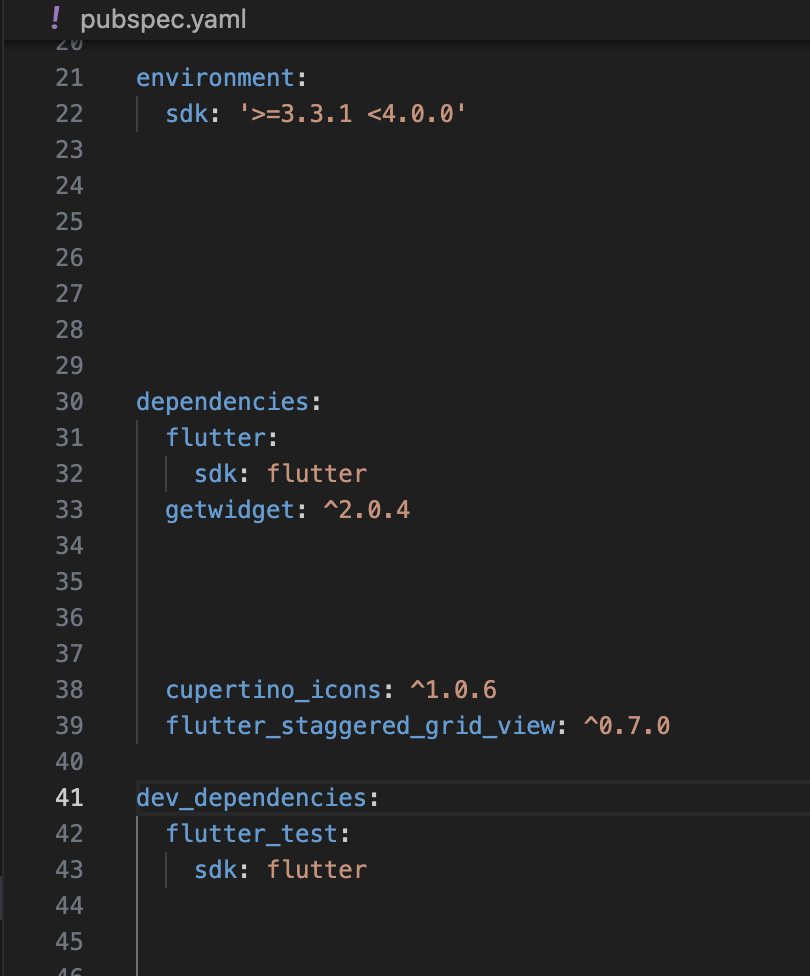
save or use following command in your terminal to get dependency
flutter pub get
create dashboard.dart in your lib folder
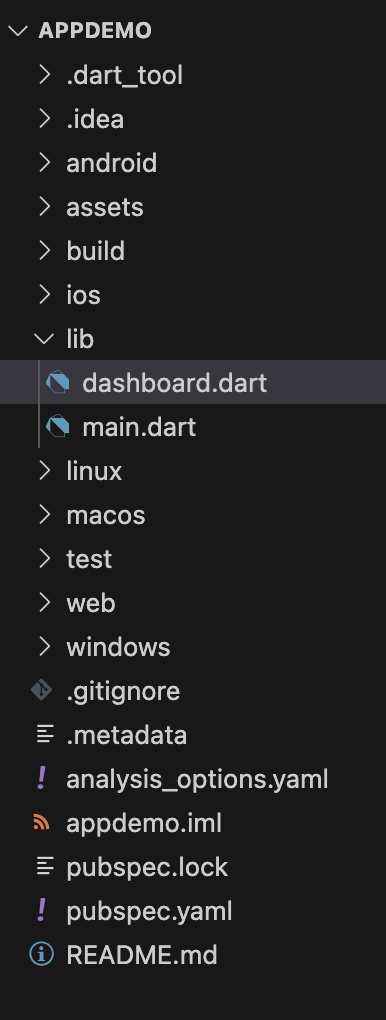
main.dart
import 'package:appdemo/dashboard.dart';
import 'package:flutter/material.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({super.key});
// This widget is the root of your application.
@override
Widget build(BuildContext context) {
return MaterialApp(
title: 'Flutter Demo',
debugShowCheckedModeBanner: false,
theme: ThemeData(
colorScheme: ColorScheme.fromSeed(seedColor: Colors.deepPurple),
useMaterial3: true,
),
home: const Dashboard(),
);
}
}
dashboardt.dart
import "package:flutter/material.dart";
import 'package:flutter_staggered_grid_view/flutter_staggered_grid_view.dart';
class Dashboard extends StatelessWidget {
const Dashboard({super.key});
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("Dashboard"),
centerTitle: true,
backgroundColor: Colors.amber,
),
body: SingleChildScrollView(
child: StaggeredGrid.count(
crossAxisCount: 2,
mainAxisSpacing: 10,
crossAxisSpacing: 10,
children: [
StaggeredGridTile.count(
crossAxisCellCount: 1,
mainAxisCellCount: 1,
child: Imagecontainer('assets/1.jpg'),
),
StaggeredGridTile.count(
crossAxisCellCount: 1,
mainAxisCellCount: 2,
child: Imagecontainer('assets/2.jpg'),
),
StaggeredGridTile.count(
crossAxisCellCount: 1,
mainAxisCellCount: 1,
child: Imagecontainer('assets/3.jpg'),
),
StaggeredGridTile.count(
crossAxisCellCount: 1,
mainAxisCellCount: 1,
child: Imagecontainer('assets/2.jpg'),
),
StaggeredGridTile.count(
crossAxisCellCount: 1,
mainAxisCellCount: 2,
child: Imagecontainer('assets/1.jpg'),
),
StaggeredGridTile.count(
crossAxisCellCount: 1,
mainAxisCellCount: 2,
child: Imagecontainer('assets/2.jpg'),
),
],
),
),
);
}
Imagecontainer(imgPath) {
return Image.asset(
imgPath,
fit: BoxFit.cover,
);
}
}
you can change design as per your requirements by changing crossAxisCellCount & mainAxisCellCount
StaggeredGridTile.count(
crossAxisCellCount: 1,
mainAxisCellCount: 1,
child: Imagecontainer('assets/1.jpg'),
)
Screenshots
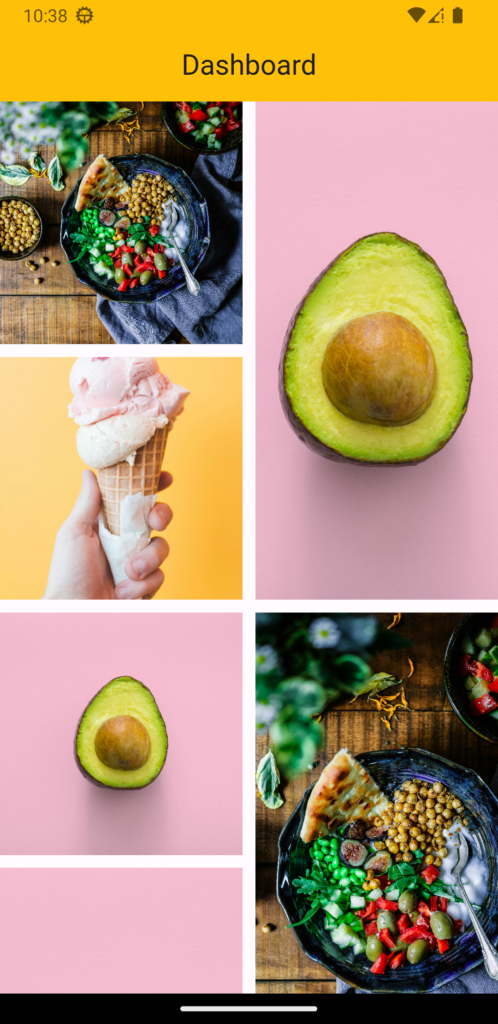