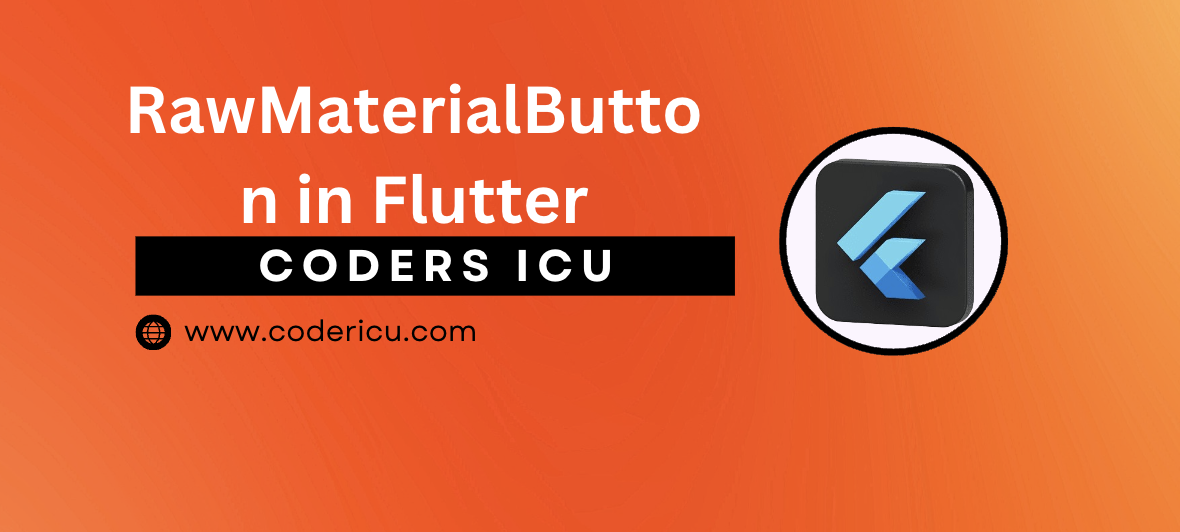
In Flutter, a RawMaterialButton is a widget that provides a basic button implementation without any visual styling or decoration. It is a raw, unstyled button that can be customized to fit your app’s design.
Properties:
- onPressed: The callback function to handle the button press.
- child: The widget to display the button’s content.
- padding: The padding around the button’s content.
- splash color: The color of the splash effect when the button is pressed.
- highlightColor: The color of the highlight effect when the button is pressed.
- elevation: The elevation of the button.
- shape: The shape of the button.
- clipBehavior: The clip behavior of the button.
RawMaterialButton(
onPressed: () {
print('Button pressed!');
},
child: Text('Raw Button'),
padding: EdgeInsets.all(16),
splashColor: Colors.blue,
highlightColor: Colors.red,
elevation: 5,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10),
),
)
import 'package:flutter/material.dart';
// Define the MeterialbuttonDemo widget, which is a StatefulWidget
class MeterialbuttonDemo extends StatefulWidget {
// Constructor for MeterialbuttonDemo widget
const MeterialbuttonDemo({Key? key}) : super(key: key);
@override
State<MeterialbuttonDemo> createState() => _MeterialbuttonDemoState();
}
// State class for MeterialbuttonDemo
class _MeterialbuttonDemoState extends State<MeterialbuttonDemo> {
@override
Widget build(BuildContext context) {
return SafeArea(
// Ensures that the widget is rendered within the safe area of the screen
child: Scaffold(
// Provides the basic material design visual layout structure
appBar: AppBar(
// Title of the AppBar
title: Text(
"Material Button",
style: TextStyle(
color: Colors.white, // Title text color
fontWeight: FontWeight.bold, // Title text weight
),
),
flexibleSpace: Container(
// Custom decoration for the AppBar
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.deepPurple, Colors.orangeAccent], // Gradient colors
begin: Alignment.topLeft, // Gradient start alignment
end: Alignment.topRight, // Gradient end alignment
),
),
),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
// MaterialButton widget
MaterialButton(
onPressed: () {
// Display a SnackBar when the button is pressed
ScaffoldMessenger.of(context).showSnackBar(
SnackBar(content: Text('MaterialButton pressed!')),
);
},
color: Colors.blue, // Background color of the button
textColor: Colors.white, // Text color of the button
padding: EdgeInsets.symmetric(horizontal: 20, vertical: 10), // Padding inside the button
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10), // Rounded corners of the button
),
elevation: 5, // Elevation (shadow) of the button
splashColor: Colors.lightBlueAccent, // Splash color when the button is pressed
child: Text('Material Button'), // Text inside the button
),
],
),
),
);
}
}
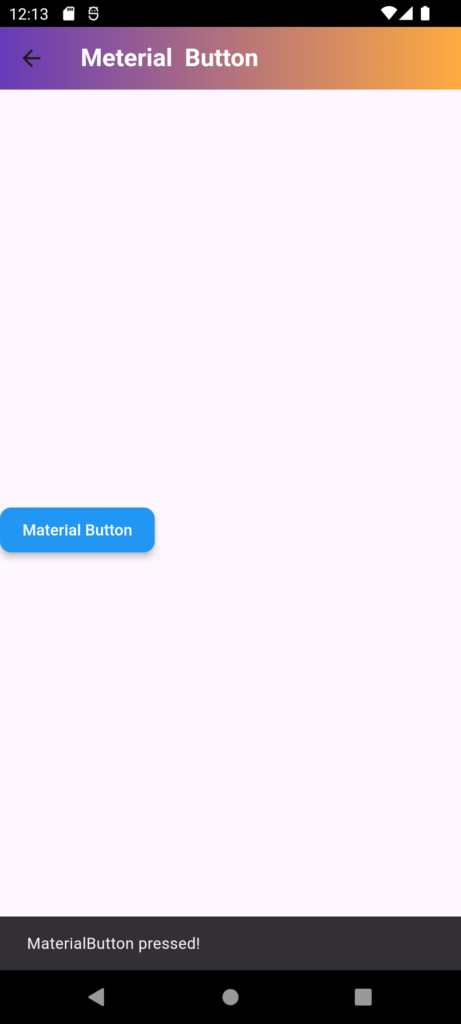
1 thought on “RawMaterialButton in Flutter”