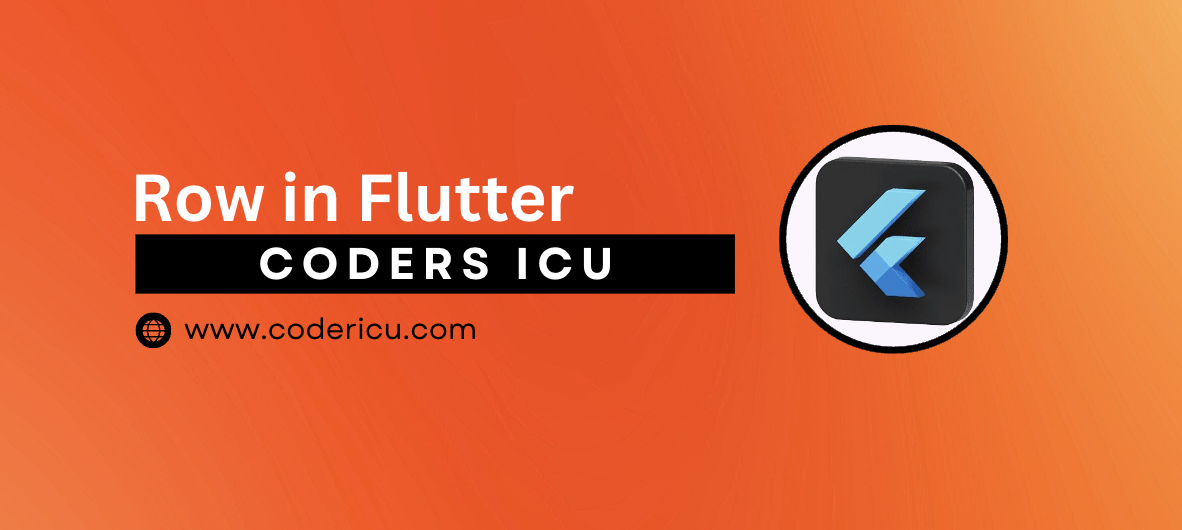
In Flutter, a Row is a widget that displays its children in a horizontal array. It is a built-in widget that allows you to create a row of widgets, such as text, images, or other widgets.
Properties
Properties of a Row
widget:
- children: A list of widgets to be displayed in the row
- mainAxisAlignment: Specifies how to align the children horizontally (e.g., start, center, end, space-between)
- crossAxisAlignment: Specifies how to align the children vertically (e.g., start, center, end, stretch)
- mainAxisSize: Specifies the width of the row (e.g., max, min)
Row(
children: [
Text('Hello'),
Text('World'),
Icon(Icons.heart),
],
)
// This code creates a row with three children: two text widgets and an icon widget.
Use cases:
- Create a horizontal array of widgets
- Display a list of items horizontally
- Create a custom toolbar or action bar
- Display a horizontal progress bar
Row is similar to Column, but it displays its children horizontally instead of vertically.
Code:
import 'package:flutter/material.dart';
class RowCode extends StatefulWidget {
const RowCode({Key? key}) : super(key: key);
@override
State<RowCode> createState() => _RowCodeState();
}
class _RowCodeState extends State<RowCode> {
@override
Widget build(BuildContext context) {
return SafeArea(
// SafeArea ensures that the widget is rendered within the visible screen area
child: Scaffold(
// Scaffold provides a high-level structure for visual elements of the app
appBar: AppBar(
// The AppBar widget is used for the top app bar of the screen
title: Text(
"Row Code",
style: TextStyle(
fontWeight: FontWeight.bold, // Makes the text bold
color: Colors.white, // Sets the text color to white
),
),
flexibleSpace: Container(
// Container used for customizing the app bar's background with decoration
decoration: BoxDecoration(
// BoxDecoration allows styling of the container
gradient: LinearGradient(
// LinearGradient creates a gradient effect for the background
colors: [Colors.deepPurple, Colors.orangeAccent], // Colors of the gradient
begin: Alignment.topLeft, // Gradient starts from the top-left
end: Alignment.topRight, // Gradient ends at the top-right
),
),
),
),
body: Row(
// Row arranges its children horizontally
crossAxisAlignment: CrossAxisAlignment.center, // Aligns children vertically in the center
mainAxisAlignment: MainAxisAlignment.center, // Aligns children horizontally in the center
children: [
// You can add multiple children here that will be arranged horizontally
// Example children could be Container, Text, or any other widget
Container(
width: 100,
height: 100,
color: Colors.red,
child: Center(child: Text('Red', style: TextStyle(color: Colors.white))),
),
SizedBox(width: 10), // Adds horizontal space between children
Container(
width: 100,
height: 100,
color: Colors.green,
child: Center(child: Text('Green', style: TextStyle(color: Colors.white))),
),
SizedBox(width: 10), // Adds horizontal space between children
Container(
width: 100,
height: 100,
color: Colors.blue,
child: Center(child: Text('Blue', style: TextStyle(color: Colors.white))),
),
],
),
),
);
}
}
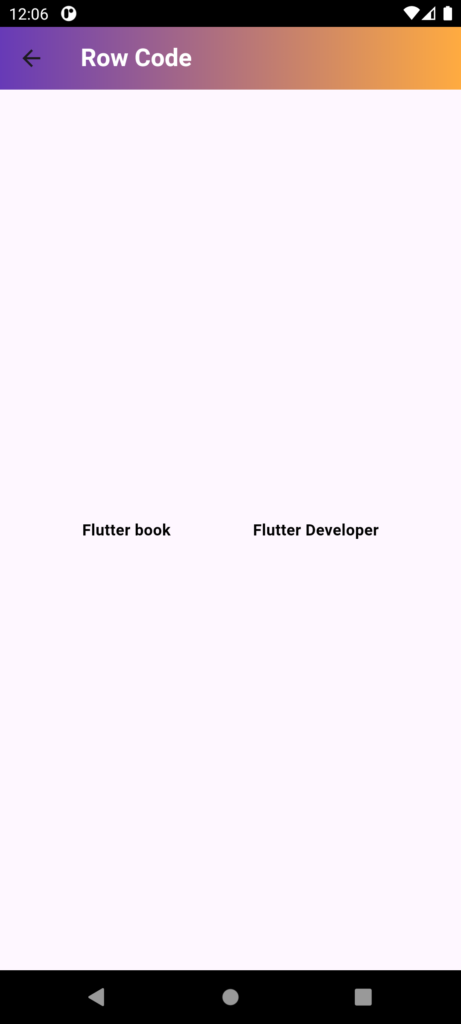