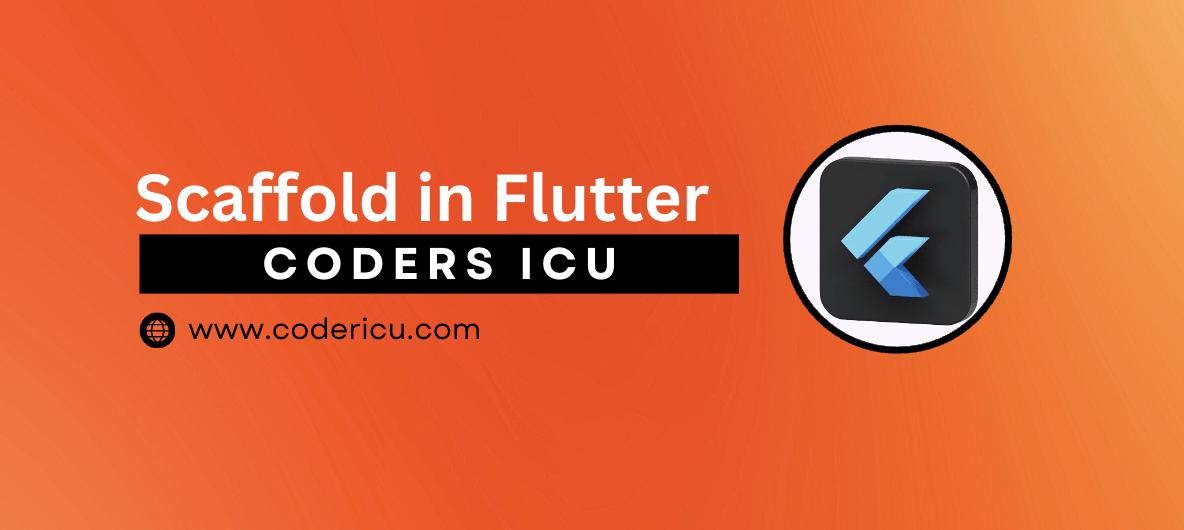
In Flutter, a Scaffold
is a top-level widget that provides a basic material design layout structure. It is a built-in widget that implements the basic material design visual layout structure, including:
- AppBar: A top app bar with a title, actions, and navigation menu
- Body: The main content area of the screen
backgroundColor
: Sets the background color of the scaffold.- FloatingActionButton: A floating action button that can be placed at the bottom right of the screen
- BottomNavigationBar: A bottom navigation bar with multiple tabs
- bottomSheet : A sheet that slides up from the bottom of the screen and can be used to display additional content or action
- Drawer: A side drawer that can be opened by swiping from the left edge of the screen
- SnackBar: A snack bar that can be used to display messages at the bottom of the screen
Example:
Scaffold(
appBar: AppBar(
title: Text('Hello, World!'),
),
body: Center(
child: Text('This is the body'),
),
floatingActionButton: FloatingActionButton(
onPressed: () {},
child: Icon(Icons.add),
),
)
A simple example demonstrating the use of Scaffold:
import 'package:flutter/material.dart';
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text('Scaffold Example'),
),
body: Center(
child: Text('Hello, Scaffold!'),
),
floatingActionButton: FloatingActionButton(
onPressed: () {
// Action to perform when the button is pressed
},
child: Icon(Icons.add),
),
drawer: Drawer(
child: ListView(
padding: EdgeInsets.zero,
children: <Widget>[
DrawerHeader(
child: Text('Drawer Header'),
decoration: BoxDecoration(
color: Colors.blue,
),
),
ListTile(
title: Text('Item 1'),
onTap: () {
// Update the state or navigate
Navigator.pop(context);
},
),
ListTile(
title: Text('Item 2'),
onTap: () {
// Update the state or navigate
Navigator.pop(context);
},
),
],
),
),
);
}
}