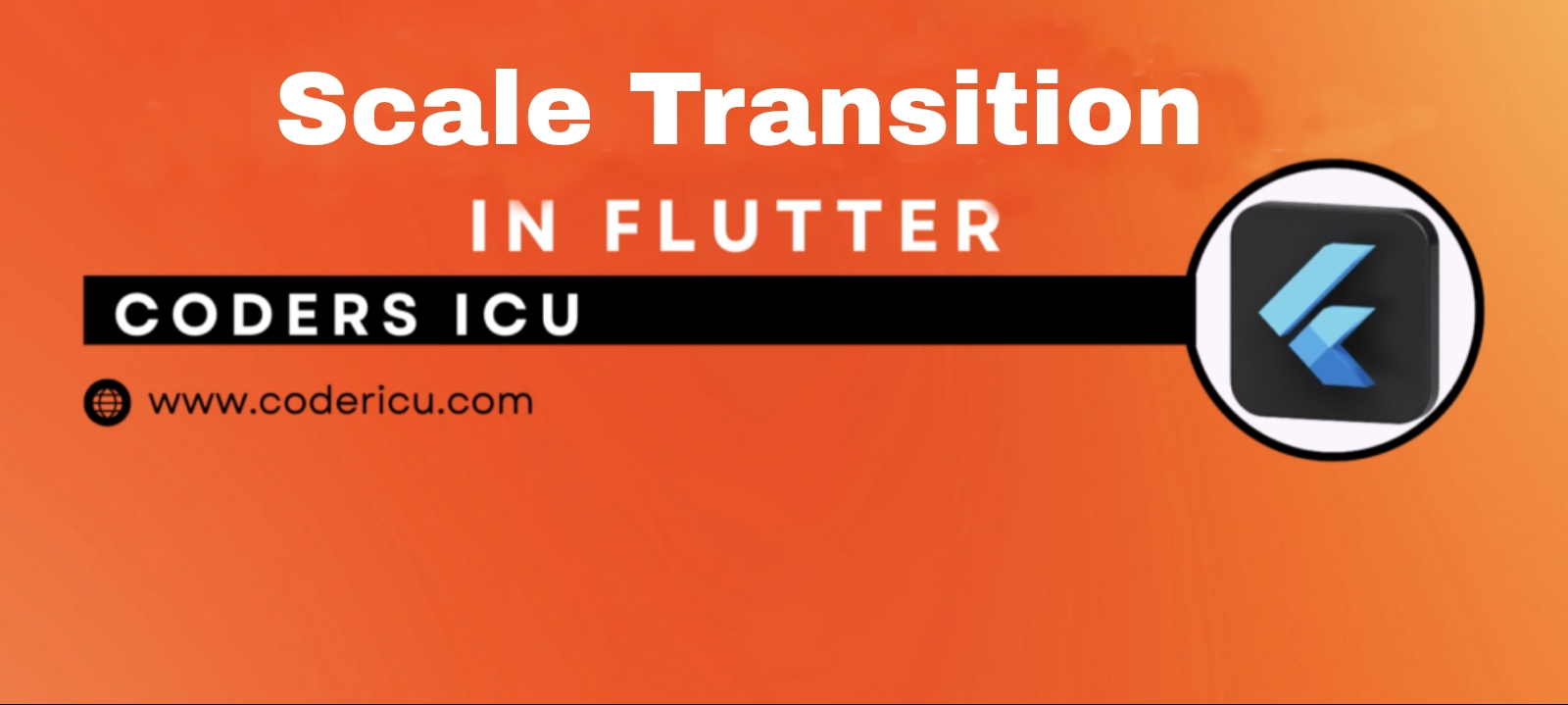
{"remix_data":[],"remix_entry_point":"challenges","source_tags":["local"],"origin":"unknown","total_draw_time":0,"total_draw_actions":0,"layers_used":0,"brushes_used":0,"photos_added":0,"total_editor_actions":{},"tools_used":{},"is_sticker":false,"edited_since_last_sticker_save":false,"containsFTESticker":false}
In Flutter, the ScaleTransition widget is used to create a scaling animation for a widget. It animates the scale (size) of a widget over a specific time duration, which is helpful when you want to create smooth scaling effects like zooming in or out.
How it works
The Scale Transition widget works by scaling the incoming route (the new screen) from a small size to its full size, while simultaneously scaling the outgoing route (the previous screen) from its full size to a smaller size.
Properties
Here are the key properties of the Scale Transition widget:
scale
: The scale factor to apply to the incoming route.alignment
: The alignment of the incoming route.filterQuality
: The filter quality to apply to the incoming route.
Example
import 'package:flutter/material.dart';
class ScaleTransitionScreen extends StatefulWidget {
const ScaleTransitionScreen({Key? key}) : super(key: key);
@override
_ScaleTransitionScreenState createState() => _ScaleTransitionScreenState();
}
class _ScaleTransitionScreenState extends State<ScaleTransitionScreen> with SingleTickerProviderStateMixin {
late AnimationController _controller;
late Animation<double> _animation;
@override
void initState() {
super.initState();
// Initializing the AnimationController with 2 seconds duration
_controller = AnimationController(
duration: const Duration(seconds: 2),
vsync: this,
)..repeat(reverse: true); // Repeats animation in reverse
// Defining the scale animation range from 0 to 1
_animation = Tween<double>(begin: 0, end: 1).animate(_controller);
}
@override
void dispose() {
// Dispose the controller when it's no longer needed
_controller.dispose();
super.dispose();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: const Text("ScaleTransition Example"),
flexibleSpace: Container(
decoration: const BoxDecoration(
gradient: LinearGradient(
colors: [Colors.deepPurple, Colors.orangeAccent],
begin: Alignment.topLeft,
end: Alignment.topRight,
),
),
),
),
body: Center(
child: ScaleTransition(
scale: _animation, // Applying the scale animation to the child
child: Container(
width: 100,
height: 100,
color: Colors.blue, // Blue box that will scale
),
),
),
);
}
}