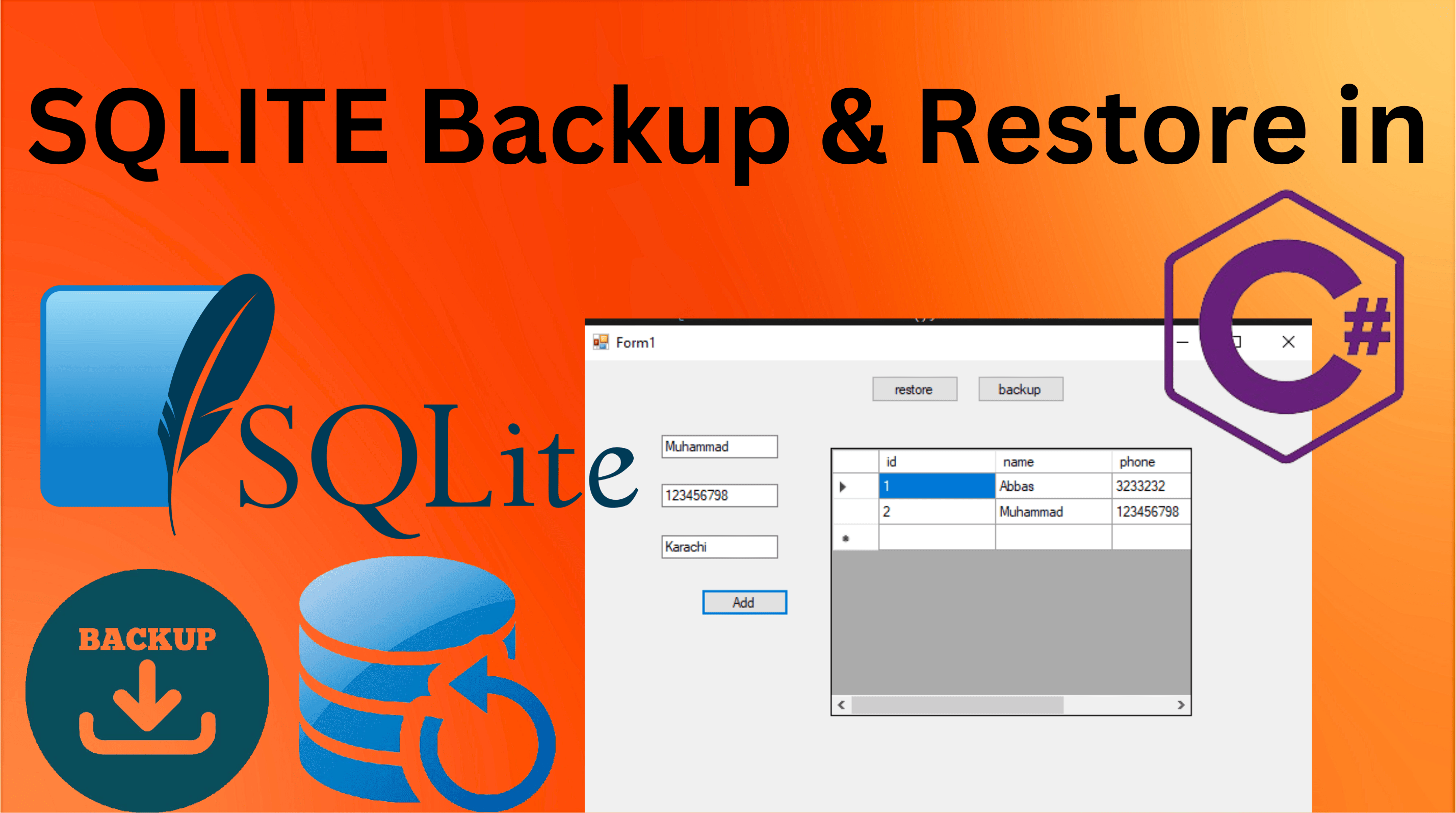
SQLite is a popular choice for lightweight, file-based databases, especially in applications where simplicity and local data storage are priorities. Implementing SQLite backup and restore functionality in C# can help ensure data integrity and provide a mechanism for data recovery in case of data corruption or loss. In this guide, we will cover how to create a backup of an SQLite database and restore it using C#.
To begin with, creating a backup of an SQLite database involves copying the current state of the database file to a backup location. This can be done using the Backup
method provided by SQLite, which allows for copying the entire database or specific tables to a backup file. In C#, you can utilize the System.Data.SQLite
library, which provides an interface for interacting with SQLite databases. The process involves opening a connection to the existing database, creating a new file for the backup, and then using the SQLite Backup
method to copy the data to the new file.
Restoring an SQLite database in C# is essentially the reverse of the backup process. To restore a database, you would first close any existing connections to the current database, then replace the current database file with the backup file. This operation can be done using standard file I/O operations provided by C# to copy the backup file over the existing database file. It’s crucial to ensure that the backup file is not corrupted and is up-to-date to prevent any data loss during the restore process.
how to make backup and restore backup of sqlite in c# !
Create C# Windows Form Project
add some textboxes to store data in your database
add Gridview to show stored data from sqlite
add Restore and Backup Button in Windows Form
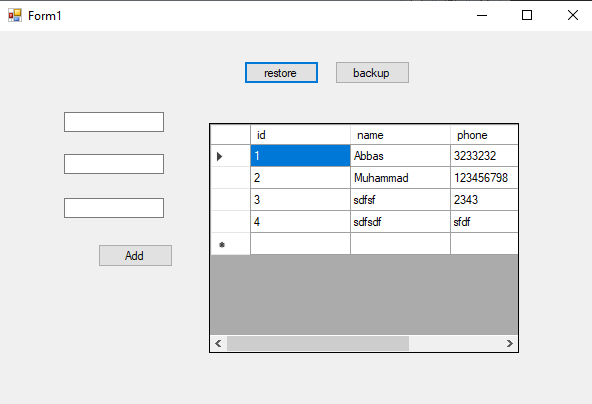
write the following code for database backup
using (SaveFileDialog saveFileDialog = new SaveFileDialog())
{
saveFileDialog.Filter = "SQLite Database File|*.db|All Files|*.*";
saveFileDialog.Title = "Save Backup File";
saveFileDialog.FileName = "backup.db"; // Default filename
if (saveFileDialog.ShowDialog() == DialogResult.OK)
{
string backupFilePath = saveFileDialog.FileName;
try
{
string sourceDatabaseFile = "database.db"; // Change this to your source database file
// Close any existing connections to the target database
SQLiteConnection.ClearAllPools();
// Copy the source database file to create a backup
File.Copy(sourceDatabaseFile, backupFilePath, true);
MessageBox.Show("Backup created successfully.", "Backup Success", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
catch (Exception ex)
{
LogError(ex); // Log the error to the log file
MessageBox.Show("An error occurred: " + ex.Message, "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
}
write the following code for restoring database backup
// Prompt the user to select the backup file
using (OpenFileDialog openFileDialog = new OpenFileDialog())
{
openFileDialog.Filter = "SQLite Database File|*.db|All Files|*.*";
openFileDialog.Title = "Select Backup File";
if (openFileDialog.ShowDialog() == DialogResult.OK)
{
string backupFilePath = openFileDialog.FileName;
try
{
string targetDatabaseFile = "database.db"; // Change this to your target database file
// Close any existing connections to the target database
SQLiteConnection.ClearAllPools();
// Copy the backup file to replace the target database file
File.Copy(backupFilePath, targetDatabaseFile, true);
LoadUserData();
MessageBox.Show("Backup restored successfully.", "Restore Success", MessageBoxButtons.OK, MessageBoxIcon.Information);
}
catch (Exception ex)
{
LogError(ex); // Log the error to the log file
MessageBox.Show("An error occurred: " + ex.Message, "Error", MessageBoxButtons.OK, MessageBoxIcon.Error);
}
}
}
use the following code for error logs
private void LogError(Exception ex)
{
try
{
using (StreamWriter writer = new StreamWriter(logFilePath, true))
{
writer.WriteLine(DateTime.Now.ToString() + ": " + ex.Message);
writer.WriteLine(ex.StackTrace);
writer.WriteLine("-------------------------------------------");
}
}
catch (Exception)
{
// If logging fails, do nothing or display an error message
}
}
if there were any error in your code it will generate run time error logs in error.txt in your bin folder
if facing any issue you can contact me at my facebook page
bookmarked!!, I love your site!