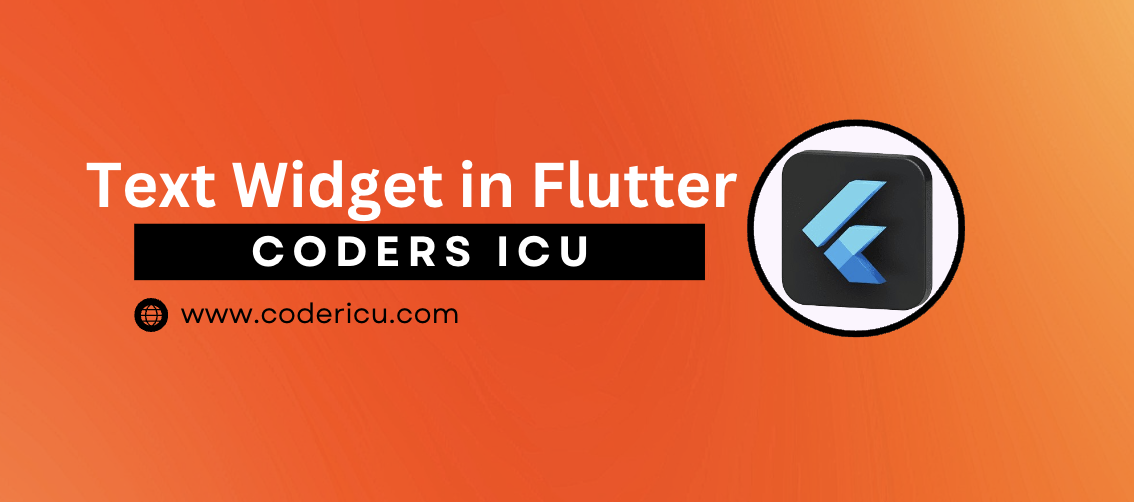
What is a Text Widget in Flutter?
One of the essential tools for displaying a single line of text on the screen in Flutter is the Text widget. The Text widget is a fundamental Flutter widget used to render a single line of text. It is one of the most basic and important widgets in Flutter, providing a straightforward way to display text in your app
The Text widget is an essential component in Flutter, displaying text on the screen. Its numerous attributes allow for customized text appearance and behavior, making it a versatile choice for creating dynamic user interfaces.
Text("Hello World"),
Common Properties of Text Widget
- Text: The text that will be shown. (Type:
String
) - Style: All aspects of the text’s style, such as weight, color, and font size. (Type:
TextStyle
) - TextAlign: This is the widget’s text alignment. determines how the text is aligned horizontally within its container (e.g.,
left
,center
,right
,justify
). (Type:TextAlign
) - Text Direction: text direction describes the directionality of the text (e.g., rtl for right-to-left, ltr for left-to-right).(Type:
TextDirection
) - TextOverflow: What to do if text extends beyond the boundaries of the widget?
Simple Example
Text( 'Coder ICU!', style: TextStyle(fontSize: 24, fontWeight: FontWeight.bold), textAlign: TextAlign.center,,color: Colors.blue
),
Code :
// Import the Flutter material package to use material design widgets
import 'package:flutter/material.dart';
class TextDemo extends StatelessWidget {
@override
Widget build(BuildContext context) {
// The build method returns the widget tree that describes the part of the user interface
return Scaffold(
// Scaffold is a top-level container that holds the basic visual layout structure
appBar: AppBar(
// AppBar is a material design app bar that typically contains a title and actions
title: Text('Flutter Text Example'), // The title of the AppBar
),
body: Center(
// Center widget aligns its child to the center of the available space
child: Text(
'Hello, Coder ICU!', // The text to display on the screen
style: TextStyle(
// TextStyle is used to customize the appearance of the text
fontSize: 24, // Sets the font size of the text
fontWeight: FontWeight.bold, // Sets the font weight to bold
color: Colors.blue, // Sets the color of the text to blue
),
),
),
);
}
}
Next Topics
- RichText
- TextField
- EditableText Widget
- SelectableText
- TextFormField
- CupertinoTextField
1 thought on “Text Widget”