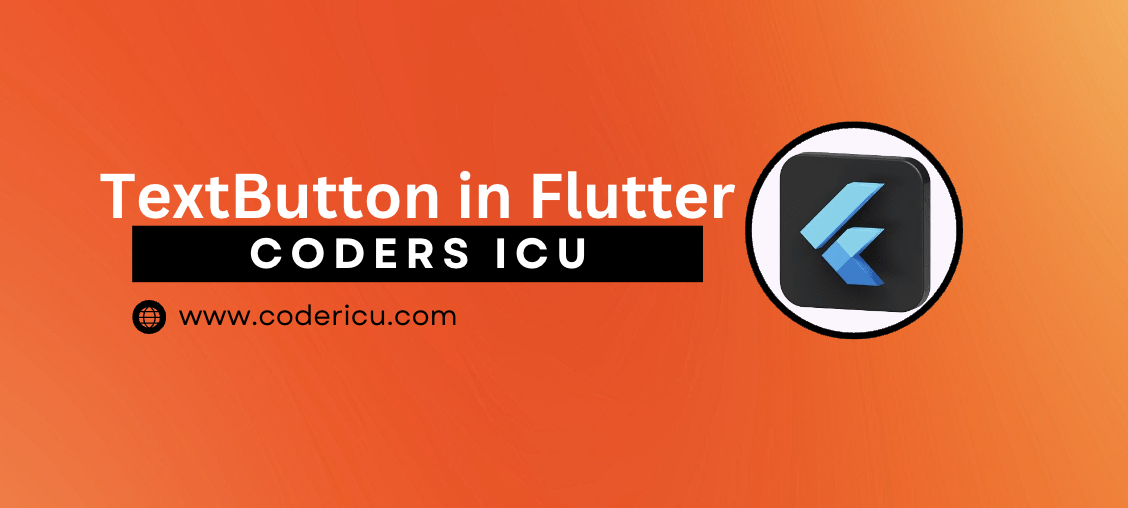
TextButton is a widget in Flutter that displays a text label and can be used to act when tapped. It’s a simple and flexible button widget that can be customized to fit your app’s design.
properties of TextButton:
- child: The text label to display on the button.
- style: The style of the button, including text color, background color, and padding.
- onPressed: The callback function to call when the button is tapped.
- onLongPress: The callback function to call when the button is long-pressed.
- autofocus: Whether the button should autofocus.
TextButton(
child: Text('Tap me!'),
style: TextButton.styleFrom(
primary: Colors.blue, // text color
backgroundColor: Colors.grey[200], // background color
),
onPressed: () {
print('Button tapped!');
},
)
Example
import 'package:flutter/material.dart';
class TextbuttonDemo extends StatefulWidget {
const TextbuttonDemo({Key? key}) : super(key: key);
@override
State<TextbuttonDemo> createState() => _TextbuttonDemoState();
}
class _TextbuttonDemoState extends State<TextbuttonDemo> {
@override
Widget build(BuildContext context) {
return SafeArea(
child: Scaffold(
appBar: AppBar(
title: Text(
"Text Button",
style: TextStyle(color: Colors.white, fontWeight: FontWeight.bold),
),
flexibleSpace: Container(
decoration: BoxDecoration(
gradient: LinearGradient(
colors: [Colors.deepPurple, Colors.orangeAccent],
begin: Alignment.topLeft,
end: Alignment.topRight,
)),
),
),
body: Column(
mainAxisAlignment: MainAxisAlignment.center,
children: [
Center(
child: TextButton(
onPressed: () {
// Handle button press
},
style: TextButton.styleFrom(
foregroundColor: Colors.blue,
backgroundColor: Colors.lightBlueAccent, // Background color
padding: EdgeInsets.symmetric(
horizontal: 20, vertical: 10), // Padding
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.circular(10), // Rounded corners
),
elevation: 5, // Elevation
),
child: Text('Text Button'),
),
),
],
),
),
);
}
}
Output:
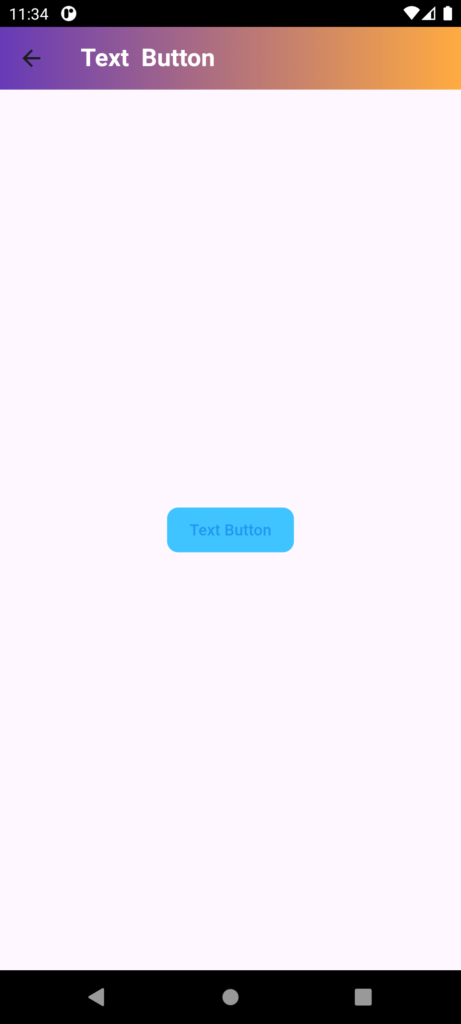