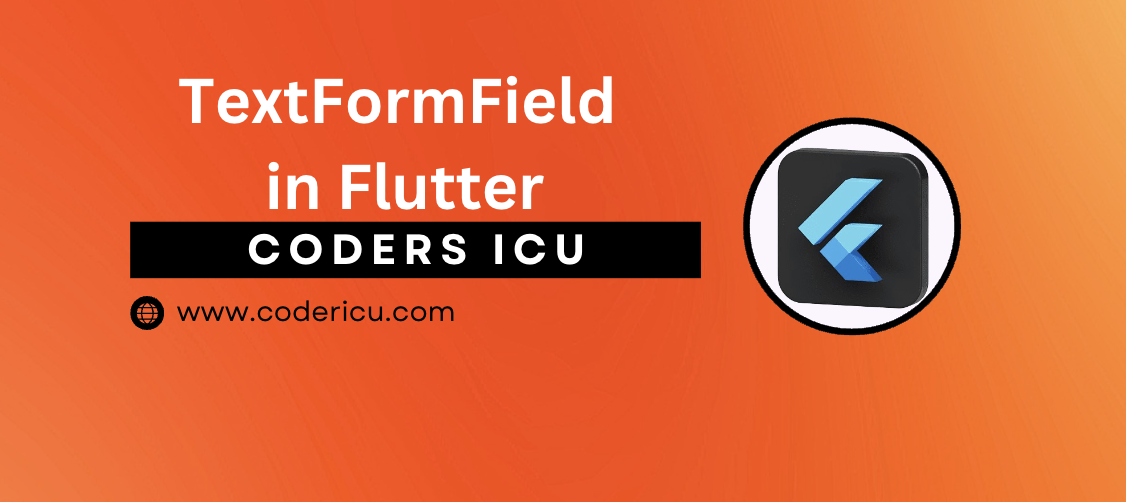
The TextFormField widget in Flutter is a pre-built widget that wraps a TextField widget and integrates it with a Form widget. It’s a convenient way to create a text input field that can be validated and controlled as part of a form. A specialized form field widget for text input with built-in support for form validation, decorating, and interactivity is the TextFormField widget in Flutter.
Some key features of the TextFormField widget:
- Text input: Allows users to input and edit text.
- Validation: Supports validation using a validator callback function.
- Error display: Displays an error message if the input fails validation.
- Form integration: This can be used as part of a Form widget to manage multiple fields.
- Customizable: Can be customized with various properties, such as
keyboardType
, obscureText, and decoration.
Common properties of the TextFormField widget:
- controller: A TextEditingController to manage the text input.
- decoration: An InputDecoration to customize the appearance of the field.
- validator: A callback function to validate the input text.
- keyboardType: The type of keyboard to display (e.g., text, email, password).
- obscureText: Whether to obscure the text input (e.g., for passwords).
- maxLength: The maximum length of the input text.
- onChanged: A callback function when the text input changes.
- onSaved: A callback function when the form is saved.
- autovalidateMode: When to validate the input text (e.g., on user interaction).
- enabled: Whether the field is enabled for user input.
Common uses of TextFormField:
- Login forms
- Registration forms
- Contact forms
- Surveys
- Any scenario where you need to collect user input and validate it.
- And Many More
Simple Example:
TextFormField(
decoration: InputDecoration(
labelText: 'Username'
),
)
)
Example With validator property:
TextFormField(
decoration: InputDecoration(labelText: 'Username'),
onChanged: (value) {
if (value.isEmpty) {
print('Please enter a username');
} else if (value.length < 4) {
print('Username must be at least 4 characters');
}
},
)
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('TextFormField Example'),
),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: MyForm(),
),
),
);
}
}
class MyForm extends StatefulWidget {
@override
_MyFormState createState() => _MyFormState();
}
class _MyFormState extends State<MyForm> {
final _formKey = GlobalKey<FormState>();
final _controller = TextEditingController();
@override
Widget build(BuildContext context) {
return Form(
key: _formKey,
child: Column(
children: <Widget>[
TextFormField(
controller: _controller,
decoration: InputDecoration(
labelText: 'Enter your text',
border: OutlineInputBorder(),
),
validator: (value) {
if (value == null || value.isEmpty) {
return 'Please enter some text';
}
return null;
},
),
SizedBox(height: 20),
ElevatedButton(
onPressed: () {
if (_formKey.currentState!.validate()) {
// Process data
print('Text: ${_controller.text}');
}
},
child: Text('Submit'),
),
],
),
);
}
}
Comparison between TextFormField and TextField:
TextFormField:
- A pre-built widget that wraps a TextField and integrates it with a
Form
widget. - Supports validation and error messages.
- Can be used as part of a form to manage multiple fields.
- Has a validator property to define validation logic.
- Has an autovalidateMode property to control when validation occurs.
TextField:
- A basic text input field widget.
- Does not support validation or error messages by default.
- Can be used standalone or as part of a form.
- Has a
controller
property to manage the text input. - Has a
decoration
property to customize the appearance.